import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.ColorMatrix;
import android.graphics.ColorMatrixColorFilter;
import android.graphics.Paint;
import android.graphics.PorterDuffXfermode;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.Bitmap.Config;
import android.graphics.PorterDuff.Mode;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.Drawable;
public class imageTool {
public static final int LEFT = 0;
public static final int RIGHT = 1;
public static final int TOP = 3;
public static final int BOTTOM = 4;
public static Bitmap toGrayscale(Bitmap bmpOriginal) {
int width, height;
height = bmpOriginal.getHeight();
width = bmpOriginal.getWidth();
Bitmap bmpGrayscale = Bitmap.createBitmap(width, height,
Bitmap.Config.RGB_565);
Canvas c = new Canvas(bmpGrayscale);
Paint paint = new Paint();
ColorMatrix cm = new ColorMatrix();
cm.setSaturation( 0);
ColorMatrixColorFilter f = new ColorMatrixColorFilter(cm);
paint.setColorFilter(f);
c.drawBitmap(bmpOriginal, 0, 0, paint);
return bmpGrayscale;
}
public static Bitmap toGrayscale(Bitmap bmpOriginal, int pixels) {
return toRoundCorner(toGrayscale(bmpOriginal), pixels);
}
public static Bitmap toRoundCorner(Bitmap bitmap, int pixels) {
Bitmap output = Bitmap.createBitmap(bitmap.getWidth(), bitmap
.getHeight(), Config.ARGB_8888);
Canvas canvas = new Canvas(output);
final int color = 0xff424242;
final Paint paint = new Paint();
final Rect rect = new Rect( 0, 0, bitmap.getWidth(), bitmap.getHeight());
final RectF rectF = new RectF(rect);
final float roundPx = pixels;
paint.setAntiAlias( true);
canvas.drawARGB( 0, 0, 0, 0);
paint.setColor(color);
canvas.drawRoundRect(rectF, roundPx, roundPx, paint);
paint.setXfermode( new PorterDuffXfermode(Mode.SRC_IN));
canvas.drawBitmap(bitmap, rect, rect, paint);
return output;
}
public static BitmapDrawable toRoundCorner(BitmapDrawable bitmapDrawable,
int pixels) {
Bitmap bitmap = bitmapDrawable.getBitmap();
bitmapDrawable = new BitmapDrawable(toRoundCorner(bitmap, pixels));
return bitmapDrawable;
}
public static void saveBefore(String path) {
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
// 获取这个图片的宽和高
Bitmap bitmap = BitmapFactory.decodeFile(path, options); // 此时返回bm为空
options.inJustDecodeBounds = false;
// 计算缩放比
int be = ( int) (options.outHeight / ( float) 200);
if (be <= 0)
be = 1;
options.inSampleSize = 2; // 图片长宽各缩小二分之一
// 重新读入图片,注意这次要把options.inJustDecodeBounds 设为 false哦
bitmap = BitmapFactory.decodeFile(path, options);
int w = bitmap.getWidth();
int h = bitmap.getHeight();
System. out.println(w + " " + h);
// savePNG_After(bitmap,path);
saveJPGE_After(bitmap, path);
}
public static void savePNG_After(Bitmap bitmap, String name) {
File file = new File(name);
try {
FileOutputStream out = new FileOutputStream(file);
if (bitmap.compress(Bitmap.CompressFormat.PNG, 100, out)) {
out.flush();
out.close();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void saveJPGE_After(Bitmap bitmap, String path) {
File file = new File(path);
try {
FileOutputStream out = new FileOutputStream(file);
if (bitmap.compress(Bitmap.CompressFormat.JPEG, 100, out)) {
out.flush();
out.close();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public static Bitmap createBitmapForWatermark (Bitmap src, Bitmap watermark) {
if (src == null) {
return null;
}
int w = src.getWidth();
int h = src.getHeight();
int ww = watermark.getWidth();
int wh = watermark.getHeight();
// create the new blank bitmap
Bitmap newb = Bitmap.createBitmap(w, h, Config.ARGB_8888); // 创建一个新的和SRC长度宽度一样的位图
Canvas cv = new Canvas(newb);
// draw src into
cv.drawBitmap(src, 0, 0, null); // 在 0,0坐标开始画入src
// draw watermark into
cv.drawBitmap(watermark, w - ww + 5, h - wh + 5, null); // 在src的右下角画入水印
// save all clip
cv.save(Canvas.ALL_SAVE_FLAG); // 保存
// store
cv.restore(); // 存储
return newb;
}
public static Bitmap potoMix( int direction, Bitmap... bitmaps) {
if (bitmaps.length <= 0) {
return null;
}
if (bitmaps.length == 1) {
return bitmaps[ 0];
}
Bitmap newBitmap = bitmaps[ 0];
// newBitmap = createBitmapForFotoMix(bitmaps[0],bitmaps[1],direction);
for ( int i = 1; i < bitmaps.length; i++) {
newBitmap = createBitmapForFotoMix(newBitmap, bitmaps[i], direction);
}
return newBitmap;
}
private static Bitmap createBitmapForFotoMix(Bitmap first, Bitmap second,
int direction) {
if (first == null) {
return null;
}
if (second == null) {
return first;
}
int fw = first.getWidth();
int fh = first.getHeight();
int sw = second.getWidth();
int sh = second.getHeight();
Bitmap newBitmap = null;
if (direction == LEFT) {
newBitmap = Bitmap.createBitmap(fw + sw, fh > sh ? fh : sh,
Config.ARGB_8888);
Canvas canvas = new Canvas(newBitmap);
canvas.drawBitmap(first, sw, 0, null);
canvas.drawBitmap(second, 0, 0, null);
} else if (direction == RIGHT) {
newBitmap = Bitmap.createBitmap(fw + sw, fh > sh ? fh : sh,
Config.ARGB_8888);
Canvas canvas = new Canvas(newBitmap);
canvas.drawBitmap(first, 0, 0, null);
canvas.drawBitmap(second, fw, 0, null);
} else if (direction == TOP) {
newBitmap = Bitmap.createBitmap(sw > fw ? sw : fw, fh + sh,
Config.ARGB_8888);
Canvas canvas = new Canvas(newBitmap);
canvas.drawBitmap(first, 0, sh, null);
canvas.drawBitmap(second, 0, 0, null);
} else if (direction == BOTTOM) {
newBitmap = Bitmap.createBitmap(sw > fw ? sw : fw, fh + sh,
Config.ARGB_8888);
Canvas canvas = new Canvas(newBitmap);
canvas.drawBitmap(first, 0, 0, null);
canvas.drawBitmap(second, 0, fh, null);
}
return newBitmap;
}
public static Bitmap createBitmapBySize(Bitmap bitmap, int width, int height) {
return Bitmap.createScaledBitmap(bitmap, width, height, true);
}
public static Bitmap drawableToBitmapByBD(Drawable drawable) {
BitmapDrawable bitmapDrawable = (BitmapDrawable) drawable;
return bitmapDrawable.getBitmap();
}
public static Drawable bitmapToDrawableByBD(Bitmap bitmap) {
Drawable drawable = new BitmapDrawable(bitmap);
return drawable;
}
public static Bitmap bytesToBimap( byte[] b) {
if (b.length != 0) {
return BitmapFactory.decodeByteArray(b, 0, b.length);
} else {
return null;
}
}
public static byte[] bitmapToBytes(Bitmap bm) {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
bm.compress(Bitmap.CompressFormat.PNG, 100, baos);
return baos.toByteArray();
}
}
android 处理图片工具
原创
©著作权归作者所有:来自51CTO博客作者Bensantan的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:Android 轻松实现语音识别
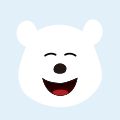
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
PictureCleaner:一款实用的本地图片处理工具
PictureCleaner:一款实用的本地图片处理工具
图片处理 实用软件 背景色 PictureCleaner -
图片处理 - PHP 工具类
图片处理 - PHP 工具类
php 图片处理 工具类 -
nodejs处理图片工具sharp
有个需求是把图片裁剪成目标大小,这块因为是前端,采用的技术是js,
javascript 前端 开发语言 运行环境 centos -
android 图片处理滤镜功能 安卓图片处理工具
在上期内容中,我分享了几款私藏的VSCO调色参数和照片朦胧效果制作方法,许多朋友都对文末的照片朦胧效果制作方法的内容十分感兴趣,而同时提到的一款图片编辑软件 —— Pixlr, 许多朋友都感到有点陌生。其实 Pixlr 也是也是一款优秀的老软件了,起步于安卓系统,目前双平台兼具。在使用Pixlr拍照时,有8种实时滤镜效果可以选择。另外它的内置滤镜多达600多种,很多是其它软件没有的效果;相框和贴纸
android 图片处理滤镜功能 render cols 图片 uniapp 用nvue分享图片不显示 手机照片局部放大镜 Express