func matchCards(cards []int, wildCount int) int {
// 统计每个点数的数量
countMap := make(map[int]int)
for _, card := range cards {
countMap[card]++
}
points := []int{31, 32, 33, 34}
// 计算所有牌的总数(包括万能牌)
totalCards := wildCount
for _, count := range countMap {
totalCards += count
}
// 如果总牌数不是3的倍数,无法完全匹配
if totalCards%3 != 0 {
return 0
}
targetMatches := totalCards / 3
maxMatches := 0
// 尝试所有可能的组合方式
var tryMatch func(map[int]int, int, int) int
tryMatch = func(currCount map[int]int, remainWild int, depth int) int {
if depth == targetMatches {
return depth
}
matches := 0
// 遍历所有可能的三张不同点数组合
for i := 0; i < len(points); i++ {
for j := i + 1; j < len(points); j++ {
for k := j + 1; k < len(points); k++ {
// 复制当前状态
tempCount := make(map[int]int)
for k, v := range currCount {
tempCount[k] = v
}
// 计算需要的万能牌数量
needWild := 0
if tempCount[points[i]] == 0 {
needWild++
}
if tempCount[points[j]] == 0 {
needWild++
}
if tempCount[points[k]] == 0 {
needWild++
}
// 如果需要的万能牌数量在允许范围内
if needWild <= remainWild {
// 使用牌
if tempCount[points[i]] > 0 {
tempCount[points[i]]--
}
if tempCount[points[j]] > 0 {
tempCount[points[j]]--
}
if tempCount[points[k]] > 0 {
tempCount[points[k]]--
}
// 递归尝试下一组
currMatches := tryMatch(tempCount, remainWild-needWild, depth+1)
if currMatches > matches {
matches = currMatches
}
}
}
}
}
return matches
}
// 开始尝试匹配
maxMatches = tryMatch(countMap, wildCount, 0)
// 只有当能完全匹配所有牌时才返回结果
if maxMatches*3 == totalCards {
return maxMatches
}
return 0
}
func main() {
//cards := []int32{24, 24, 25, 25, 14, 16, 17, 18, 19, 6, 6}
//patternCards := []int32{6, 8}
//isWin := CheckWin(cards, patternCards)
//fmt.Printf("isWin:%v\n", isWin)
testCases := []struct {
cards []int
wild int
expected int
}{
{[]int{31, 31, 32, 32}, 2, 2}, // 2组:(31,32,wild),(31,32,wild)
{[]int{31, 32, 33}, 0, 1}, // 1组:(31,32,33)
{[]int{31, 31, 32}, 1, 0}, // 0组:无法完全匹配所有牌
{[]int{31, 32}, 1, 1}, // 1组:(31,32,wild)
{[]int{31, 31, 31, 32, 32, 32}, 3, 3}, // 3组:每组用1个万能牌当33
{[]int{31, 31, 32, 32, 33, 33, 34}, 2, 3}, // 3组
{[]int{31, 31, 32, 32}, 2, 2}, // 使用2张万能牌组成2组
{[]int{31, 32, 33}, 0, 1}, // 不使用万能牌
{[]int{31, 31, 32}, 1, 0}, // 无法完全匹配
{[]int{31, 32}, 1, 1}, // 使用1张万能牌
{[]int{31, 31, 31, 32, 32, 32}, 3, 3}, // 每组使用1张万能牌
{[]int{31, 31, 32, 32, 33, 33, 34}, 2, 3}, // 最后一组使用2张万能牌
// 补充测试用例
{[]int{}, 3, 1}, // 只有万能牌
{[]int{31}, 2, 1}, // 1张实际牌+2张万能牌
{[]int{31, 32}, 4, 2}, // 2张实际牌+4张万能牌
{[]int{31, 31, 31}, 6, 3}, // 3张相同点数+6张万能牌
{[]int{31, 32, 33, 34}, 2, 2}, // 4种点数+2张万能牌
{[]int{31, 31, 32, 32, 33, 33}, 0, 2}, // 6张牌刚好组成2组
{[]int{31, 31, 31, 32, 32, 32, 33, 33, 33}, 0, 3}, // 9张牌刚好组成3组
{[]int{31, 32, 33, 34}, 5, 3}, // 可以组成3组
{[]int{31, 31, 32, 32}, 1, 0}, // 无法完全匹配(5张牌)
{[]int{31, 31, 31, 32, 32}, 0, 0}, // 无法完全匹配(5张牌)
{[]int{31, 32, 33, 34}, 1, 0}, // 无法完全匹配(5张牌)
{[]int{31, 31, 32, 32, 33, 33, 34, 34}, 1, 3}, // 8张实际牌+1张万能牌
{[]int{31, 32, 33, 34}, 8, 4}, // 4张实际牌+8张万能牌
{[]int{31, 31, 32, 32, 33, 33, 34, 34}, 4, 4}, // 8张实际牌+4张万能牌
}
for _, tc := range testCases {
result := matchCards(tc.cards, tc.wild)
fmt.Printf("Cards: %v, Wild: %d, Expected: %d, Got: %d\n",
tc.cards, tc.wild, tc.expected, result)
}
}
实现一个go实现的牌型算法匹配器
原创
©著作权归作者所有:来自51CTO博客作者mb67569ac90cbc1的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:Promtail配置容器日志采集
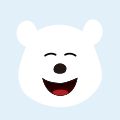
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java实现一个简单的计算器
Java编写一个简单的计算器。
System 运算符 字符串 -
Go 实现一个队列
Go 实现一个队列
golang c++ 开发语言 先进先出 -
用 Go 实现一个 LRU cache
早在几年前写过关于 LRU cache 的文章.
数据 存储数据 链表 -
使用 Go实现一个注册中心
使用 Go 和 HashiCorp Raft 库实现的注册中心的详细代码逻辑示例,包括服务注册、注销、健康检查等功能。
golang 开发语言 后端 json github -
如何实现一个LRU算法?
LRU算法实现的几种方式~
LRU LinkedHashMap