后端代码
1.文件下载工具类 FileUtils
import org.apache.commons.io.IOUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.UUID;
public class FileUtils {
/**
* 输出指定文件的byte数组
* @param filePath 文件路径
* @param os 输出流
* @return
*/
public static void writeBytes(String filePath, OutputStream os) throws IOException
{
FileInputStream fis = null;
try
{
File file = new File(filePath);
if (!file.exists())
{
throw new FileNotFoundException(filePath);
}
fis = new FileInputStream(file);
byte[] b = new byte[1024 * 10];
int length;
while ((length = fis.read(b)) > 0)
{
os.write(b, 0, length);
os.flush();
}
}
catch (IOException e)
{
throw e;
}
finally
{
IOUtils.closeQuietly(os);
IOUtils.closeQuietly(fis);
}
}
}
2.业务层service处理
public void download(Long id, HttpServletResponse response) throws Exception {
PdmDeliverable pdmDeliverable = this.getById(id);
String fileName = pdmDeliverable.getFileName();
//将文件名编码支持中文格式
String encodedFileName = URLEncoder.encode(fileName, StandardCharsets.UTF_8.name()).replaceAll("\\+", "%20");
//设置HTTP响应头以支持下载附件,并指定字符编码为UTF-8
response.setCharacterEncoding("utf-8");
response.setHeader("Content-Disposition", "attachment;filename*=UTF-8''" + encodedFileName);
//设置响应类型为二进制流
response.setContentType(MediaType.APPLICATION_OCTET_STREAM_VALUE);
String filePath = pdmDeliverable.getFilePath();
ServletOutputStream out = response.getOutputStream();
//获取文件路径,将文件内容写入HTTP响应输出流中
FileUtils.writeBytes(filePath,out);
}
3.controller接口
@GetMapping("download")
public Result download(@RequestParam Long id, HttpServletResponse response) {
try {
service.download(id, response);
return new Result().ok();
} catch (Exception e) {
e.printStackTrace();
return new Result().err().setMsg("下载失败");
}
}
前端代码
<el-table :data="tableData" :max-height="tableHeight" @selection-change="handleSelectionChange" stripe
border>
<el-table-column type="selection" width="55" fixed></el-table-column>
<el-table-column type="index" label="序号" width="50"></el-table-column>
<el-table-column prop="fileName" label="交付物" min-width="150">
<template #default="scope">
<a rel="nofollow" @click="download(scope.row)" href="javascript:void(0)">{{ scope.row.fileName }}</a>
</template>
</el-table-column>
<el-table-column prop="partName" label="零件名称" min-width="100"></el-table-column>
<el-table-column prop="processStatus" label="流程状态" min-width="70">
<template #default="scope">
{{ processStatusFormat(scope.row.processStatus) }}
</template>
</el-table-column>
<el-table-column prop="createDate" label="接收日期" min-width="100"></el-table-column>
<el-table-column prop="creatName" label="接收人" min-width="100"></el-table-column>
<el-table-column prop="status" label="状态" min-width="120">
<template #default="scope">
{{ statusFormat(scope.row.status) }}
</template>
</el-table-column>
<el-table-column prop="modifier" label="最后修改人" min-width="100"></el-table-column>
<el-table-column prop="updateDate" label="最后修改时间" min-width="100"></el-table-column>
<el-table-column prop="remark" label="备注" min-width="100"></el-table-column>
<el-table-column fixed="right" label="操作" width="300">
<template #default="scope">
<el-button type="primary" icon="el-icon-edit-outline"
@click="handleEdit(scope.row)">编辑</el-button>
<el-button type="primary" icon="el-icon-document-checked"
@click="handleAudit(scope.row)">审核</el-button>
<el-button type="primary" icon="el-icon-view" @click="showView(scope.row)">详情</el-button>
<i class="el-icon-delete" @click="deleteData(scope.row)"></i>
</template>
</el-table-column>
</el-table>
<el-pagination @size-change="handleSizeChange" @current-change="handleCurrentChange"
:current-page="query.pageNum" :page-sizes="[10, 15, 20, 25, 30]" :page-size="query.pageSize"
layout="total, sizes, prev, pager, next, jumper" :total="total">
</el-pagination>
const download = async (row) => {
try {
const data = {
id: row.id
}
downloadFile(data).then(response => {
if (response.code && response.code == 500) {
ElMessage.error(response.msg)
} else {
// 创建一个URL表示Blob对象
// const url = window.URL.createObjectURL(new Blob([response]));
const url = '/deliverable/download?id=' + row.id;
// 创建隐藏的可下载链接
const link = document.createElement('a');
link.href = url
link.style.display = 'none';
// 将链接添加到DOM中,然后模拟点击下载
document.body.appendChild(link);
link.click();
// 清理工作,释放URL对象
window.URL.revokeObjectURL(url);
link.remove();
}
})
} catch (error) {
ElMessage.error('下载失败')
}
};
实现效果
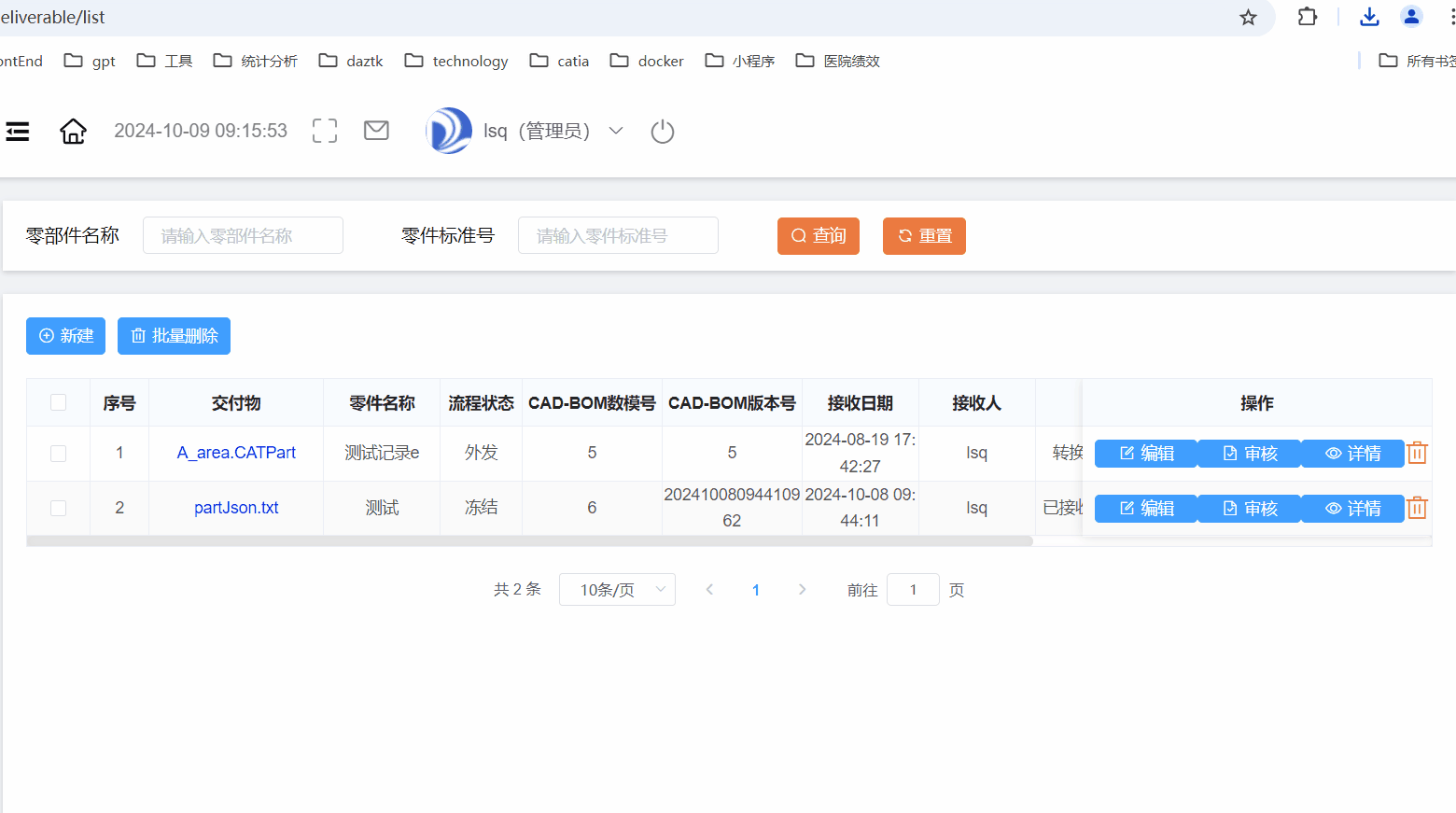