package org.springframework.core.env;
/**
• Interface representing the environment in which the current application is running.
• Models two key aspects of the application environment: profiles and
• properties. Methods related to property access are exposed via the
• {@link PropertyResolver} superinterface.
•
• A profile is a named, logical group of bean definitions to be registered
• with the container only if the given profile is active. Beans may be assigned
• to a profile whether defined in XML or via annotations; see the spring-beans 3.1 schema
• or the {@link org.springframework.context.annotation.Profile @Profile} annotation for
• syntax details. The role of the {@code Environment} object with relation to profiles is
• in determining which profiles (if any) are currently {@linkplain #getActiveProfiles
• active}, and which profiles (if any) should be {@linkplain #getDefaultProfiles active
• by default}.
•
• Properties play an important role in almost all applications, and may
• originate from a variety of sources: properties files, JVM system properties, system
• environment variables, JNDI, servlet context parameters, ad-hoc Properties objects,
• Maps, and so on. The role of the environment object with relation to properties is to
• provide the user with a convenient service interface for configuring property sources
• and resolving properties from them.
•
• Beans managed within an {@code ApplicationContext} may register to be {@link
• org.springframework.context.EnvironmentAware EnvironmentAware} or {@code @Inject} the
• {@code Environment} in order to query profile state or resolve properties directly.
•
• In most cases, however, application-level beans should not need to interact with the
• {@code Environment} directly but instead may have to have {@code ${…}} property
• values replaced by a property placeholder configurer such as
• {@link org.springframework.context.support.PropertySourcesPlaceholderConfigurer
• PropertySourcesPlaceholderConfigurer}, which itself is {@code EnvironmentAware} and
• as of Spring 3.1 is registered by default when using
• {@code context:property-placeholder/}.
•
• Configuration of the environment object must be done through the
• {@code ConfigurableEnvironment} interface, returned from all
• {@code AbstractApplicationContext} subclass {@code getEnvironment()} methods. See
• {@link ConfigurableEnvironment} Javadoc for usage examples demonstrating manipulation
• of property sources prior to application context {@code refresh()}.
•
• @author Chris Beams
• @since 3.1
• @see PropertyResolver
• @see EnvironmentCapable
• @see ConfigurableEnvironment
• @see AbstractEnvironment
• @see StandardEnvironment
• @see org.springframework.context.EnvironmentAware
• @see org.springframework.context.ConfigurableApplicationContext#getEnvironment
• @see org.springframework.context.ConfigurableApplicationContext#setEnvironment
• @see org.springframework.context.support.AbstractApplicationContext#createEnvironment
*/
public interface Environment extends PropertyResolver {
/**
• Return the set of profiles explicitly made active for this environment. Profiles
• are used for creating logical groupings of bean definitions to be registered
• conditionally, for example based on deployment environment. Profiles can be
• activated by setting {@linkplain AbstractEnvironment#ACTIVE_PROFILES_PROPERTY_NAME
• “spring.profiles.active”} as a system property or by calling
• {@link ConfigurableEnvironment#setActiveProfiles(String…)}.
• If no profiles have explicitly been specified as active, then any {@linkplain
• #getDefaultProfiles() default profiles} will automatically be activated.
• @see #getDefaultProfiles
• @see ConfigurableEnvironment#setActiveProfiles
• @see AbstractEnvironment#ACTIVE_PROFILES_PROPERTY_NAME
*/
String[] getActiveProfiles();
/**
• Return the set of profiles to be active by default when no active profiles have
• been set explicitly.
• @see #getActiveProfiles
• @see ConfigurableEnvironment#setDefaultProfiles
• @see AbstractEnvironment#DEFAULT_PROFILES_PROPERTY_NAME
*/
String[] getDefaultProfiles();
/**
• Return whether one or more of the given profiles is active or, in the case of no
• explicit active profiles, whether one or more of the given profiles is included in
• the set of default profiles. If a profile begins with ‘!’ the logic is inverted,
• i.e. the method will return true if the given profile is not active.
• For example, env.acceptsProfiles(“p1”, “!p2”) will
• return {@code true} if profile ‘p1’ is active or ‘p2’ is not active.
• @throws IllegalArgumentException if called with zero arguments
• or if any profile is {@code null}, empty or whitespace-only
• @see #getActiveProfiles
• @see #getDefaultProfiles
*/
boolean acceptsProfiles(String… profiles);
}
鉴于注释太多,抽出其中核心代码:
package org.springframework.core.env;
public interface Environment extends PropertyResolver {
String[] getActiveProfiles();
String[] getDefaultProfiles();
boolean acceptsProfiles(String… profiles);
}
可以看到Environment 又extends PropertyResolver
/*
• Copyright 2002-2013 the original author or authors.
•
• Licensed under the Apache License, Version 2.0 (the “License”);
• you may not use this file except in compliance with the License.
• You may obtain a copy of the License at
•
http://www.apache.org/licenses/LICENSE-2.0•
• Unless required by applicable law or agreed to in writing, software
• distributed under the License is distributed on an “AS IS” BASIS,
• WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
• See the License for the specific language governing permissions and
• limitations under the License.
*/
package org.springframework.core.env;
/**
• Interface for resolving properties against any underlying source.
•
• @author Chris Beams
• @since 3.1
• @see Environment
• @see PropertySourcesPropertyResolver
*/
public interface PropertyResolver {
/**
• Return whether the given property key is available for resolution, i.e.,
• the value for the given key is not {@code null}.
*/
boolean containsProperty(String key);
/**
• Return the property value associated with the given key, or {@code null}
• if the key cannot be resolved.
• @param key the property name to resolve
• @see #getProperty(String, String)
• @see #getProperty(String, Class)
• @see #getRequiredProperty(String)
*/
String getProperty(String key);
/**
• Return the property value associated with the given key, or
• {@code defaultValue} if the key cannot be resolved.
• @param key the property name to resolve
• @param defaultValue the default value to return if no value is found
• @see #getRequiredProperty(String)
• @see #getProperty(String, Class)
*/
String getProperty(String key, String defaultValue);
/**
• Return the property value associated with the given key, or {@code null}
• if the key cannot be resolved.
• @param key the property name to resolve
• @param targetType the expected type of the property value
• @see #getRequiredProperty(String, Class)
*/
T getProperty(String key, Class targetType);
/**
• Return the property value associated with the given key, or
• {@code defaultValue} if the key cannot be resolved.
• @param key the property name to resolve
• @param targetType the expected type of the property value
• @param defaultValue the default value to return if no value is found
• @see #getRequiredProperty(String, Class)
*/
T getProperty(String key, Class targetType, T defaultValue);
/**
• Convert the property value associated with the given key to a {@code Class}
• of type {@code T} or {@code null} if the key cannot be resolved.
• @throws org.springframework.core.convert.ConversionException if class specified
• by property value cannot be found or loaded or if targetType is not assignable
• from class specified by property value
• @see #getProperty(String, Class)
*/
Class getPropertyAsClass(String key, Class targetType);
/**
• Return the property value associated with the given key (never {@code null}).
• @throws IllegalStateException if the key cannot be resolved
springboot获取系统环境
转载文章标签 springboot获取系统环境 spring boot 数据库 后端 spring 文章分类 架构 后端开发
上一篇:centos 复制文件夹到
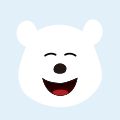
-
php获取外部环境变量的几种方式
php环境变量的使用
环境变量 php windows操作系统 -
Springboot yml获取系统环境变量的值
注意,这里说的是获取系统环境变量的值,譬如Windows里配置的JAVA_HOME之类的,可
yml配置环境变量 yml读取环境变量 Springboot读取环境变量 环境变量 maven -
【搭建环境】springboot医院管理系统
【搭建环境】springboot医院管理系统
intellij-idea java spring boot 数据库 ide