CLLocationManager
CLLocationManager使用步骤
1.创建一个CLLocationManager实例
CLLocationManager *locationManager = [[CLLocationManager alloc]init];
2.设置CLLocationManager实例委托和精度
locationManager.delegate = self;
locationManager.desiredAccuracy = kCLLocationAccuracyBest;
3.设置距离筛选器distanceFilter,下面表示设备至少移动1000米,才通知delegate
locationManager.distanceFilter = 1000.0f;
或者没有筛选器的默认设置:
locationManager.distanceFilter = kCLDistanceFilterNone;
4.启动请求
[locationManager startUpdatingLocation];
5.停止请求
[locationManager stopUpdatingLocation];
罗盘功能
1.通过CLLocationManagerDelegat
e的-(void)locationManager:(CLLocationManager *)manager
didUpdateHeading:(CLHeading *)newHeading获取当前用户手机与地磁北极或者地理北极的夹角。
夹角范围[0, 360)
0-north 90-east 180-south
2.将罗盘上的指南针旋转一定角度
CGFloat heading =(-1.0f*M_PI*newHeading.trueHeading)/180.f;
self.circleView.transform =CGAffineTransformMakeRot
ation(heading);
注意事项:
1.CLLocationManager用于获取当前位置,定位有相当大的误差。如果app不需要实时的获取位置信息且
使用了MKMapView, 则使用MKMapView获取当前位置,这个位置比较准确
2.desiredAccuracy适合需求就可以了,并不是都需要最佳的精度。
3.当不需要更新位置信息的时候,一定要停止。
MKReverseGeocoder查询地理位置信息,包括国家、城市、区域等等
1.实例MKReverseGeocoder
MKReverseGeocoder *geocoder =[[MKReverseGeocoder alloc] initWithCoordinate:coordinate];
geocoder.delegate =self;
[geocoder start];
2.在MKReverseGeocoderDelegat
e方法中获取placemark信息
- (void)reverseGeocoder:(MKReverseGeocoder *)geocoderdidFindPlacemark:(MKPlacemark *)placemark 成功查询出了地理位置信息
- (void)reverseGeocoder:(MKReverseGeocoder *)geocoderdidFailWithError:(NSError *)error 获取失败
注意事项:
不需要的时候一定要调用cancel方法,停止查询
MKMapView MapKit地图控件
可以使用在map上显示圆. 点, 大头针,画路径等。
1.MKMapViewDelegate的常用回掉方法
2.mapview缩小的时候,有时后不会回掉regionDidChangeAnimated这个方法,可以通过添加gesturerecognizer检测这个时间。
但必须实现UIGestureRecognizerDeleg
ate的
- (BOOL)gestureRecognizer:(UIGestureRecognizer *)gestureRecognizershouldRecognizeSimultane
ouslyWithGestureRecogniz
er:(UIGestureRecognizer*)otherGestureRecognizer方法
以添加大头针为例
1.创建一个自定义的MKPinAnnotation类,必须遵守MKAnnotation协议
2.在mapview上添加一个MKPinAnnotation是实例
MKPintAnnotation*pinAnnotation = [[MKPintAnnotation alloc]initWithCoordinate:_currentCoordinate];
pinAnnotation.title =@"PIN-TITLE";
pinAnnotation.subtitle =@"PIN_SUBTITLE";
[_mapViewaddAnnotation:pinAnnotation];
3.实现- (MKAnnotationView *)mapView:(MKMapView *)mapViewviewForAnnotation:(id<MKAnnotation>)annotation {
MKPinAnnotationView *pinView = [[[MKPinAnnotationView alloc]initWithAnnotation:annotation reuseIdentifier:pinIdentifier]autorelease];
pinView.animatesDrop = TRUE;
pinView.pinColor =MKPinAnnotationColorRed;//MKPinAnnotationColorGree
n;
pinView.canShowCallout = TRUE;
UIButton*button = [UIButton buttonWithType:UIButtonTypeDetailDisclo
sure];
button.frame = CGRectMake(0, 0, 25, 25);
pinView.rightCalloutAccessoryVie
w = button;
}
注意事项:
实际使用的时候在添加overlay和annotation的时候需要先移除mapview上已经有的overlays和annotations,避免叠加在一起了。
使用Google API在mapview上画路径
在mapview画指定两点之间的路径的原理和在地图上添加一个overlayview是相同的,关键是获取路径的数据.
一般路径数据是经纬度的数据,需要转化成mapview上的的mkmappoint数据类型的。
1.调用GoogleAPI获取两点之间的路径数据,详见http://code.google.com/apis/maps/documentation/directions/
2.解析路径数据(json格式),将经纬度的点转化为mkmapoint
3.在mapview上加个polygon line overlay
MKPolyline *routeLine = [MKPolylinepolylineWithPoints:points count:numberOfPoints;
[_mapViewaddOverlay:routeLine];
[_mapViewsetVisibleMapRect:routeVisableRegion animated:YES];
4.实现
- (MKOverlayView *)mapView:(MKMapView *)mapView viewForOverlay:(id<MKOverlay>)overlay {
MKPolygonView*overlayPolygonView = nil;
if ([overlayisKindOfClass:[MKPolyline class]]) {
CGFloat lineWidth = 6;
UIColor *fillColor = [UIColor redColor];
UIColor *strokeColor = [UIColorgreenColor];
overlayPolygonView = [[[MKPolylineView alloc]initWithPolyline:overlay] autorelease];
overlayPolygonView.fillColor = fillColor;
overlayPolygonView.strokeColor =strokeColor;
overlayPolygonView.lineWidth = lineWidth;
}
returnoverlayPolygonView;
}
iOS的定位经纬度坐标系转换 iphone经纬度
转载文章标签 iOS的定位经纬度坐标系转换 mkmapview 地图 CoreLocation 数据 文章分类 iOS 移动开发
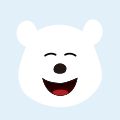
-
python抓取经纬度信息
爬取城市经纬度信息
ci 结果集 返回结果