import java.applet.*;
import java.awt.*;
import java.io.*;
import java.net.*;
import java.awt.event.*;
public class ChatClient extends Applet{
protected boolean loggedIn;//登入状态
protected Frame cp;//聊天室框架
protected static int PORTNUM=7777; //缺省端口号7777
protected int port;//实际端口号
protected Socket sock;
protected BufferedReader is;//用于从sock读取数据的BufferedReader
protected PrintWriter pw;//用于向sock写入数据的PrintWriter
protected TextField tf;//用于输入的TextField
protected TextArea ta;//用于显示对话的TextArea
protected Button lib;//登入按钮
protected Button lob;//登出的按钮
final static String TITLE ="Chatroom applet>>>>>>>>>>>>>>>>>>>>>>>>";
protected String paintMessage;//发表的消息
public ChatParameter Chat;
public void init(){
paintMessage="正在生成聊天窗口";
repaint();
cp=new Frame(TITLE);
cp.setLayout(new BorderLayout());
String portNum=getParameter("port");//呢个参数勿太明
port=PORTNUM;
if (portNum!=null) //书上是portNum==null,十分有问题
port=Integer.parseInt(portNum);
//CGI
ta=new TextArea(14,80);
ta.setEditable(false);//read only attribute
ta.setFont(new Font("Monospaced",Font.PLAIN,11));
cp.add(BorderLayout.NORTH,ta);
Panel p=new Panel();
Button b;
//for login button
p.add(lib=new Button("Login"));
lib.setEnabled(true);
lib.requestFocus();
lib.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e){
login();
lib.setEnabled(false);
lob.setEnabled(true);
tf.requestFocus();//将键盘输入锁定再右边的文本框中
}
});
//for logout button
p.add(lob=new Button ("Logout"));
lob.setEnabled(false);
lob.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
logout();
lib.setEnabled(true);
lob.setEnabled(false);
lib.requestFocus();
}
});
p.add(new Label ("输入消息:"));
tf=new TextField(40);
tf.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
if(loggedIn){
//pw.println(Chat.CMD_BCAST+tf.getText());//Chat.CMD....是咩野来?
int j=tf.getText().indexOf(":");
if(j>0) pw.println(Chat.CMD_MESG+tf.getText());
else
pw.println(Chat.CMD_BCAST+tf.getText());
tf.setText("");//勿使用flush()?
}
}
});
p.add(tf);
cp.add(BorderLayout.SOUTH,p);
cp.addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent e){
//如果执行了setVisible或者dispose,关闭窗口
ChatClient.this.cp.setVisible(false);
ChatClient.this.cp.dispose();
logout();
}
});
cp.pack();//勿明白有咩用?
//将Frame cp放在中间
Dimension us=cp.getSize(),
them=Toolkit.getDefaultToolkit().getScreenSize();
int newX=(them.width-us.width)/2;
int newY=(them.height-us.height)/2;
cp.setLocation(newX,newY);
cp.setVisible(true);
paintMessage="Window should now be visible";
repaint();
}
//登录聊天室
public void login(){
if(loggedIn) return;
try{
sock=new Socket(getCodeBase().getHost(),port);
is=new BufferedReader(new InputStreamReader(sock.getInputStream()));
pw=new PrintWriter(sock.getOutputStream(),true);
}catch(IOException e){
showStatus("Can't get socket: "+e);
cp.add(new Label("Can't get socket: "+e));
return;}
//构造并且启动读入器,从服务器读取数据,输出到文本框中
//这里,长成一个线程来避免锁住资源(lockups)
new Thread (new Runnable(){
public void run(){
String line;
try{
while(loggedIn &&((line=is.readLine())!=null))
ta.appendText(line+"/n");
}catch(IOException e){
showStatus("我的天啊,掉线了也!!!!");
return;
}
}
}).start();
//假定登录(其实只是打印相关信息,并没有真正登录)
// pw.println(Chat.CMD_LOGIN+"AppletUser");
pw.println(Chat.CMD_LOGIN+"AppletUser");
loggedIn =true; }
//模仿退出的代码
public void logout(){
if(!loggedIn)
return;
loggedIn=false;
try{
if(sock!=null)
sock.close();
}catch(IOException ign){
// 异常处理哦
}
}
//没有设置stop的方法,即使从浏览器跳到另外一个网页的时候
//聊天程序还可以继续运行
public void paint(Graphics g){
Dimension d=getSize();
int h=d.height;
int w=d.width;
g.fillRect(0,0,w,2);
g.setColor(Color.black);
g.drawString(paintMessage,10,(h/2)-5);
}
}
聊天室服务器端
import java.net.*;
import java.io.*;
import java.util.*; public class ChatServer{
//聊天室管理员ID
protected final static String CHATMASTER_ID="ChatMaster";
//系统信息的分隔符
protected final static String SEP=": ";
//服务器的Socket
protected ServerSocket servSock;
//当前客户端列表
protected ArrayList clients;
//调试标记
protected boolean DEBUG=false;
public ChatParameter Chat; //主方法构造一个ChatServer,没有返回值
public static void main(String[] argv){
System.out.println("Chat server0.1 starting>>>>>>>>>>>>>>>>");
ChatServer w=new ChatServer();
w.runServer();
System.out.println("***ERROR*** Chat server0.1 quitting"); }
//构造和运行一个聊天服务
ChatServer(){
Chat=new ChatParameter();
clients=new ArrayList();
try{
servSock=new ServerSocket(7777);//实有问题拉,不过可能是他自己定义既一个class.
System.out.println("Chat Server0.1 listening on port:"+7777);
}catch(Exception e){
log("IO Exception in ChatServer.<init>");
System.exit(0);
}
} public void runServer(){
try{
while(true){
Socket us=servSock.accept();
String hostName=us.getInetAddress().getHostName();
System.out.println("Accpeted from "+hostName);
//一个处理的线程
ChatHandler cl=new ChatHandler(us,hostName);
synchronized(clients){
clients.add(cl);
cl.start();
if(clients.size()==1)
cl.send(CHATMASTER_ID,"Welcome!You are the first one here");
else{
cl.send(CHATMASTER_ID,"Welcome!You are the latest of"+
clients.size()+" users.");
}
}
}
}catch(Exception e){
log("IO Exception in runServer:"+e);
System.exit(0);
}
} protected void log(String s){
System.out.println(s);
} //处理会话的内部的类
protected class ChatHandler extends Thread {
//客户端scoket
protected Socket clientSock;
//读取socket的BufferedReader
protected BufferedReader is ;
//在socket 上发送信息行的PrintWriter
protected PrintWriter pw;
//客户端出主机
protected String clientIP;
//句柄
protected String login; public ChatHandler (Socket sock,String clnt)throws IOException {
clientSock=sock;
clientIP=clnt;
is=new BufferedReader(
new InputStreamReader(sock.getInputStream()));
pw=new PrintWriter (sock.getOutputStream(),true); }
//每一个ChatHandler是一个线程,下面的是他的run()方法
//用于处理会话
public void run(){
String line; try{
while((line=is.readLine())!=null){
char c=line.charAt(0);// ,果只Chat.CMD咩xx冇定义 扑啊///!!!
line=line.substring(1);
switch(c){
//case Chat.CMD_LOGIN:
case 'l':
if(!Chat.isValidLoginName(line)){
send(CHATMASTER_ID,"LOGIN"+line+"invalid");
log("LOGIN INVALID from:"+clientIP);
continue;
} login=line;
broadcast(CHATMASTER_ID,login+" joins us,for a total of"+
clients.size()+" users");
break; // case Chat.CMD_MESG:
case 'm':
if(login==null){
send(CHATMASTER_ID,"please login first");
continue;
} int where =line.indexOf(Chat.SEPARATOR);
String recip=line.substring(0,where);
String mesg=line.substring (where+1);
log("MESG: "+login+"--->"+recip+": "+mesg);
ChatHandler cl=lookup(recip);
if(cl==null)
psend(CHATMASTER_ID,recip+"not logged in.");
else
cl.psend(login,mesg); break;
//case Chat.CMD_QUIT:
case 'q':
broadcast(CHATMASTER_ID,"Goodbye to "+login+"@"+clientIP);
close();
return;//ChatHandler结束 // case Chat.CMD_BCAST:
case 'b':
if(login!=null)
broadcast(login,line);
else
log("B<L FROM"+clientIP);
break; default:
log("Unknow cmd"+c+"from"+login+"@"+clientIP);
}
}
}catch(IOException e){
log("IO Exception :"+e);
}finally{
//sock 结束,我们完成了
//还不能发送再见的消息
//得有简单的基于命令的协议才行
System.out.println(login+SEP+"All Done");
synchronized(clients){
clients.remove(this);
if(clients.size()==0){
System.out.println(CHATMASTER_ID+SEP+
"I'm so lonely I could cry>>>>>");
}else if(clients.size()==1){
ChatHandler last=(ChatHandler)clients.get(0);
last.send(CHATMASTER_ID,"Hey,you are talking to yourself again");
}
else{
broadcast(CHATMASTER_ID,"There are now"+clients.size()+" users");
}
}
}
}
protected void close(){
if(clientSock==null){
log("close when not open");
return;
}
try{
clientSock.close();
clientSock=null;
}catch(IOException e){
log("Failure during close to "+clientIP);
}
}
//发送一条消息给用户
public void send(String sender,String mesg){
pw.println(sender+SEP+"*>"+mesg);
}
//发送私有的消息
protected void psend(String sender ,String msg){
send("<*"+sender+"*>",msg);
}
//发送一条消息给所有的用户
public void broadcast (String sender,String mesg){
System.out.println("Broadcasting"+sender+SEP+mesg);
for(int i=0;i<clients.size();i++){
ChatHandler sib=(ChatHandler)clients.get(i);
if(DEBUG)
System.out.println("Sending to"+sib);
sib.send(sender,mesg);
}
if(DEBUG) System.out.println("Done broadcast");
}
protected ChatHandler lookup(String nick){
synchronized(clients){
for(int i=0;i<clients.size();i++){
ChatHandler cl=(ChatHandler)clients.get(i);
if(cl.login.equals(nick))
return cl;
}
}
return null;
}
//将ChatHandler对象转换成一个字符串
public String toString(){
return "ChatHandler["+login+"]";
}
}
}
public class ChatParameter {
public static final char CMD_BCAST='b';
public static final char CMD_LOGIN='l';
public static final char CMD_MESG='m';
public static final char CMD_QUIT='q';
public static final char SEPARATOR=':';//?????
public static final int PORTNUM=7777;
public boolean isValidLoginName(String line){
if (line.equals("CHATMASTER_ID"))
return false;
return true;
}
public void main(String[] argv){
}
}
!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
import java.awt.*;
import java.net.*;
import java.awt.event.*;
import java.io.*;
import java.util.Hashtable; public class ChatArea extends Panel implements ActionListener,Runnable
{
Socket socket=null;
DataInputStream in=null;
DataOutputStream out=null;
Thread threadMessage=null;
TextArea 谈话显示区,私聊显示区=null;
TextField 送出信息=null;
Button 确定,刷新谈话区,刷新私聊区;
Label 提示条=null;
String name=null;
Hashtable listTable;
List listComponent=null;
Choice privateChatList;
int width,height;
public ChatArea(String name,Hashtable listTable,int width,int height)
{
setLayout(null);
setBackground(Color.orange);
this.width=width;
this.height=height;
setSize(width,height);
this.listTable=listTable;
this.name=name;
threadMessage=new Thread(this);
谈话显示区=new TextArea(10,10);
私聊显示区=new TextArea(10,10);
确定=new Button("送出信息到:");
刷新谈话区=new Button("刷新谈话区");
刷新私聊区=new Button("刷新私聊区");
提示条=new Label("双击聊天者可私聊",Label.CENTER);
送出信息=new TextField(28);
确定.addActionListener(this);
送出信息.addActionListener(this);
刷新谈话区.addActionListener(this);
刷新私聊区.addActionListener(this);
listComponent=new List();
listComponent.addActionListener(this);
privateChatList=new Choice();
privateChatList.add("大家(*)");
privateChatList.select(0); add(谈话显示区);
谈话显示区.setBounds(10,10,(width-120)/2,(height-120));
add(私聊显示区);
私聊显示区.setBounds(10+(width-120)/2,10,(width-120)/2,(height-120));
add(listComponent);
listComponent.setBounds(10+(width-120),10,100,(height-160));
add(提示条);
提示条.setBounds(10+(width-120),10+(height-160),110,40);
Panel pSouth=new Panel();
pSouth.add(送出信息);
pSouth.add(确定);
pSouth.add(privateChatList);
pSouth.add(刷新谈话区);
pSouth.add(刷新私聊区);
add(pSouth);
pSouth.setBounds(10,20+(height-120),width-20,60); }
public void setName(String s)
{
name=s;
}
public void setSocketConnection(Socket socket,DataInputStream in,DataOutputStream out)
{
this.socket=socket;
this.in=in;
this.out=out;
try
{
threadMessage.start();
}
catch(Exception e)
{
}
}
public void actionPerformed(ActionEvent e)
{ if(e.getSource()==确定||e.getSource()==送出信息)
{
String message="";
String people=privateChatList.getSelectedItem();
people=people.substring(0,people.indexOf("("));
message=送出信息.getText();
if(message.length()>0)
{
try {
if(people.equals("大家"))
{
out.writeUTF("公共聊天内容:"+name+"说:"+message);
}
else
{
out.writeUTF("私人聊天内容:"+name+"悄悄地说:"+message+"#"+people);
}
}
catch(IOException event)
{
}
}
}
else if(e.getSource()==listComponent)
{
privateChatList.insert(listComponent.getSelectedItem(),0);
privateChatList.repaint();
}
else if(e.getSource()==刷新谈话区)
{
谈话显示区.setText(null);
}
else if(e.getSource()==刷新私聊区)
{
私聊显示区.setText(null);
}
}
public void run()
{
while(true)
{
String s=null;
try
{
s=in.readUTF();
if(s.startsWith("聊天内容:"))
{
String content=s.substring(s.indexOf(":")+1);
谈话显示区.append("/n"+content);
}
if(s.startsWith("私人聊天内容:"))
{
String content=s.substring(s.indexOf(":")+1);
私聊显示区.append("/n"+content);
}
else if(s.startsWith("聊天者:"))
{
String people=s.substring(s.indexOf(":")+1,s.indexOf("性别"));
String sex=s.substring(s.indexOf("性别")+2); listTable.put(people,people+"("+sex+")");
listComponent.add((String)listTable.get(people));
listComponent.repaint();
}
else if(s.startsWith("用户离线:"))
{
String awayPeopleName=s.substring(s.indexOf(":")+1);
listComponent.remove((String)listTable.get(awayPeopleName));
listComponent.repaint();
谈话显示区.append("/n"+(String)listTable.get(awayPeopleName)+"离线");
listTable.remove(awayPeopleName);
}
Thread.sleep(5);
}
catch(IOException e)
{
listComponent.removeAll();
listComponent.repaint();
listTable.clear();
谈话显示区.setText("和服务器的连接已中断/n必须刷新浏览器才能再次聊天");
break;
}
catch(InterruptedException e)
{
}
}
}
} ChatServer.java
import java.io.*;
import java.net.*;
import java.util.*;
public class ChatServer
{
public static void main(String args[])
{
ServerSocket server=null;
Socket you=null;
Hashtable peopleList;
peopleList=new Hashtable();
while(true)
{
try
{
server=new ServerSocket(6666);
}
catch(IOException e1)
{
System.out.println("正在监听");
}
try {
you=server.accept();
InetAddress address=you.getInetAddress();
System.out.println("用户的IP:"+address); }
catch (IOException e)
{
}
if(you!=null)
{
Server_thread peopleThread=new Server_thread(you,peopleList);
peopleThread.start();
}
else {
continue;
}
}
}
}
class Server_thread extends Thread
{
String name=null,sex=null;
Socket socket=null;
File file=null;
DataOutputStream out=null;
DataInputStream in=null;
Hashtable peopleList=null;
Server_thread(Socket t,Hashtable list)
{
peopleList=list;
socket=t;
try {
in=new DataInputStream(socket.getInputStream());
out=new DataOutputStream(socket.getOutputStream());
}
catch (IOException e)
{
}
}
public void run()
{ while(true)
{ String s=null;
try
{
s=in.readUTF();
if(s.startsWith("姓名:"))
{
name=s.substring(s.indexOf(":")+1,s.indexOf("性别"));
sex=s.substring(s.lastIndexOf(":")+1); boolean boo=peopleList.containsKey(name);
if(boo==false)
{
peopleList.put(name,this);
out.writeUTF("可以聊天:");
Enumeration enum=peopleList.elements();
while(enum.hasMoreElements())
{
Server_thread th=(Server_thread)enum.nextElement();
th.out.writeUTF("聊天者:"+name+"性别"+sex); if(th!=this)
{
out.writeUTF("聊天者:"+th.name+"性别"+th.sex);
}
} }
else
{
out.writeUTF("不可以聊天:");
}
}
else if(s.startsWith("公共聊天内容:"))
{
String message=s.substring(s.indexOf(":")+1);
Enumeration enum=peopleList.elements();
while(enum.hasMoreElements())
{
((Server_thread)enum.nextElement()).out.writeUTF("聊天内容:"+message);
}
} else if(s.startsWith("用户离开:"))
{
Enumeration enum=peopleList.elements();
while(enum.hasMoreElements())
{ try
{
Server_thread th=(Server_thread)enum.nextElement();
if(th!=this&&th.isAlive())
{
th.out.writeUTF("用户离线:"+name);
}
}
catch(IOException eee)
{
}
}
peopleList.remove(name);
socket.close();
System.out.println(name+"用户离开了");
break;
}
else if(s.startsWith("私人聊天内容:"))
{
String 悄悄话=s.substring(s.indexOf(":")+1,s.indexOf("#"));
String toPeople=s.substring(s.indexOf("#")+1); Server_thread toThread=(Server_thread)peopleList.get(toPeople);
if(toThread!=null)
{
toThread.out.writeUTF("私人聊天内容:"+悄悄话);
}
else
{
out.writeUTF("私人聊天内容:"+toPeople+"已经离线");
}
}
}
catch(IOException ee)
{
Enumeration enum=peopleList.elements();
while(enum.hasMoreElements())
{ try
{
Server_thread th=(Server_thread)enum.nextElement();
if(th!=this&&th.isAlive())
{
th.out.writeUTF("用户离线:"+name);
}
}
catch(IOException eee)
{
}
}
peopleList.remove(name);
try
{
socket.close();
}
catch(IOException eee)
{
} System.out.println(name+"用户离开了");
break;
}
}
}
} import java.awt.*;
import java.io.*;
import java.net.*;
import java.applet.*;
import java.util.Hashtable; ClientChat.java
public class ClientChat extends Applet implements Runnable
{
Socket socket=null;
DataInputStream in=null;
DataOutputStream out=null;
InputNameTextField 用户提交昵称界面=null;
ChatArea 用户聊天界面=null;
Hashtable listTable;
Label 提示条;
Panel north, center;
Thread thread;
public void init()
{
int width=getSize().width;
int height=getSize().height;
listTable=new Hashtable();
setLayout(new BorderLayout());
用户提交昵称界面=new InputNameTextField(listTable);
int h=用户提交昵称界面.getSize().height;
用户聊天界面=new ChatArea("",listTable,width,height-(h+5));
用户聊天界面.setVisible(false);
提示条=new Label("正在连接到服务器,请稍等...",Label.CENTER);
提示条.setForeground(Color.red);
north=new Panel(new FlowLayout(FlowLayout.LEFT));
center=new Panel();
north.add(用户提交昵称界面);
north.add(提示条);
center.add(用户聊天界面);
add(north,BorderLayout.NORTH);
add(center,BorderLayout.CENTER);
validate();
}
public void start()
{
if(socket!=null&&in!=null&&out!=null)
{ try
{
socket.close();
in.close();
out.close();
用户聊天界面.setVisible(false); }
catch(Exception ee)
{
}
}
try
{
socket = new Socket(this.getCodeBase().getHost(), 6666);
in=new DataInputStream(socket.getInputStream());
out=new DataOutputStream(socket.getOutputStream());
}
catch (IOException ee)
{
提示条.setText("连接失败");
}
if(socket!=null)
{
InetAddress address=socket.getInetAddress();
提示条.setText("连接:"+address+"成功");
用户提交昵称界面.setSocketConnection(socket,in,out);
north.validate();
}
if(thread==null)
{
thread=new Thread(this);
thread.start();
}
} public void stop()
{
try
{
socket.close();
thread=null;
}
catch(IOException e)
{
this.showStatus(e.toString());
}
}
public void run()
{
while(thread!=null)
{
if(用户提交昵称界面.get能否聊天()==true)
{
用户聊天界面.setVisible(true);
用户聊天界面.setName(用户提交昵称界面.getName());
用户聊天界面.setSocketConnection(socket,in,out);
提示条.setText("祝聊天愉快!");
center.validate();
break;
}
try
{
Thread.sleep(100);
}
catch(Exception e)
{
}
}
}
}
InputNameTextField。java
import java.awt.*;
import java.net.*;
import java.awt.event.*;
import java.io.*;
import java.util.Hashtable;
public class InputNameTextField extends Panel implements ActionListener,Runnable
{
TextField nameFile=null;
String name=null;
Checkbox male=null,female=null;
CheckboxGroup group=null;
Button 进入聊天室=null,退出聊天室=null;
Socket socket=null;
DataInputStream in=null;
DataOutputStream out=null;
Thread thread=null;
boolean 能否聊天=false;
Hashtable listTable;
public InputNameTextField(Hashtable listTable)
{
this.listTable=listTable;
nameFile=new TextField(10);
group=new CheckboxGroup();
male=new Checkbox("男",true,group);
female=new Checkbox("女",false,group);
进入聊天室=new Button("进入");
退出聊天室=new Button("退出");
进入聊天室.addActionListener(this);
退出聊天室.addActionListener(this);
thread=new Thread(this);
add(new Label("昵称:"));
add(nameFile);
add(male);
add(female);
add(进入聊天室);
add(退出聊天室);
退出聊天室.setEnabled(false);
}
public void set能否聊天(boolean b)
{
能否聊天=b;
}
public boolean get能否聊天()
{
return 能否聊天;
}
public String getName()
{
return name;
}
public void setName(String s)
{
name=s;
}
public void setSocketConnection(Socket socket,DataInputStream in,DataOutputStream out)
{ this.socket=socket;
this.in=in;
this.out=out;
try{
thread.start();
}
catch(Exception e)
{
nameFile.setText(""+e);
}
}
public Socket getSocket()
{
return socket;
}
public void actionPerformed(ActionEvent e)
{
if(e.getSource()==进入聊天室)
{
退出聊天室.setEnabled(true);
if(能否聊天==true)
{
nameFile.setText("您正在聊天:"+name);
}
else
{
this.setName(nameFile.getText());
String sex=group.getSelectedCheckbox().getLabel();
if(socket!=null&&name!=null)
{
try{
out.writeUTF("姓名:"+name+"性别:"+sex);
}
catch(IOException ee)
{
nameFile.setText("没有连通服务器"+ee);
}
}
}
}
if(e.getSource()==退出聊天室)
{
try
{
out.writeUTF("用户离开:");
}
catch(IOException ee)
{
}
}
}
public void run()
{
String message=null;
while(true)
{
if(in!=null)
{
try
{
message=in.readUTF();
}
catch(IOException e)
{
nameFile.setText("和服务器断开"+e);
}
}
if(message.startsWith("可以聊天:"))
{
能否聊天=true;
break;
}
else if(message.startsWith("聊天者:"))
{
String people=message.substring(message.indexOf(":")+1);
listTable.put(people,people);
}
else if(message.startsWith("不可以聊天:"))
{
能否聊天=false;
nameFile.setText("该昵称已被占用");
}
}
}
}
!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
import java.awt.*;
import java.awt.event.*;
import javax.swing.*; import java.io.*;
import java.net.*;
public class liaotian extends JFrame implements ActionListener{
public static void main(String[] args){
liaotian frame=new liaotian();
}
JButton command,command2,command3;
JRadioButton rb[]=new JRadioButton[2];
JTextArea ta1;
JTextField tf1,tf2,tf3;
ServerSocket socket1;
Socket insocket1,socket2;
String inbuf;
BufferedReader in1;
PrintWriter out1;
mt625_server t1;
mt625_client t2;
public liaotian(){
super("聊天");
Container c=getContentPane();
c.setLayout(null);
JLabel lb=new JLabel("TCP通信程序");
lb.setFont(new Font("宋体",Font.BOLD,16));
lb.setForeground(Color.black);
lb.setSize(2000,20);
lb.setLocation(10,2);
c.add(lb);
String str1[]={"服务端","客户端"};
ButtonGroup bg1=new ButtonGroup();
for(int i=0;i<2;i++){
rb[i]=new JRadioButton(str1[i]);
rb[i].setFont(new Font("宋体",Font.BOLD,14));
rb[i].setForeground(Color.black);
rb[i].setSize(80,20);
rb[i].setLocation(10+i*80,27);
c.add(rb[i]);
bg1.add(rb[i]);
}
rb[0].setSelected(true);
JLabel lb1=new JLabel("连接主机IP");
lb1.setFont(new Font("宋体",Font.BOLD,16));
lb1.setForeground(Color.black);
lb1.setSize(120,25);
lb1.setLocation(16,55);
c.add(lb1);
tf1=new JTextField("59.68.255.27");
tf1.setForeground(Color.black);
tf1.setSize(250,25);
tf1.setLocation(120,55);
c.add(tf1);
command=new JButton("连接");
command.setFont(new Font("宋体",Font.BOLD,16));
command.setSize(110,20);
command.setLocation(380,55);
command.addActionListener(this);
c.add(command);
JLabel lb2=new JLabel("接收到信息");
lb2.setFont(new Font("宋体",Font.BOLD,16));
lb2.setForeground(Color.black);
lb2.setSize(120,20);
lb2.setLocation(10,85);
c.add(lb2);
ta1=new JTextArea();
ta1.setForeground(Color.black);
ta1.setSize(250,200);
ta1.setLocation(120,85);
c.add(ta1);
JLabel lb3=new JLabel("发送信息");
lb3.setFont(new Font("宋体",Font.BOLD,16));
lb3.setForeground(Color.black);
lb3.setSize(120,25);
lb3.setLocation(10,300);
c.add(lb3);
tf2=new JTextField();
tf2.setForeground(Color.black);
tf2.setSize(250,25);
tf2.setLocation(120,300);
c.add(tf2);
command2=new JButton("发送信息");
command2.setFont(new Font("宋体",Font.BOLD,16));
command2.setSize(110,25);
command2.setLocation(380,300);
command2.addActionListener(this);
command2.setEnabled(false);
c.add(command2);
JLabel lb4=new JLabel("连接状态: ");
lb4.setFont(new Font("宋体",Font.BOLD,14));
lb4.setForeground(Color.black);
lb4.setSize(120,25);
lb4.setLocation(180,27);
c.add(lb4);
tf3=new JTextField("离线");
tf3.setForeground(Color.black);
tf3.setSize(120,25);
tf3.setLocation(270,27);
c.add(tf3);
command3=new JButton("结束连接");
command3.setFont(new Font("宋体",Font.BOLD,16));
command3.setSize(110,20);
command3.setLocation(380,85);
command3.addActionListener(this);
command3.setEnabled(false);
c.add(command3);
t1=new mt625_server();
t2=new mt625_client();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500,400);
setVisible(true);
setLocation(300,300); }
public void actionPerformed(ActionEvent e)
{
if(e.getSource()==command){
try{
if(rb[0].isSelected()==true){
inbuf="";
tf2.setText("");
t1.start();
}
else{
inbuf="";
tf2.setText("");
t2.start();
}
}
catch (Exception e2) {tf3.setText("发生错误");}
}
if(e.getSource()==command2){
out1.write(tf2.getText()+"/n");
out1.flush();
tf2.setText("");
}
if(e.getSource()==command3){
try{
if(rb[0].isSelected()==true){
insocket1.close();
tf3.setText("离线");
command2.setEnabled(false);
command3.setEnabled(false);
}
else{
socket2.close();
tf3.setText("离线!");
command2.setEnabled(false);
command3.setEnabled(false);
}
}catch (Exception e2) {tf3.setText("发生错误");}
}
}
class mt625_server extends Thread{
public mt625_server(){}
public void run(){
try{
command.setEnabled(false);
tf3.setText("正在等待连接!");
tf1.setText(Inet4Address.getLocalHost().getHostAddress());
socket1=new ServerSocket(21);
insocket1=socket1.accept();
in1=new BufferedReader(new InputStreamReader(insocket1.getInputStream()));
out1=new PrintWriter(insocket1.getOutputStream(),true); while(true){
if(socket1.isBound()==true){
tf3.setText("正在连接!");
command2.setEnabled(true);
command3.setEnabled(true);
break;
}
}
while(true){
inbuf=in1.readLine();
if(inbuf.length()>0)
{
ta1.append(inbuf);
ta1.append("/n");
}
}
}
catch(Exception e){}
}
}
class mt625_client extends Thread{
public mt625_client(){}
public void run(){
try{
command.setEnabled(false);
tf3.setText("正在等待连接!");
socket2=new Socket();
socket2.connect(new InetSocketAddress(tf1.getText(),21),5000);
in1=new BufferedReader(new InputStreamReader(socket2.getInputStream()));
out1=new PrintWriter(socket2.getOutputStream(),true);
while(true){
if(socket2.isConnected()==true){
tf3.setText("正在连接!");
command2.setEnabled(true);
command3.setEnabled(true);
break;
}
}
inbuf="";
while(true){
inbuf=in1.readLine();
if(inbuf.length()>0)
{
ta1.append(inbuf);
ta1.append("/n");
}
}
}
catch(Exception e){}
}
} }
java 控制台 聊天窗 java聊天窗口代码
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
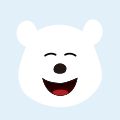
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
控制台打印进度条
控制台打印进度条,实时展示任务进度。
进度条 System Java -
websockt一对一聊天java部分
websocket
java websocket -
sys输出python路径
模块—文件。搜索路径: 搜索路径是一个目录列表,在这些目录中搜索想要加载的模块。 PYTHONPATH环境变量:冒号分割的目录路径。 s
sys输出python路径 Python 加载 搜索