1。写死数据的蠢办法
/
// 一个显示姓名,性别和出生年月的表格,点击表格某行的时候会有两种事件发生 //
/ package com.yinbodotcc.JTable;
/*
A网友说: repain()调用Component类的update()方法清除Component背景中以前的绘图,
然后update()在调用paint()。一般不要重载repaint(),有必要的话重载update()。B网友说: repaint() -> update() -> paint()
update() 一般都是有需要才被调用的。
通常应该重载 update() 和 paint() 而不是 repaint()。我说: 我就喜欢看大家说,然后记录下来,哈哈
*/
// 这里需要学会JTable事件处理, 以及为了线程安全,需要从事件分派线程出发来显示界面import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import java.awt.Dimension;
import java.awt.GridLayout;import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;import javax.swing.ListSelectionModel;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;public class SimpleTableDemo extends JPanel {
private boolean DEBUG = false;
private boolean ALLOW_ROW_SELECTION = true;
//个人感觉列可以选择,用处不是很大,掌握行可以选择,及单独的单元可以选中比较重要
private boolean ALLOW_COLUMN_SELECTION = false; public SimpleTableDemo() {
super(new GridLayout(1,0)); //表格中数据填充
String[] columnNames = {"姓名","性别","出生年月","婚否"};
Object[][] data = {
{"杨安印", "男",
new Integer(19880206), new Boolean(false)},
{"吴义田", "男",
new Integer(19890206), new Boolean(true)}
};
final JTable table = new JTable(data, columnNames);
table.setPreferredScrollableViewportSize(new Dimension(500, 70));
///
///
//点击表格体的事件
if (DEBUG) {
table.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
printDebugData(table);
}
});
}
table.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
//点击表格某行的事件
if(ALLOW_ROW_SELECTION){
ListSelectionModel lsm=table.getSelectionModel();
lsm.addListSelectionListener(new ListSelectionListener(){
public void valueChanged(ListSelectionEvent e)
{
//这一句若没有,则点击另外一行时候,将 "...被选中"打印两次
if (e.getValueIsAdjusting()) return; ListSelectionModel ls=(ListSelectionModel)e.getSource();
if(ls.isSelectionEmpty())
{
System.out.println("什么也没有选中");
}
else
{
int index=ls.getMinSelectionIndex();
System.out.println("行: "+index+" 被选中");
}
}
}); }
else table.setRowSelectionAllowed(false);
//点击表格某列的事件
if(ALLOW_COLUMN_SELECTION)
{
if (ALLOW_ROW_SELECTION)
{
//可以选择单独的单元
table.setCellSelectionEnabled(true);
}
table.setColumnSelectionAllowed(true);
ListSelectionModel colSM =table.getColumnModel().getSelectionModel();
colSM.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent e) {
if (e.getValueIsAdjusting()) return; ListSelectionModel lsm = (ListSelectionModel)e.getSource();
if (lsm.isSelectionEmpty()) {
System.out.println("没有列被选中");
} else {
int selectedCol = lsm.getMinSelectionIndex();
System.out.println("列 " + selectedCol
+ " 被选中");
}
}
}); }
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
} private void printDebugData(JTable table) {
int numRows = table.getRowCount();
int numCols = table.getColumnCount();
javax.swing.table.TableModel model = table.getModel(); System.out.println("Value of data: ");
for (int i=0; i < numRows; i++) {
System.out.print(" row " + i + ":");
for (int j=0; j < numCols; j++) {
System.out.print(" " + model.getValueAt(i, j));
}
System.out.println();
}
System.out.println("--------------------------");
} /**
* Create the GUI and show it. For thread safety,
* this method should be invoked from the
* event-dispatching thread.
*/
private static void createAndShowGUI() {
JFrame frame = new JFrame("没有用DataModel的JTable例子");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
SimpleTableDemo newContentPane = new SimpleTableDemo();
newContentPane.setOpaque(true); //content panes must be opaque
frame.setContentPane(newContentPane);
//调整此窗口的大小,以适合其子组件的首选大小和布局
frame.pack();
frame.setVisible(true);
} public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
缺点评价:
A。所有行都能编辑,不一定好。
B。要求是数组数据,但是如果数据是从数据库中获取,则不方便。
C。所有数据都被作为String类型,非常不方便。
所以我们需要更大的方便,请望下面看,我们会有进步的
2。 让表格某一行不被选中(网友提供的做法)
///class MyLSL implements ListSelectionListener {
private final int notSelectableRow;
public MyLSL( int notSelectableRow ) {
this.notSelectableRow = notSelectableRow;
}
public void valueChanged( ListSelectionEvent e ) {
if ( ! e.getValueIsAdjusting( ) && t.isRowSelected( notSelectableRow ) )
t.removeRowSelectionInterval( notSelectableRow, notSelectableRow );
}
}
然后就可以:
t.getSelectionModel( ).addListSelectionListener( new MyLSL( 1 ) );
这里t是一个
3.
///
自己定义数据模型,然后传给视图
import javax.swing.JFrame;
import javax.swing.table.AbstractTableModel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import java.awt.Dimension;
import java.awt.GridLayout;import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;import javax.swing.ListSelectionModel;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
看模型类:
class MyTableModel extends AbstractTableModel
{
//表格中数据填充
String[] columnNames = {"姓名","性别","出生年月","婚否"};
Object[][] data = {
{"杨安印", "男",
new Integer(19880206), new Boolean(false)},
{"吴义田", "男",
new Integer(19890206), new Boolean(true)}
};
public int getRowCount()
{
return data.length;
}
public int getColumnCount()
{
return columnNames.length;
}
public Object getValueAt(int row, int column)
{
return data[row][column];
}
public Class<?> getColumnClass(int columnIndex)
{
return getValueAt(0, columnIndex).getClass();
}
public String getColumnName(int column)
{
return columnNames[column];
}
public void setValueAt(Object value, int row, int col) {//其实这个就包含了一定得事件处理性质
if (SimpleTableDemo.DEBUG) {
System.out.println("设置第 " + row + "行,第" + col
+ "列得值为 " + value
+ " 它得类是 "
+ value.getClass());
} data[row][col] = value;
fireTableCellUpdated(row, col);//还需要更新 if (SimpleTableDemo.DEBUG) {
System.out.println("新得数据是:");
printDebugData();
}
}
public boolean isCellEditable(int rowIndex,int columnIndex)
{
if(columnIndex<1)
return false;
else return true;
}
private void printDebugData() {
int numRows = getRowCount();
int numCols = getColumnCount(); for (int i=0; i < numRows; i++) {
System.out.print(" row " + i + ":");
for (int j=0; j < numCols; j++) {
System.out.print(" " + data[i][j]);
}
System.out.println();
}
System.out.println("--------------------------");
}
}
视图类:
public class SimpleTableDemo extends JPanel {
public static final boolean DEBUG = true;
private boolean ALLOW_ROW_SELECTION = true;
public SimpleTableDemo() {
super(new GridLayout(1,0));
MyTableModel m=new MyTableModel();
final JTable table = new JTable(m);
table.setPreferredScrollableViewportSize(new Dimension(500, 70));
///
//点击表格体的事件
if (DEBUG) {
table.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
printDebugData(table);
}
});
}
table.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
//点击表格某行的事件
if(ALLOW_ROW_SELECTION){
ListSelectionModel lsm=table.getSelectionModel();
lsm.addListSelectionListener(new ListSelectionListener(){
public void valueChanged(ListSelectionEvent e)
{
//这一句若没有,则点击另外一行时候,将 "...被选中"打印两次
if (e.getValueIsAdjusting()) return; ListSelectionModel ls=(ListSelectionModel)e.getSource();
if(ls.isSelectionEmpty())
{
System.out.println("什么也没有选中");
}
else
{
int index=ls.getMinSelectionIndex();
System.out.println("行: "+index+" 被选中");
}
}
}); }
else table.setRowSelectionAllowed(false);
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
} private void printDebugData(JTable table) {
int numRows = table.getRowCount();
int numCols = table.getColumnCount();
javax.swing.table.TableModel model = table.getModel(); System.out.println("Value of data: ");
for (int i=0; i < numRows; i++) {
System.out.print(" row " + i + ":");
for (int j=0; j < numCols; j++) {
System.out.print(" " + model.getValueAt(i, j));
}
System.out.println();
}
System.out.println("--------------------------");
} /**
* Create the GUI and show it. For thread safety,
* this method should be invoked from the
* event-dispatching thread.
*/
private static void createAndShowGUI() {
JFrame frame = new JFrame("没有用DataModel的JTable例子");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
SimpleTableDemo newContentPane = new SimpleTableDemo();
newContentPane.setOpaque(true); //content panes must be opaque
frame.setContentPane(newContentPane);
//调整此窗口的大小,以适合其子组件的首选大小和布局
frame.pack();
frame.setVisible(true);
} public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
java Jtable 点一下才刷新 jtable刷新表格
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
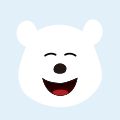
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
如何做到无感刷新Token?
如何做到无感刷新Token?
System 表单 json token -
swing jtable 表格
【代码】swing jtable 表格。
windows 服务器 运维 ci java