• 字节流读数据
• public class FileInputStreamDemo02 {
• public static void main(String[] args) throws IOException {
• //创建字节输入流对象
• FileInputStream fis = new FileInputStream("myByteStream\\fos.txt");
• byte[] bys = new byte[1024]; //1024及其整数倍
• int len;
• //循环读取
• while ((len=fis.read(bys))!=-1) {
• System.out.print(new String(bys,0,len));
• }
• //释放资源
• fis.close();
•
• 字节流写数据
• import java.io.*;
• public class WriteToFile {
• public static void main(String[] args) {
• try {
• // 创建一个 FileOutputStream 对象来写入数据
• FileOutputStream outputStream = new FileOutputStream("output.txt");
• // 要写入的数据
• String data = "Hello, World!";
• // 将字符串转换为字节数组
• byte[] bytes = data.getBytes();
• // 写入数据到文件中
• outputStream.write(bytes);
• // 关闭输出流
• outputStream.close();
• }
• catch (IOException e) {
• e.printStackTrace(); } } }
•
• 字节流复制文件
• :复制文本文件,其实就把文本文件的内容从一个文件中读取出来(数据源),然后写入到另一个文件中(目的地)
• 根据数据源创建字节输入流对象
• 根据目的地创建字节输出流对象
• 读写数据,复制图片(一次读取一个字节数组,一次写入一个字节数组)
• 释放资源
•
• public class CopyJpgDemo {
• public static void main(String[] args) throws IOException {
• //根据数据源创建字节输入流对象
• FileInputStream fis = new FileInputStream("E:\\develop\\a.jpg");
• //根据目的地创建字节输出流对象
• FileOutputStream fos = new FileOutputStream("myByteStream\\a.jpg");
• //读写数据,复制图片(一次读取一个字节数组,一次写入一个字节数组)
• byte[] bys = new byte[1024];
• int len;
• while ((len=fis.read(bys))!=-1) {
• fos.write(bys,0,len);
• }
• //释放资源
• fos.close();
• fis.close();
• }
•
• 字节缓冲流
• public class BufferStreamDemo {
• public static void main(String[] args) throws IOException {
• //字节缓冲输出流:BufferedOutputStream(OutputStream out)
• BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("myByteStream\\bos.txt"));
• //写数据
• bos.write("hello\r\n".getBytes());
• bos.write("world\r\n".getBytes());
• //释放资源
• bos.close();
• //字节缓冲输入流:BufferedInputStream(InputStream in)
• BufferedInputStream bis = new BufferedInputStream(new FileInputStream("myByteStream\\bos.txt"));
• //一次读取一个字节数据
• // int by;
• // while ((by=bis.read())!=-1) {
• // System.out.print((char)by);
• // }
• //一次读取一个字节数组数据
• byte[] bys = new byte[1024];
• int len;
• while ((len=bis.read(bys))!=-1) {
• System.out.print(new String(bys,0,len));
• }
• //释放资源
• bis.close();
• }
•
• 字符流
• Writer: 用于写入字符流的抽象父类
• FileWriter: 用于写入字符流的常用子类
• 构造方法
• public class InputStreamReaderDemo {
• public static void main(String[] args) throws IOException {
• FileReader fr = new FileReader("myCharStream\\b.txt");
• //int read():一次读一个字符数据
• // int ch;
• // while ((ch=fr.read())!=-1) {
• // System.out.print((char)ch);
• // }
• //int read(char[] cbuf):一次读一个字符数组数据
• char[] chs = new char[1024];
• int len;
• while ((len = fr.read(chs)) != -1) {
• System.out.print(new String(chs, 0, len));
• }
• //释放资源
• fr.close();
• }
•
• 字符缓冲流
•
• public class BufferedStreamDemo01 {
• public static void main(String[] args) throws IOException {
• //BufferedWriter(Writer out)
• BufferedWriter bw = new BufferedWriter(new FileWriter("myCharStream\\bw.txt"));
• bw.write("hello\r\n");
• bw.write("world\r\n");
• bw.close();
•
• //BufferedReader(Reader in)
• BufferedReader br = new BufferedReader(new FileReader("myCharStream\\bw.txt"));
• //一次读取一个字符数据
• // int ch;
• // while ((ch=br.read())!=-1) {
• // System.out.print((char)ch);
• // }
• //一次读取一个字符数组数据
• char[] chs = new char[1024];
• int len;
• while ((len=br.read(chs))!=-1) {
• System.out.print(new String(chs,0,len));
• }
• br.close();
•
• }
• }
•
• 字符缓冲流操作文件中数据排序案例:使用字符缓冲流读取文件中的数据,排序后再次写到本地文件
• 将文件中的数据读取到程序中
• 对读取到的数据进行处理
• 将处理后的数据添加到集合中
• 对集合中的数据进行排序
• 将排序后的集合中的数据写入到文件中
•
• public class CharStreamDemo14 {
• public static void main(String[] args) throws IOException {
• //需求:读取文件中的数据,排序后再次写到本地文件
• //分析:
• //1.要把文件中的数据读取进来。
• BufferedReader br = new BufferedReader(new FileReader("charstream\\sort.txt"));
• //输出流一定不能写在这里,因为会清空文件中的内容
• //BufferedWriter bw = new BufferedWriter(new FileWriter("charstream\\sort.txt"));
• String line = br.readLine();
• System.out.println("读取到的数据为" + line);
• br.close();
• //2.按照空格进行切割
• String[] split = line.split(" ");//9 1 2 5 3 10 4 6 7 8
• //3.把字符串类型的数组变成int类型
• int [] arr = new int[split.length];
• //遍历split数组,可以进行类型转换。
• for (int i = 0; i < split.length; i++) {
• String smallStr = split[i];
• //类型转换
• int number = Integer.parseInt(smallStr);
• //把转换后的结果存入到arr中
• arr[i] = number;
• }
• //4.排序
• Arrays.sort(arr);
• System.out.println(Arrays.toString(arr));
• //5.把排序之后结果写回到本地 1 2 3 4...
• BufferedWriter bw = new BufferedWriter(new FileWriter("charstream\\sort.txt"));
• //写出
• for (int i = 0; i < arr.length; i++) {
• bw.write(arr[i] + " ");
• bw.flush();
• }
• //释放资源
• bw.close();
• }
• 转换流
• 它读取字节,并使用指定的编码将其解码为字符,它使用的字符集可以由名称指定,也可以被明确指定,或者可以接受平台的默认字符
• InputStreamReader(InputStream in,String chatset) 使用指定的字符编码创建InputStreamReader对象
• OutputStreamWriter(OutputStream out) 使用默认字符编码创建OutputStreamWriter对象
•
• 对象操作流
• 对象序列化:就是将对象保存到磁盘中,或者在网络中传输对象使用一个字节序列表示一个对象,该字节序列包含:对象的类型、对象的数据和对象中存储的属性等信息字节序列写到文件之后,相当于文件中持久保存了一个对象的信息
• 对象序列化流: ObjectOutputStream
• 该字节序列还可以从文件中读取回来,重构对象,对它进行反序列化
• 序列化对象的方法
• void writeObject(Object obj) 将指定的对象写入ObjectOutputStream(写)
•
• public class ObjectOutputStreamDemo {
• public static void main(String[] args) throws IOException {
• //ObjectOutputStream(OutputStream out):创建一个写入指定的OutputStream的ObjectOutputStream
• ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("myOtherStream\\oos.txt"));
• //创建对象
• Student s = new Student("佟丽娅",30);
• //void writeObject(Object obj):将指定的对象写入ObjectOutputStream
• oos.writeObject(s);
• //释放资源
• oos.close();
• }
• }
•
• 对象反序列化流(读)
• public class ObjectInputStreamDemo {
• public static void main(String[] args) throws IOException, ClassNotFoundException {
• //ObjectInputStream(InputStream in):创建从指定的InputStream读取的ObjectInputStream
• ObjectInputStream ois = new ObjectInputStream(new FileInputStream("myOtherStream\\oos.txt"));
• //Object readObject():从ObjectInputStream读取一个对象
• Object obj = ois.readObject();
• Student s = (Student) obj;
• System.out.println(s.getName() + "," + s.getAge());
• ois.close();
• }
•
•
• 创建多线程程序,
• // 定义一个新的Thread子类
• class MyThread extends Thread {
• // 重写run方法,该方法会在线程启动后自动执行
• @Override public void run() {
• for (int i = 0; i < 10; i++) {
• System.out.println(Thread.currentThread().getId() + " Value " + i);
• try {
• Thread.sleep(1000); // 让线程睡眠一段时间,模拟耗时操作
• }
• catch (InterruptedException e) {
• e.printStackTrace();
• }
• }
• }
• }
• 类实现 Runnable 接口
• public class Main { public static void main(String[] args) {
• // 创建MyThread对象
• MyThread t1 = new MyThread();
• MyThread t2 = new MyThread();
• // 启动线程
• t1.start();
• t2.start(); } }
• public class MyRunnable implements Runnable {
• @Override public void run() {
• System.out.println("Running in the thread: " + Thread.currentThread().getId());
• }
• public static void main(String[] args) {
• MyRunnable myRunnable = new MyRunnable();
• Thread thread = new Thread(myRunnable);
• thread.start(); } }
•
• 爬山问题:
• package cn.kgc.pojo;
• public class ClimbShan extends Thread{
• private int time; private int num; public void setTime(int time){
• this.time =time; }
• public int getTime() {
• return time; }
• public int getNum() {
• return num; }
• public void setNum(int num) {
• this.num = num; }
• public ClimbShan(){
• }
• public ClimbShan(String name,int time,int num){
• super(name);
• this.time =time;
• this.num = num;}
• @Override public void run() {
• for (int i=0;i<10;i++){
• System.out.println(Thread.currentThread().getName()+"爬完了一百米");
• try {
• Thread.sleep(time);
• if(i==9){
• System.out.println(Thread.currentThread().getName()+"到达终点"); }
• } catch (InterruptedException e) {
• throw new RuntimeException(e); }
• }
• }
• }
• 测试类
• package cn.kgc.dao;import cn.kgc.pojo.ClimbShan;
• public class Climbdao {
• public static void main(String[] args) {
• ClimbShan cs = new ClimbShan("老年人",50,10);
• ClimbShan c1 = new ClimbShan("年轻人",30,10);
• Thread thread = new Thread(cs);
• Thread thread1 = new Thread(c1);
• cs.start();
• c1.start(); }
• }
• 抢票问题
• package cn.kgc.pojo;
• public class BuyTicket {
• private static int num =0;
• public static int count = 15;
• public synchronized void book1(String name){
• if(count>0){
• if(name.equals("黄牛")&&num==0){
• System.out.println(name+"抢到了第:"+count+"张票");
• count--;
• num++; }
• else if (!name.equals("黄牛")){
• if (count>0){
• System.out.println(name+"抢到了第:"+count+"张票");
• count--; }
• else {
• System.out.println(name+"的票已经被抢完了");
• }
• }
• else {
• System.out.println("票已经卖完了!"); }
• }
• }
• }
• 测试类
• package cn.kgc.test;import cn.kgc.pojo.BuyTicket;public class TestBuyTicket {
• public static void main(String[] args) {
• BuyTicket buyTicket = new BuyTicket(); Thread thread = new Thread();
• for(int i = 0; i <5; i++) {
• buyTicket.book1("桃跑跑"); }
• Thread thread1 = new Thread();
• for(int i = 0; i < 5; i++) {
• buyTicket.book1("张票票"); }
• Thread thread2 = new Thread();
• for(int i = 0; i < 5; i++) {
• buyTicket.book1("黄牛党"); }
• thread.start();
• thread1.start();
• thread2.start(); }
• }
•
java ftl动态模板
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:hadoop的9866端口作用
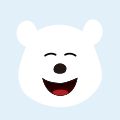
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章