import requests
def get_code():
import requests
import json
headers = {
"Accept": "application/json, text/plain, */*", "Accept-Language": "zh-CN,zh;q=0.9", "Authorization": "", "Connection": "keep-alive", "Content-Type": "application/json;charset=UTF-8", "Origin": "https://disclosure.shcpe.com.cn", "Referer": "https://disclosure.shcpe.com.cn/", "Sec-Fetch-Dest": "empty", "Sec-Fetch-Mode": "cors", "Sec-Fetch-Site": "same-origin", "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36", "sec-ch-ua": "\"Not.A/Brand\";v=\"8\", \"Chromium\";v=\"114\", \"Google Chrome\";v=\"114\"", "sec-ch-ua-mobile": "?0", "sec-ch-ua-platform": "\"Windows\"" }
url = "https://disclosure.shcpe.com.cn/ent/ever-captcha/slider" data = {
"body": {
"uuid": "", "scenes": "哈尔滨宝钜房地产有限公司(91230109MA1BJ3CQX2)" }, "head": {
"transCode": "", "traceId": "" }
}
data = json.dumps(data, separators=(',', ':'))
response = requests.post(url, headers=headers, data=data)
responses = response.json()
datas = responses['data']
get_image(datas, 'bigImg')
get_image(datas, 'tarImg')
offset = get_offset()
def get_image(datas, img):
import base64
images = datas.get('{}'.format(img))
image = images.split(',')[1]
imgdata = base64.b64decode(image)
with open('{}.jpg'.format(img), 'wb') as f:
f.write(imgdata)
def get_offset():
import cv2
import numpy as np
bg = cv2.imread('./bigImg.jpg')
tg = cv2.imread('./tarImg.jpg')
result = cv2.matchTemplate(bg, tg, cv2.TM_CCOEFF_NORMED)
y, x = np.unravel_index(result.argmax(), result.shape)
print(x)
return x
if __name__ == "__main__":
get_offset()
滑块计算
原创
©著作权归作者所有:来自51CTO博客作者Θλιβερό.的原创作品,请联系作者获取转载授权,否则将追究法律责任
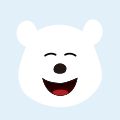
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章