1://获取当前方法的名称
String methodName = Thread.currentThread().getStackTrace()[1].getMethodName();
2:字符串和整形数据之间 的想换转换
String a = String.valueOf(2);
int i = Integer.parseInt(a);
3:文件末尾添加数据内容
BufferedWriter out = null ;
try {
out = new BufferedWriter( new FileWriter(”filename”, true ));
out.write(”aString”);
} catch (IOException e) {
// error processing code
} finally {
if (out != null ) {
out.close();
}
}
4:使用NIO快速拷贝文件
public static void fileCopy( File in, File out )
throws IOException
{
FileChannel inChannel = new FileInputStream( in ).getChannel();
FileChannel outChannel = new FileOutputStream( out ).getChannel();
try
{
// inChannel.transferTo(0, inChannel.size(), outChannel); // original -- apparently has trouble copying large files on Windows
// magic number for Windows, 64Mb - 32Kb)
int maxCount = ( 64 * 1024 * 1024 ) - ( 32 * 1024 );
long size = inChannel.size();
long position = 0 ;
while ( position < size )
{
position += inChannel.transferTo( position, maxCount, outChannel );
}
}
finally
{
if ( inChannel != null )
{
inChannel.close();
}
if ( outChannel != null )
{
outChannel.close();
}
}
}
5:创建图片缩略图
private void createThumbnail(String filename, int thumbWidth, int thumbHeight, int quality, String outFilename)
throws InterruptedException, FileNotFoundException, IOException
{
// load image from filename
Image image = Toolkit.getDefaultToolkit().getImage(filename);
MediaTracker mediaTracker = new MediaTracker( new Container());
mediaTracker.addImage(image, 0 );
mediaTracker.waitForID( 0 );
// use this to test for errors at this point: System.out.println(mediaTracker.isErrorAny());
// determine thumbnail size from WIDTH and HEIGHT
double thumbRatio = ( double )thumbWidth / ( double )thumbHeight;
int imageWidth = image.getWidth( null );
int imageHeight = image.getHeight( null );
double imageRatio = ( double )imageWidth / ( double )imageHeight;
if (thumbRatio < imageRatio) {
thumbHeight = ( int )(thumbWidth / imageRatio);
} else {
thumbWidth = ( int )(thumbHeight * imageRatio);
}
// draw original image to thumbnail image object and
// scale it to the new size on-the-fly
BufferedImage thumbImage = new BufferedImage(thumbWidth, thumbHeight, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics2D = thumbImage.createGraphics();
graphics2D.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
graphics2D.drawImage(image, 0 , 0 , thumbWidth, thumbHeight, null );
// save thumbnail image to outFilename
BufferedOutputStream out = new BufferedOutputStream( new FileOutputStream(outFilename));
JPEGImageEncoder encoder = JPEGCodec.createJPEGEncoder(out);
JPEGEncodeParam param = encoder.getDefaultJPEGEncodeParam(thumbImage);
quality = Math.max( 0 , Math.min(quality, 100 ));
param.setQuality(( float )quality / 100 .0f, false );
encoder.setJPEGEncodeParam(param);
encoder.encode(thumbImage);
out.close();
}
java的常用 java的常用方法
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
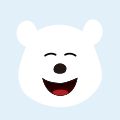
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java常用数据结构的常用方法
注意:操作符(+ == !=)只能作用在基础数据类型上,唯一一个特例是String类,而且String还只能用+ 表示连接两个字符串...
java 字符串 包装类 浅拷贝 正则表达式