import com.google.zxing.BarcodeFormat; import com.google.zxing.EncodeHintType; import com.google.zxing.MultiFormatWriter; import com.google.zxing.WriterException; import com.google.zxing.client.j2se.MatrixToImageWriter; import com.google.zxing.common.BitMatrix; import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import sun.font.FontDesignMetrics;
import javax.imageio.ImageIO; import javax.swing.; import java.awt.; import java.awt.image.BufferedImage; import java.io.*; import java.util.HashMap; import java.util.Map;
public class QrCodeUtil { private static final Logger logger = LoggerFactory.getLogger(QrCodeUtil.class);
// logo默认边框颜色 public static final Color DEFAULT_BORDERCOLOR = Color.WHITE; // logo默认边框宽度 public static final int DEFAULT_BORDER = 1; // logo大小默认为照片的1/6 public static final int DEFAULT_LOGOPART = 6; private final int border = DEFAULT_BORDER; private final Color borderColor; private final int logoPart; /** * Creates a default config with on color and off color * generating normal black-on-white barcodes. * 在颜色{@ link #黑}和颜色上创建一个默认配置 * {@link # WHITE},生成正常的黑白条码。 */ public QrCodeUtil() { this(DEFAULT_BORDERCOLOR, DEFAULT_LOGOPART); } public QrCodeUtil(Color borderColor, int logoPart) { this.borderColor = borderColor; this.logoPart = logoPart; } public Color getBorderColor() { return borderColor; } public int getBorder() { return border; } public int getLogoPart() { return logoPart; } /** * 给二维码图片添加Logo * * @param qrPic * @param logoPic */ public static void addLogo_QRCode(File qrPic, File logoPic, QrCodeUtil creatrQrCode) { try { if (!qrPic.isFile() || !logoPic.isFile()) { System.out.print("file not find !"); System.exit(0); } /** * 读取二维码图片,并构建绘图对象 */ BufferedImage image = ImageIO.read(qrPic); Graphics2D g = image.createGraphics(); /** * 读取Logo图片 */ BufferedImage logo = ImageIO.read(logoPic); int widthLogo = image.getWidth() / creatrQrCode.getLogoPart(); // int heightLogo = image.getHeight()/logoConfig.getLogoPart(); int heightLogo = image.getWidth() / creatrQrCode.getLogoPart(); //保持二维码是正方形的 // 计算图片放置位置 int x = (image.getWidth() - widthLogo) / 2; int y = (image.getHeight() - heightLogo) / 2; //开始绘制图片 g.drawImage(logo, x, y, widthLogo, heightLogo, null); g.drawRoundRect(x, y, widthLogo, heightLogo, 10, 10); g.setStroke(new BasicStroke(creatrQrCode.getBorder())); g.setColor(creatrQrCode.getBorderColor()); g.drawRect(x, y, widthLogo, heightLogo); g.dispose(); ImageIO.write(image, "jpeg", new File("D:/ceshi/789.png")); } catch (Exception e) { e.printStackTrace(); } } /** * @param pressText 文字 * @param targetImg 需要添加文字的图片 * @param fontStyle * @param color * @param fontSize * @param width * @param height * @为图片添加文字 */ public static void pressText(String pressText, String targetImg, int fontStyle, Color color, int fontSize, int width, int height) { //计算文字开始的位置 //x开始的位置:(图片宽度-字体大小*字的个数)/2 int startX = (width - (fontSize * pressText.length())) / 100; //y开始的位置:图片高度-(图片高度-图片宽度)/2 int startY = height - (height - width) / 3; Font font = new Font("微软雅黑", fontStyle,fontSize); try { File file = new File(targetImg); Image src = ImageIO.read(file); int imageW = src.getWidth(null); int imageH = src.getHeight(null); BufferedImage image = new BufferedImage(imageW, imageH, BufferedImage.TYPE_INT_RGB); Graphics g = image.createGraphics(); g.drawImage(src, 0, 0, imageW, imageH, null); g.setColor(color); g.setFont(new Font(null, fontStyle, fontSize)); String text="扫一扫获取预览效果图"; int wordWidth = getWordWidth(font, text); g.drawString(text,(width-wordWidth)/2,40); pressText="人工客服升级了,便捷服务只为您!"; drawString(g,font,pressText,(width-wordWidth)/2, startY,180); g.dispose(); System.out.println("image press success"); } catch (Exception e) { System.out.println(e); } } /** * @param firstTitle 顶部标题,固定文字 * @param secoundTitle 底部标题,消息标题 * @param out 需要添加文字的图片 * @param fontStyle * @param color * @param fontSize * @param width * @param height * @为图片添加文字 */ public static InputStream pressText(String firstTitle, String secoundTitle, ByteArrayOutputStream out,int fontStyle, Color color, int fontSize, int width, int height) throws IOException { //计算文字开始的位置 //x开始的位置:(图片宽度-字体大小*字的个数)/2
// int startX = (width - (fontSize * secoundTitle.length())) / 100; //y开始的位置:图片高度-(图片高度-图片宽度)/2 int startY = height - (height - width) / 3; InputStream input=null; Font font = new Font("微软雅黑", fontStyle,fontSize); ByteArrayInputStream resultInputStream = null; try { input=ConvertUtil.parse(out); input.mark(0); Image src = ImageIO.read(input); int imageW = src.getWidth(null); int imageH = src.getHeight(null); BufferedImage image = new BufferedImage(imageW, imageH, BufferedImage.TYPE_INT_RGB); Graphics g = image.createGraphics(); g.setColor(color); g.setFont(new Font(null, fontStyle, fontSize)); g.drawImage(src, 0, 0, imageW, imageH, null); int wordWidth = getWordWidth(font, firstTitle); g.drawString(firstTitle,(width-wordWidth)/2,40); drawString(g,font,secoundTitle,(width-wordWidth)/2, startY,180); g.dispose(); out=new ByteArrayOutputStream(); ImageIO.write(image, "jpg", out); resultInputStream =ConvertUtil.parse(out); logger.info("image press success"); } catch (Exception e) { logger.info("系统异常,异常信息为:"+e.getMessage()); }finally { if (input!=null){ input.close(); } if(out != null){ out.close(); } } return resultInputStream; }
public static void drawString(Graphics g , Font font , String text , int x , int y , int maxWidth) { JLabel label = new JLabel(text); label.setFont(font); FontMetrics metrics = label.getFontMetrics(label.getFont()); int textH = metrics.getHeight(); int textW = metrics.stringWidth(label.getText()); //字符串的宽 String tempText = text; while(textW > maxWidth) { int n = textW / maxWidth; int subPos = tempText.length() / n; String drawText = tempText.substring(0 , subPos); int subTxtW = metrics.stringWidth(drawText); while(subTxtW > maxWidth) { subPos--; drawText = tempText.substring(0 , subPos); subTxtW = metrics.stringWidth(drawText); } g.drawString(drawText , x , y); y += textH; textW = textW - subTxtW; tempText = tempText.substring(subPos); } g.drawString(tempText , x , y); } //得到该字体字符串的长度 public static int getWordWidth(Font font, String content) { FontDesignMetrics metrics = FontDesignMetrics.getMetrics(font); int width = 0; for (int i = 0; i < content.length(); i++) { width += metrics.charWidth(content.charAt(i)); } return width; } public static InputStream createQrcode(String url) throws Exception { ByteArrayOutputStream output = new ByteArrayOutputStream(); MultiFormatWriter multiFormatWriter = new MultiFormatWriter(); InputStream input=null; @SuppressWarnings("rawtypes") Map hints = new HashMap(); //设置UTF-8, 防止中文乱码 hints.put(EncodeHintType.CHARACTER_SET, "UTF-8"); //设置二维码四周白色区域的大小 hints.put(EncodeHintType.MARGIN,0); //设置二维码的容错性 hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H); //width:图片完整的宽;height:图片完整的高 //因为要在二维码下方附上文字,所以把图片设置为长方形(高大于宽) int width = 200; int height = 300; int font = 15; //字体大小 int fontStyle = 4; //字体风格4 //画二维码,记得调用multiFormatWriter.encode()时最后要带上hints参数,不然上面设置无效 BitMatrix bitMatrix = null; try { bitMatrix = multiFormatWriter.encode(url, BarcodeFormat.QR_CODE, width, height, hints); //开始画二维码 MatrixToImageWriter.writeToStream(bitMatrix, "jpg", output); input=ConvertUtil.parse(output); // input= pressText(firstTitle,secoundTitle,output,fontStyle,Color.black,font,width,height); } catch (WriterException e) { e.printStackTrace(); logger.info("系统异常,异常信息为:"+e.getMessage()); } catch (IOException e) { e.printStackTrace(); logger.info("系统异常,异常信息为:"+e.getMessage()); } return input; }
}
二维码工具类
原创
©著作权归作者所有:来自51CTO博客作者IT达仁的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:Base64Image工具类
下一篇:阿里云oss 上传进度条
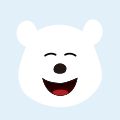
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
草料二维码-免费的二维码生成工具
二维码又称QR Code,是一个近几年来移动设备上超流行的一种编码方式,它比传统的Bar Code条成二维码的工具类小程序。『草料二维码』小程序。...
python 小程序 人工智能 大数据 机器学习 -
php二维码识别类
php二维码识别类
php 二维码识别 -
ZXing 二维码解析生成工具类
原文:http://www.open-open.com/code/view/1455848023292
java 二维码 生成二维码 二维码生成 支付宝