package com.sample.pass.gmine.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class ParameterUtil {
/**
* 判断集合是否为空
*
* @param collection 集合
* @return true 为空 false 不为空
*/
public static boolean isEmpty(Collection<?> collection) {
return collection == null || collection.isEmpty();
}
/**
* 判断Map是否为空
*
* @param map map
* @return true 为空 false 不为空
*/
public static boolean isEmpty(Map<?, ?> map) {
return map == null || map.isEmpty();
}
/**
* 判断数组是否为空
*
* @param array 数组
* @return true 为空 false 不为空
*/
public static boolean isEmpty(Object[] array) {
return array == null || array.length == 0;
}
/**
* 判断List是否为空
*
* @param list List
* @return true 为空 false 不为空
*/
public static boolean isEmpty(List<Object> list) {
return list == null || list.size() == 0;
}
/**
* 判断字符串是否为空
*
* @param str 字符串
* @return true 为空 false 不为空
*/
public static boolean isEmpty(String str) {
if ("".equals(str) || str == null) {
return true;
} else {
return false;
}
}
//不为空
/**
* 判断字符串是否不为空
*
* @param str 字符串
* @return true 不为空 false 为空
*/
public static boolean isNotEmpty(String str) {
return !isEmpty(str);
}
/**
* 判断字符串是否殊字符
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isSpecial(String str) {
if (str != null && !"".equals(str)) {//""
String regEx = "[&\\\\/*<>@!]";
Pattern p = Pattern.compile(regEx);
Matcher m = p.matcher(str);
//如果含有就返回true
return m.find();
} else {
return false;
}
}
/**
* 字符串数字和- 判断
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isNumberAnd(String str) {
if (str != null && !"".equals(str)) {
Pattern pattern = Pattern.compile("[0-9,-]+");
Matcher isNum = pattern.matcher(str);
return !isNum.matches();
} else {
return false;
}
}
/**
* 字符串仅支持纯数字
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isOnlyNumber(String str) {
if (str != null && !"".equals(str)) {
Pattern pattern = Pattern.compile("[0-9,.]+");
Matcher isNum = pattern.matcher(str);
return !isNum.matches();
} else {
return false;
}
}
/**
* 字符串是否超过最大长度
*
* @param str 字符串
* @param max 最大长度
* @return true 是 false 否
*/
public static boolean maxLength(String str, Integer max) {
if (str != null && !"".equals(str)) {
return (str.length() > max);
} else {
return false;
}
}
/**
* 字符串是否低于最小长度
*
* @param str 字符串
* @param min 最小长度
* @return true 是 false 否
*/
public static boolean minLength(String str, Integer min) {
if (str != null && !"".equals(str)) {
return (str.length() < min);
} else {
return false;
}
}
/**
* 字符串判断为数字或字母
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isNumOrEng(String str) {
if (str != null && !"".equals(str)) {
String regex = "[a-z0-9A-Z]+";
return !str.matches(regex);
} else {
return false;
}
}
/**
* 字符串判断为字母
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isEng(String str) {
if (str != null && !"".equals(str)) {
String regex = "[a-zA-Z]+";
return !str.matches(regex);
} else {
return false;
}
}
/**
* 字符串判断是否为邮箱
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isEmail(String str) {
if (str != null && !"".equals(str)) {
String regex = "\\w+@\\w+(\\.[a-zA-Z]+)+";
return !str.matches(regex);
} else {
return false;
}
}
/**
* 判断是否有空格
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isBlank(String str) {
if (str != null && !"".equals(str)) {
return (str.lastIndexOf(" ") != -1);
} else {
return false;
}
}
/**
* 判断是否有汉字
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isCharacter(String str) {
if (str != null && !"".equals(str)) {
String regex = "[\\u4e00-\\u9fa5]+";
return str.matches(regex);
} else {
return false;
}
}
/**
* 判断日期格式
*
* @param str 字符串
* @return true 是 false 否
*/
public static boolean isValidDate(String str) {
boolean convertSuccess = true;
SimpleDateFormat format = new SimpleDateFormat("yyyy/MM/dd");
try {
format.setLenient(false);
format.parse(str);
} catch (ParseException e) {
convertSuccess = false;
}
return convertSuccess;
}
}
java $ 参数 java参数校验工具类推荐
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
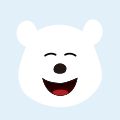
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
实现java参数非必传
1、路径参数:@PathVariable(required = false)实现参数aaa非必传2、非路径参数:@RequestParam(required = false) 实现参数aaa非必传3、实体参数:@RequestBody(required = false)实现实体aaa非必传
参数 required @PathVariable @RequestParam @RequestBody