java-onClick事件未触发 安卓系统
我用一个活动和一个布局制作了一个非常简单的测试应用程序。 editText1不会按其第一次那样触发。
活动:
package com.example.mytest;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final EditText ed1 = (EditText) findViewById(R.id.editText1);
ed1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Toast.makeText(getApplicationContext(), "1", Toast.LENGTH_LONG)
.show();
}
});
}
}
布局:
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10" >
android:id="@+id/editText2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/editText1"
android:ems="10" />
如果运行此应用程序,然后单击第二个editText1,然后再单击第一个,则它将不会触发onClick。您可以继续选择来回选择,它根本不会触发editText2。 我需要此基本功能,但尚未想出使其工作的方法。 有想法吗?
注意
我尝试了在API级别16物理设备和API级别8模拟器上推荐的所有方法,但是遇到了相同的问题。
澄清度
聚焦并单击editText1时,将触发onClick方法。 如果将editText2聚焦,然后单击editText1,则不会触发。 大问题。
EGHDK asked 2020-01-10T03:52:16Z
8个解决方案
186 votes
概述,当用户与任何UI组件进行交互时,将按从上到下的顺序调用各种侦听器。 如果较高优先级的侦听器之一“消费事件”,则较低的侦听器将不会被调用。
在您的情况下,以下三个侦听器按顺序调用:
OnTouchListener
OnFocusChangeListener
OnClickListener
用户第一次触摸EditText时,它将获得焦点,以便用户可以键入。 动作在这里消耗。 因此,不会调用优先级较低的OnClickListener。 每次连续的触摸都不会改变焦点,因此这些事件会滴落到OnClickListener上。
按钮(以及其他类似组件)不会从触摸事件中获得焦点,这就是为什么每次都调用OnClickListener的原因。
基本上,您有三个选择:
自己实现一个OnTouchListener:
v.getContext()
这将在每次触摸EditText时执行。 请注意,侦听器返回v.getContext(),这使事件能够下移到内置的OnFocusChangeListener,后者会更改焦点,以便用户可以键入EditText。
与OnClickListener一起实现OnFocusChangeListener:
v.getContext()
当焦点改变时,此侦听器捕获第一个触摸事件,而OnClickListener捕获其他所有事件。
(这不是一个有效的答案,但是,这是一个很好的技巧。)在XML中将focusable属性设置为v.getContext():
v.getContext()
现在,OnClickListener将在每次单击时触发。 但这使EditText变得无用,因为用户无法再输入任何文本...
注意:
v.getContext()会造成内存泄漏。 一个好的习惯是除非绝对必要,否则避免使用它。 您可以安全地使用v.getContext()。
希望有帮助!
Sam answered 2020-01-10T03:54:53Z
5 votes
使编辑文本可点击。 在XML android:clickable="true"中 或用代码
ed1.setClickable(true);
然后做
ed1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getBaseContext(), "1",Toast.LENGTH_SHORT).show();
}
});
mainu answered 2020-01-10T03:55:17Z
4 votes
我可能参加聚会太晚了,但是下面的代码片段解决了onClick()不被调用的问题:
ed1.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_UP && !v.hasFocus()) {
// onClick() is not called when the EditText doesn't have focus,
// onFocusChange() is called instead, which might have a different
// meaning. This condition calls onClick() when click was performed
// but wasn't reported. Condition can be extended for v.isClickable()
// or v.isEnabled() if needed. Returning false means that everything
// else behaves as before.
v.performClick();
}
return false;
}
});
Matěj Hrazdíra answered 2020-01-10T03:55:37Z
2 votes
发生这种情况是因为第一次轻拍将焦点移到了视图中。 下一次点击会触发点击。
如果要动态放大视图,请执行以下操作:
android:clickable="true"
android:focusable="false"
android:focusableInTouchMode="false"
如果这不起作用,请尝试将其应用于父视图。
Irshu answered 2020-01-10T03:56:06Z
0 votes
public class TestProject extends Activity implements OnClickListener {
TextView txtmsg;
EditText ed1, ed2;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
txtmsg = (TextView) findViewById(R.id.txtmsg);
ed1 = (EditText) findViewById(R.id.edt1);
ed2 = (EditText) findViewById(R.id.edt2);
ed1.setOnClickListener(this);
ed2.setOnClickListener(this);
}
@Override
public void onClick(View v) {
if(v==ed1){
txtmsg.setText("1");
Toast.makeText(this, "first",Toast.LENGTH_SHORT).show();
}
if(v==ed2){
txtmsg.setText("2");
Toast.makeText(this, "second",Toast.LENGTH_SHORT).show();
}
}
}
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
android:id="@+id/edt1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10" />
android:id="@+id/edt2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/edt1"
android:layout_marginTop="14dp"
android:ems="10" />
android:id="@+id/txtmsg"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignRight="@+id/edt2"
android:layout_below="@+id/edt2"
android:layout_marginRight="22dp"
android:layout_marginTop="160dp"
android:textAppearance="?android:attr/textAppearanceLarge" />
Yash answered 2020-01-10T03:56:26Z
0 votes
花了我几分钟来解决这个问题。 我的ImageButton和setOnClickListener和onClick似乎没有触发,然后我意识到它实际上位于xml布局中的另一个元素之下,所以我将其转为:
到这个:
突然之间,ImageButton没有被其他布局所覆盖,因为现在它稍后被添加到父布局中,并且位于顶部并且每次都可以工作。 祝您好运,当基本的东西突然停止工作时,总是很有趣
ColossalChris answered 2020-01-10T03:56:55Z
0 votes
避免使用onClick,因为它在您不需要时(例如,当您进入活动时)表现不正常。 只需将OnClickListener设置为OnTouchListener,如下所示:
@Override
public boolean onTouch(View view, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
view.requestFocus();
break;
}
return false;
}
这将使您的EditText首先获得焦点,而onClick则在第一次正常运行。
Andrei Lupsa answered 2020-01-10T03:57:20Z
0 votes
这是使用日期选择器的最简单方法。
private DatePickerDialog datePickerDialog;
EditText etJoiningDate;
etJoiningDate=(EditText)findViewById(R.id.etJoiningDate);
etJoiningDate.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
switch (event.getAction()){
case MotionEvent.ACTION_DOWN:
final Calendar cldr = Calendar.getInstance();
int day = cldr.get(Calendar.DAY_OF_MONTH);
int month = cldr.get(Calendar.MONTH);
int year = cldr.get(Calendar.YEAR);
// date picker dialog
datePickerDialog = new DatePickerDialog(TestActivity.this,
new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
etJoiningDate.setText(dayOfMonth + "/" + (monthOfYear + 1) + "/" + year);
}
}, year, month, day);
datePickerDialog.show();
break;
}
return false;
}
});
Hasan Masud answered 2020-01-10T03:57:40Z
java图片抖点算法
转载文章标签 java图片抖点算法 安卓java浮层不响应点击事件 android Text ide 文章分类 Java 后端开发
下一篇:Python怎么切片函数
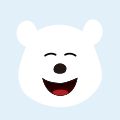
-
sigmoid 定点算法
定点算术原理在计算中,可以使用一对整数来近似分数(n,e),此整数对表示分数 e被看作放置在n中所移动的位数。 eg: 浮点&&定点: e是可变量,保存在寄存器中且在编译时未知,则数为浮点数; &nb
sigmoid 定点算法 arm #define 定点数 宏定义