阅读之后简单写其中的某些功能,先贴图:
第一步:
相关代码:
第二步:1代码中的实现:
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778798081828384858687888990919293949596979899100101102103104105106107108109110111112113114115116117118119120121122123124125126127128129130131132133134135136137138139140141142143144145146147148149150151152153154155156157158159160161162163164165166167168169170171172package
com.jiaruihuademo.testmenu;
import
java.util.ArrayList;
import
java.util.List;
import
android.os.Bundle;
import
android.annotation.SuppressLint;
import
android.app.Activity;
import
android.view.ContextMenu;
import
android.view.ContextMenu.ContextMenuInfo;
import
android.view.Menu;
import
android.view.MenuInflater;
import
android.view.MenuItem;
import
android.view.View;
import
android.view.View.OnCreateContextMenuListener;
import
android.widget.AdapterView;
import
android.widget.AdapterView.AdapterContextMenuInfo;
import
android.widget.AdapterView.OnItemClickListener;
import
android.widget.ArrayAdapter;
import
android.widget.ListView;
import
android.widget.PopupMenu;
import
android.widget.PopupMenu.OnMenuItemClickListener;
import
android.widget.Toast;
/**
*
* menu 的简单使用
*
* @author JiaRH
*
* @date 2013-12-30 上午9:55:29
*/
@SuppressLint
(NewApi)
public
class
MainActivity
extends
Activity
implements
OnMenuItemClickListener,
OnCreateContextMenuListener, OnItemClickListener {
private
ListView listview;
@Override
protected
void
onCreate(Bundle savedInstanceState) {
super
.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listview = (ListView) findViewById(R.id.listView1);
List<string> list =
new
ArrayList<string>();
for
(
int
i =
0
; i <
20
; i++) {
list.add(i + );
}
listview.setAdapter(
new
ArrayAdapter<string>(
this
,
android.R.layout.simple_list_item_1, list));
listview.setOnItemClickListener(
this
);
}
/**
* 创建popviewMenu
*
* @param v
*/
@SuppressLint
(NewApi)
public
void
showpop(View v) {
PopupMenu popup =
new
PopupMenu(
this
, v);
MenuInflater inflater = popup.getMenuInflater();
inflater.inflate(R.menu.main, popup.getMenu());
popup.setOnMenuItemClickListener(
this
);
popup.show();
}
@Override
public
boolean
onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return
true
;
}
/*
* (non-Javadoc)
*
* @see android.app.Activity#onOptionsItemSelected(android.view.MenuItem)
*/
@Override
public
boolean
onOptionsItemSelected(MenuItem item) {
switch
(item.getItemId()) {
case
R.id.item1:
Toast.makeText(
this
, you have clicked
1
,
0
).show();
break
;
case
R.id.item2:
Toast.makeText(
this
, you have clicked
2
,
0
).show();
break
;
case
R.id.item3:
Toast.makeText(
this
, you have clicked
3
,
0
).show();
break
;
case
R.id.item4:
Toast.makeText(
this
, you have clicked
4
,
0
).show();
break
;
default
:
break
;
}
return
super
.onOptionsItemSelected(item);
}
/**
* 响应popmenu的点击事件
*/
@Override
public
boolean
onMenuItemClick(MenuItem item) {
switch
(item.getItemId()) {
case
R.id.item1:
Toast.makeText(
this
, you have clicked
1
,
0
).show();
break
;
case
R.id.item2:
Toast.makeText(
this
, you have clicked
2
,
0
).show();
break
;
case
R.id.item3:
Toast.makeText(
this
, you have clicked
3
,
0
).show();
break
;
case
R.id.item4:
Toast.makeText(
this
, you have clicked
4
,
0
).show();
break
;
default
:
break
;
}
return
false
;
}
/**
* listview响应事件
*/
@Override
public
void
onItemClick(AdapterView<!--?--> parent, View view,
int
position,
long
id) {
// TODO Auto-generated method stub
registerForContextMenu(view);
}
@Override
public
void
onCreateContextMenu(ContextMenu menu, View v,
ContextMenuInfo menuInfo) {
super
.onCreateContextMenu(menu, v, menuInfo);
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.main, menu);
}
@Override
public
boolean
onContextItemSelected(MenuItem item) {
AdapterContextMenuInfo info = (AdapterContextMenuInfo) item
.getMenuInfo();
switch
(item.getItemId()) {
case
R.id.item1:
Toast.makeText(
this
, you have clicked
1
,
0
).show();
return
true
;
case
R.id.item2:
Toast.makeText(
this
, you have clicked
2
,
0
).show();
return
true
;
case
R.id.item3:
Toast.makeText(
this
, you have clicked
3
,
0
).show();
return
true
;
case
R.id.item4:
Toast.makeText(
this
, you have clicked
4
,
0
).show();
return
true
;
default
:
return
super
.onContextItemSelected(item);
}
}
}
</string></string></string>
第三步;附上布局文件:123456<relativelayout android:layout_height=
"match_parent"
android:layout_width=
"match_parent"
android:paddingbottom=
"@dimen/activity_vertical_margin"
android:paddingleft=
"@dimen/activity_horizontal_margin"
android:paddingright=
"@dimen/activity_horizontal_margin"
android:paddingtop=
"@dimen/activity_vertical_margin"
tools:context=
".MainActivity"
xmlns:android=
"http://schemas.android.com/apk/res/android"
xmlns:tools=
"http://schemas.android.com/tools"
>
<textview android:id=
"@+id/textView1"
android:layout_height=
"wrap_content"
android:layout_width=
"wrap_content"
android:text=
"@string/hello_world"
><button android:id=
"@+id/showpop"
android:layout_below=
"@+id/textView1"
android:layout_height=
"wrap_content"
android:layout_margintop=
"16dp"
android:layout_width=
"wrap_content"
android:onclick=
"showpop"
android:text=
"show"
popmenu=
""
>
<listview android:id=
"@+id/listView1"
android:layout_alignleft=
"@+id/showpop"
android:layout_below=
"@+id/showpop"
android:layout_height=
"wrap_content"
android:layout_margintop=
"40dp"
android:layout_width=
"match_parent"
>
</listview></button></textview></relativelayout>
备注;我使用的
android:minSdkVersion=8
android:targetSdkVersion=18 />
android Menu 菜单使用总结
精选 转载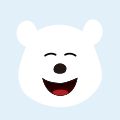
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
有趣的CSS - 汉堡菜单按钮
用 css 实现汉堡菜单图标与关闭图标过渡动画交互效果。
css 汉堡图标 动画 ux 交互体验 -
Spring Boot结合RabbitMQ使用总结
Spring Boot结合RabbitMQ使用总结
消息队列 spring java -
Android Menu菜单
dle savedInstanceState) { super.onCreate(save
选项菜单 菜单项 上下文菜单 -
Android随笔---menu菜单的使用
Android随笔---menu菜单的使用
android java apache xml ide -
android menu弹出菜单 android menu菜单
1.1 Menu 菜单【Menu 菜单1. OptionsMenu 选项菜单特点: 当通过点击menu键或者3.0以上的手机上,点击右上方的三个点,出现的列表,都是有OptionMenu对象进行控制2.  
android menu弹出菜单 menu 菜单的使用 android 实例分享 -
android menu菜单横向 android中menu
今天在微信公众号看了一篇推送文章,是一个炫酷的menu(菜单)实现方法,自己想了想,好像之前没怎么用过menu,于是今天花点时间,把menu基本用法和各种效果看看书,实现一下,算是基础学习的开始吧。查了下资料,Android常用的菜单类型主要有: 1).OptionMenu - 选项菜单 2).SubMenu &nbs
android menu菜单横向 android menu android studio 界面