代码
–
AES128Activity
import android.app.Activity;
import android.content.Context;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import tsou.com.encryption.R;
import tsou.com.encryption.aes128.AlgorithmUtil;
/**
• 自动生成base64密钥
*/
public class AES128Activity extends AppCompatActivity implements View.OnClickListener {
private EditText encryptionContext;
private Button encryption;
private TextView tvEncryption;
private Button decode;
private TextView tvDecode;
private Activity mActivity;
private Context mContext;
private byte[] encoded;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_aes);
mActivity = this;
mContext = this;
encryptionContext = (EditText) findViewById(R.id.et_encryption_context);
encryption = (Button) findViewById(R.id.btn_encryption);
tvEncryption = (TextView) findViewById(R.id.tv_encryption);
decode = (Button) findViewById(R.id.btn_decode);
tvDecode = (TextView) findViewById(R.id.tv_decode);
initListener();
}
private void initListener() {
encryption.setOnClickListener(this);
decode.setOnClickListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()) {
case R.id.btn_encryption://加密
String encryptionString = encryptionContext.getText().toString().trim();
if (TextUtils.isEmpty(encryptionString)) {
Toast.makeText(mContext, “请输入加密内容”, Toast.LENGTH_SHORT).show();
return;
}
try {
String hexKey = new AlgorithmUtil().getAESKey();
// 注意,这里的encoded是不能强转成string类型字符串的
encoded = AlgorithmUtil.getAESEncode(hexKey, encryptionString);
tvEncryption.setText(hexKey);
} catch (Exception e) {
e.printStackTrace();
}
break;
case R.id.btn_decode://解密
String decodeString = tvEncryption.getText().toString().trim();
if (TextUtils.isEmpty(decodeString)) {
Toast.makeText(mContext, “请先加密”, Toast.LENGTH_SHORT).show();
return;
}
try {
byte[] decoded = AlgorithmUtil.getAESDecode(decodeString, encoded);
tvDecode.setText(new String(decoded));
} catch (Exception e) {
e.printStackTrace();
}
break;
}
}
}
AlgorithmUtil
package tsou.com.encryption.aes128;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class AlgorithmUtil {
public final static String ENCODING = “UTF-8”;
/**
• 将二进制转换成16进制
•
• @param buf
• @return
*/
public static String parseByte2HexStr(byte buf[]) {
StringBuffer sb = new StringBuffer();
for (int i = 0; i < buf.length; i++) {
String hex = Integer.toHexString(buf[i] & 0xFF);
if (hex.length() == 1) {
hex = ‘0’ + hex;
}
sb.append(hex.toUpperCase());
}
return sb.toString();
}
/**
• 将16进制转换为二进制
•
• @param hexStr
• @return
*/
public static byte[] parseHexStr2Byte(String hexStr) {
if (hexStr.length() < 1)
return null;
byte[] result = new byte[hexStr.length() / 2];
for (int i = 0; i < hexStr.length() / 2; i++) {
int high = Integer.parseInt(hexStr.substring(i * 2, i * 2 + 1), 16);
int low = Integer.parseInt(hexStr.substring(i * 2 + 1, i * 2 + 2), 16);
result[i] = (byte) (high * 16 + low);
}
return result;
}
/**
• 生成密钥
• 自动生成base64 编码后的AES128位密钥
•
• @throws //NoSuchAlgorithmException
• @throws //UnsupportedEncodingException
*/
public static String getAESKey() throws Exception {
KeyGenerator kg = KeyGenerator.getInstance(“AES”);
kg.init(128);//要生成多少位,只需要修改这里即可128, 192或256
SecretKey sk = kg.generateKey();
byte[] b = sk.getEncoded();
return parseByte2HexStr(b);
aeskey秘钥生成 aes密钥生成过程
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
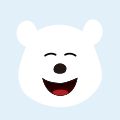
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
aes 加密 秘钥在线生成 aes加密秘钥长度
今天给大家推荐一篇文章,来自我的好朋友老程。深耕嵌入式,技术扎实,他用数学的方式讲解嵌入式系统的开发,简单明了,很容易理解,同时给我们开发带来很好的理论指导思路。需要与外界进行数据交互传输的电子产品,为保证数据安全,一般会对明文进行加密处理。总的来说就是将真正需要传输的内容转换成无法理解的数据,接收方通过预先定义的方式还原,防止第三方截取篡改。比如欧盟对数据隐私有严格的条例GDPR标准。加密算法很
aes 加密 秘钥在线生成 aes key长度 aes 加密长度 aes256 加密后的长度 aes加密后的数据长度