package com.monitoring.common.util;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.util.Map;
import org.apache.log4j.Logger;
import freemarker.template.Configuration;
import freemarker.template.Template;
/**
* @Desc:word操作工具类
*/
public class WordUtil {
private static Logger logger = Logger.getLogger(WordUtil.class);
/**
* @Desc:生成word文件
* @param dataMap word中需要展示的动态数据,用map集合来保存
* @param templateName word模板名称,例如:test.ftl
* @param filePath 文件生成的目标路径,例如:D:/wordFile/
* @param fileName 生成的文件名称,例如:test.doc
*/
@SuppressWarnings("unchecked")
public static void createWord(Map dataMap,String templateName,String filePath,String fileName){
try {
//创建配置实例
Configuration configuration = new Configuration();
//设置编码
configuration.setDefaultEncoding("UTF-8");
//ftl模板文件统一放至 com.lun.template 包下面
configuration.setClassForTemplateLoading(WordUtil.class,"/com/monitoring/common/template/");
//获取模板
Template template = configuration.getTemplate(templateName);
//输出文件
File outFile = new File(filePath+File.separator+fileName);
logger.info(filePath+File.separator+fileName);
//如果输出目标文件夹不存在,则创建
if (!outFile.getParentFile().exists()){
outFile.getParentFile().mkdirs();
}
//将模板和数据模型合并生成文件
Writer out = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(outFile),"UTF-8"));
//生成文件
template.process(dataMap, out);
//关闭流
out.flush();
out.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
@Post("/createWord")
public String createWord(Invocation inv, Analysis model){
Map<String, Object> dataMap = fbStatisticalInfoService.getMap(model);
/** 文件名称,唯一字符串 */
Random r=new Random();
SimpleDateFormat sdf1=new SimpleDateFormat("yyyyMMdd_HHmmss_SSS");
StringBuffer sb=new StringBuffer();
sb.append(sdf1.format(new Date()));
sb.append("_");
sb.append(r.nextInt(100));
//文件路径
String filePath= Constants.DOWNLOAD_BASE_FOLD+"/upload";
//文件唯一名称
String fileOnlyName = "exportWord_"+sb+".doc";
//文件名称
String fileName="news.doc";
/** 生成word */
WordUtil.createWord(dataMap, "report3.ftl", filePath, fileOnlyName);
uploadService.downloadFile(inv, fileName, filePath+"/"+fileOnlyName);
return "@";
}
public String downloadFile(Invocation inv,
String fileName,
String path) {
String userAgent = inv.getRequest().getHeader("User-Agent");
//针对IE或者以IE为内核的浏览器:
if (userAgent.contains("MSIE")||userAgent.contains("Trident")) {
try {
fileName = URLEncoder.encode(fileName, "UTF-8");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
} else {
//非IE浏览器的处理:
try {
fileName = new String(fileName.getBytes("UTF-8"),"ISO-8859-1");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
// path = Constants.DOWNLOAD_BASE_FOLD + "/upload"+"/"+path;
InputStream ins = null;
BufferedInputStream bins = null;
OutputStream outs = null;
BufferedOutputStream bouts = null;
try {
path = new String(path.getBytes("ISO-8859-1"), "UTF-8");
File file = new File(path);// 构造要下载的文件
if (file.exists()) {
// String suffix = path.substring(path.lastIndexOf(".") + 1);
ins = new FileInputStream(path);// 构造一个读取文件的IO流对象
bins = new BufferedInputStream(ins);// 放到缓冲流里面
outs = inv.getResponse().getOutputStream();// 获取文件输出IO流
bouts = new BufferedOutputStream(outs);
inv.getResponse().setContentType("application/octet-stream;charset=utf-8");// 设置response内容的类型
inv.getResponse().setHeader("Content-disposition", String.format("attachment; filename=\"%s\"", fileName));
inv.getResponse().setCharacterEncoding("UTF-8");
int bytesRead = 0;
byte[] buffer = new byte[8192];
// 开始向网络传输文件流
while ((bytesRead = bins.read(buffer, 0, 8192)) != -1) {
bouts.write(buffer, 0, bytesRead);
}
bouts.flush();// 这里一定要调用flush()方法
} else {
logger.info("==============>>下载的文件不存在");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (ins != null) {
ins.close();
ins = null;
}
if (bins != null) {
bins.close();
bins = null;
}
if (outs != null) {
outs.close();
outs = null;
}
if (bouts != null) {
bouts.close();
bouts = null;
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return "@";
}
javabean模板 java word模板
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
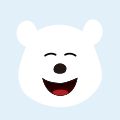
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Idea 模板建议
Idea 模板建议
java 项目结构 解决方案 -
java 模板导出word java导入模板
一:先写 导入1:java是基于包:poi 组件实现的,pom组件如下:<!-- Excel导入功能实现 --> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId>
java 模板导出word java maven struts apache