<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather Query</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
box-sizing: border-box;
background-color: #e9ecef;
}
.container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
background-color: white;
border-radius: 8px;
box-shadow: 0 2px 5px rgba(0,0,0,0.1);
}
h1 {
color: #333;
text-align: center;
}
input[type="text"] {
width: calc(100% - 22px);
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
margin-bottom: 10px;
}
button {
padding: 10px;
border: none;
border-radius: 5px;
background-color: #007bff;
color: white;
font-size: 1em;
cursor: pointer;
display: block;
margin: 0 auto;
}
button:hover {
background-color: #0056b3;
}
.weather-info {
margin-top: 20px;
}
.weather-info h2 {
margin: 0;
}
.weather-info p {
margin: 5px 0;
}
</style>
</head>
<body>
<div class="container">
<h1>Weather Query</h1>
<input type="text" id="cityInput" placeholder="Enter city name">
<button onclick="getWeather()">Get Weather</button>
<div id="weatherInfo" class="weather-info"></div>
</div>
<script>
function getWeather() {
const city = document.getElementById('cityInput').value;
if (city) {
fetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=YOUR_API_KEY`)
.then(response => response.json())
.then(data => {
const weatherInfo = document.getElementById('weatherInfo');
const temp = (data.main.temp - 273.15).toFixed(2); // Convert Kelvin to Celsius
weatherInfo.innerHTML = `
<h2>${data.name}</h2>
<p>Temperature: ${temp} °C</p>
<p>Weather: ${data.weather[0].description}</p>
`;
})
.catch(error => {
console.error('Error:', error);
});
} else {
alert('Please enter a city name.');
}
}
</script>
</body>
</html>
示例 15: 简单的天气查询页面
原创
©著作权归作者所有:来自51CTO博客作者避风塘主的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:1. 博客管理系统
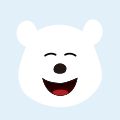
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
WPF 简单页面切换示例
【代码】WPF 简单页面切换示例。
wpf c# System Windows xml -
android 天气控件图片 android studio天气预报简单页面
Android简单版天气预报,显示天气预报(第二步)ListView的改进,因为每次在getView()方法中还是会调用View的findViewById()方法来获取一次控件的实例,写一个内部类,利用**view.setTag()**存储,getTag()取。其实实现的机制跟RecycleView差不多package com.example.xhhweather;import android
android 天气控件图片 Android天气预报 Android http访问网络 okhttp的使用 获得每日必应图片 -
jquery radio添加事件
/** * jQuery获取select的各种值 */ jQuery("#select_id").change(function(){ // 1.为Select添加事件,当选择其中一项时触发 //code... }); var checkValue = jQuery("#select_id").val(); //
jquery radio添加事件 javascript ViewUI jQuery d3