#coding=utf-8 import xlrd from xlwt import Workbook, Formula #实现数据存放至excel文件中 def dict2csv(lstDictInfo, strFilePath, lstFieldNames): w = Workbook() #创建一个工作簿 ws = w.add_sheet('sheet1') #创建一个工作表 for i in range(0,len(lstFieldNames)): ws.write(0,i,lstFieldNames[i]) for j in range(0,len(lstDictInfo)): for i in range(0,len(lstFieldNames)): ws.write(j+1,i,lstDictInfo[j][lstFieldNames[i]]) w.save(strFilePath) LstDictInfo1 =[{'id':1,'name':'student1','age':10}, {'id':2,'name':'student2','age':11}, {'id':3,'name':'student3','age':12}] lstFieldNames=['age', 'id', 'name'] dict2csv(LstDictInfo1, 'form.xls', lstFieldNames) #实现读取excel数据 def csv2dict(strFilePath): #将execle文件读出存放至数据字典 data = xlrd.open_workbook(strFilePath) #获取一个工作表 sheet1 = data.sheets()[0] #获取工作表的行数 rows =sheet1.nrows #获取工作表的列数 cols = sheet1.ncols #读取每一行的数据 row_list = [] LstDictInfo=[] for i in range(0,rows): row_data = sheet1.row_values(i) row_list.append(row_data) for i in range(1,len(row_list)-1): LstDictInfo.append(dict(username=row_list[i][0],password=row_list[i][1])) #将数据字典中的值存放至excel #csv2dict('D:\\selenium\\python_oj\\data.xlsx') strFilePath= 'D:\\selenium\\python_oj\\data.xlsx' csv2dict(strFilePath)
Pyhon学习:读写excle文件
原创
©著作权归作者所有:来自51CTO博客作者91ctt的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:Pyhon学习:断言方法
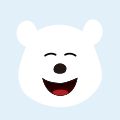
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章