<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="../js/vue.js"></script>
</head>
<body>
<div id="app">
<h1>{{msg}}</h1>
<h1>{{money}}</h1>
<h1>{{getPrice()}}</h1>
<!-- 这样就是price的过滤器写法 -->
<!-- 在VUE3中,过滤器被舍弃了 -->
<!-- 返回值还可以走一遍filter,再次实现过滤 -->
<!-- 第一个位置不用写,会自动传递 -->
<!-- 过滤器只能用在两个位置,一个是插值语法,一个是v-bind中 -->
<h1>{{price | filterA() | filterB(3)}}</h1>
<!-- v-bind也可以使用 -->
<input type="text" :value="price | filterA() | filterB(3)"/>
<!-- v-model是不能用过滤器的 -->
</div>
<div id="app1">
<h1>{{price1 | filterA() | filterB(3)}}</h1>
</div>
<script>
// 配置全局过滤器
Vue.filter("filterA",function(val){
if(val === undefined || val === null || val === '')
{
return '-';
}
return val;
});
Vue.filter("filterB",function(val,number){
// 确保传过来的数据保留两位小数
return val.toFixed(number);
});
const vm = new Vue({
el : "#app",
data : {
msg : "Hello",
price : 50.6
},
methods : {
getPrice()
{
if(this.price === undefined || this.price === null || this.price === '')
{
return '-';
}
return this.price;
}
},
computed : {
money()
{
if(this.price === undefined || this.price === null || this.price === '')
{
return '-';
}
else
{
return this.price;
}
}
},
// 过滤器可以接受value的数据,我们可以在这里进行格式化,而这里因为没有this
// 就可以和业务代码剥离开了,耦合度更低了,复用性更强了
filters : {
// 局部过滤器
// filterA(val){
// if(val === undefined || val === null || val === '')
// {
// return '-';
// }
// return val;
// },
// filterB(val,number){
// // 确保传过来的数据保留两位小数
// return val.toFixed(number);
// }
}
});
const vm1 = new Vue({
el : "#app1",
data : {
price1 : 23
}
});
</script>
</body>
</html>
VUE框架2中的过滤器之局部过滤器和全局过滤器------VUE框架
原创
©著作权归作者所有:来自51CTO博客作者旧约Alatus的原创作品,请联系作者获取转载授权,否则将追究法律责任
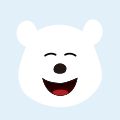
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
设计模式之过滤器模式
过滤器模式
List java System -
vue【过滤器】
过滤器的基本语法,使用Vue.filter定义全局过滤器,使用过滤器格式化时间,过滤器的其他用法
vue.js 前端 javascript 格式化时间 传参数 -
vue 全局过滤器(单个和多个过滤器)
一、单个过滤器 参考 https://cn.vuejs.org/v2/guide/f
Vue 全局过滤器 Math Vue html -
vue过滤器
显示文本摘要,截取文本指定长度: 应用: 代码: filters: { truncate: function (text, length, suffix) { if (text != undefined && text != null && text.length > length) { retur
substring module length filters undefined -
jquery 根据推流地址聊天
一、简介 1.1、概述 随着 WEB2.0及ajax思想在互联网上的快速发展传播,陆续出现了一些优秀的Js框架,其中比较著名的有Prototype、YUI、jQuery、mootools、Bindows以及国内的JSVM框架等,通过将这些JS框架应用到我们的项目中能够使程序员从设计和书写繁杂的JS应用中解脱出来,将关注点转向功能需求而非实现细节上,从
jquery 根据推流地址聊天 jquery function prototype javascript