#include<iostream> #include<assert.h> #include<malloc.h> #define CAPACITY 3 using namespace std; class String { public: String(char *str="") :_str((char*)malloc(strlen(str)+1)), _size(strlen(str)) { strcpy(_str, str); _capacity = _size+1; cout << "构造函数" << endl; } String(String const&objstr) :_str(NULL), _size(objstr._size), _capacity(objstr._capacity) { String tmp(objstr._str); swap(_str, tmp._str); } ~String() { cout << "析构函数" << endl; if (_str) { free(_str); } } size_t newcapacity(size_t size) { if (size > _capacity) { _str = (char*)realloc(_str, (size + CAPACITY)); } return size + CAPACITY; } void display() { cout << _str << endl; } void Pushback(char str) { int size = _capacity+1; _capacity = newcapacity(size); _str[_size] = str; _str[_size + 1] = '\0'; _size = _size + 1; } int Find(char x) { if (_str == NULL) { return -1; } int i = 0; for (; i <= (int)_size; i++) { if (_str[i] == x) { return i; } } return -1; } void insert(char x, size_t pos) { int size = _size + 1; _capacity = newcapacity(size); int i = _size; for (; i >(int)pos; i--) { _str[i+1] = _str[i]; } _str[pos+1] = x; _size = _size + 1; } String &operator =(String const &str) { if (str._str == NULL) { _str = NULL; _size = str._size; return *this; } _capacity = newcapacity(str._capacity); char *str1 = str._str; strcpy(_str, str1); _size = str._size; return *this; } int &operator>(String const &str) { int ret = strcmp(_str, str._str); return ret; } private: char * _str; size_t _size; size_t _capacity; }; void Test1() { String str1("abcdef"); String str2(str1); int ret=str2.Find('d'); str2.insert('x', ret); str2.Pushback('a'); cout << ret << endl; str2.display(); } void Test2() { String s1("abcdef"); String s2("efghlmn"); s1 = s2; s1.display(); } int main() { //Test1(); Test2(); return 0; }
简单模拟STL库中string的实现
原创
©著作权归作者所有:来自51CTO博客作者wpfbcr的原创作品,请联系作者获取转载授权,否则将追究法律责任
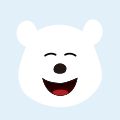
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
STL-常用容器-string
string 类内部封装了很多成员方法例如:查找find,拷贝copy,删除delete 替换replace,插入insertstring管理char*所分配的内存,不用担心复制越界和取值越界等,由类内部进行负责(RAII)
C++STL STL常用容器 string类 string类的常用接口 -
codeup 3.1 简单模拟
问题 A: 剩下的树时间限制:1 Sec内存限制:32 MB题目描述有一个长度为整数L(1<=L<=10000
算法笔记 codeup #include i++ ios -
菜鸟学SSH——简单模拟Hibernate实现原理
之前写了Spring的实现原理,今天我们接着聊聊Hibernate的实现原理,这篇文章只是简单的模
sql hibernate java -
简单模拟javaScript面向对象
动物叫
javascript html 静态变量