“Java语言程序设计”期末复习题(续)(含答案)程序阅读部分
三.程序阅读题
1.阅读以下程序:
class A
{ public static void main(String[] args)
{String s,s1="";
char c;
s=args[0];
for (int i=0;i
{ c=s.charAt(i);
if(c>='a' && c<='z'){
s1=s1+Character.toUpperCase(c);
}else{ s1=s1+Character.toLowerCase(c); }
}
System.out.println(s1); }
}
若在dos命令行输入:java A hELLO,则输出为 Hello 。
2.写出以下程序的运行结果。
public class EqualOrNot
{ public static void main(String[] args) false
{ B b1=new B(5); false
B b2=new B(5);
System.out.println(b1==b2);
System.out.println(b1.equals(b2));
}
}
class B
{ int x;
B( int y){ x=y; }
}
3.阅读以下程序:
import java.io.*;
public class ReadLineTest{
public static void main(String[ ] args){
BufferedReader b=new BufferedReader (new InputStreamReader(System.in));
String s;
System.out.flush();
s=b.readLine();
System.out.println(s);
}
}
运行以上程序,若从键盘输入: Hello
则输出结果为 Hello 。
4.写出以下程序的功能。复制文件a.txt到文件b.txt
import java.io.*;
public class C {
public static void main(String[] args) throws IOException {
File inputFile = new File(“a.txt");
File outputFile = new File(“b.txt");
FileReader in = new FileReader(inputFile);
FileWriter out = new FileWriter(outputFile);
int c;
while ((c = in.read() ) != -1) out.write(c);
in.close();
out.close(); }
}
5.写出以下程序的功能。计算命令行参数串大小写字母的个数并在屏幕上显示结果
class Test
{ public static void main(String[] args)
{ String s;
char c;
int upper,lower;
upper=lower=0;
s=args[0];
for (int i=0;i
{ c=s.charAt(i);
if(c>='a' && c<='z') lower++;
if(c>='A' && c<='Z') upper++; }
System.out.println(upper+”,”+lower); }
}
6.以下程序段的输出结果为 1 2 3 4 5 8 9 。
public class C
{ public static void main(String args[ ]){
int i , j ;
int a[ ] = { 2,1,4,8,9,5,3};
for ( i = 0 ; i < a.length-1; i ++ ) {
int k = i;
for ( j = i ; j < a.length ; j++ )
if ( a[j]
int temp =a[i];
a[i] = a[k];
a[k] = temp; }
for ( i =0 ; i
System.out.print(a[i]+" ");
System.out.println( ); }
}
7.写出以下程序的运行结果。 no1 no2 no3
import java.util.*; no1 no3
public class Vec{
public static void main(String[] args) {
String[] s;
s=new String[2];
s[0]=new String("no1");
s[1]=new String("no2");
Vector v = new Vector();
for(int i = 0; i <2; i++) v.addElement(s[i]);
v.insertElementAt(new String("no3"),2);
Enumeration e = v.elements();
while(e.hasMoreElements()) System.out.print(e.nextElement()+" ");
System.out.println();
v.removeElement("no2" );
for(int i = 0; i < v.size() ; i++) System.out.print(v.elementAt(i) +" ");
System.out.println(); }
}
8.写出以下程序的运行结果。
class StaticTest {
static int x=1;
int y;
StaticTest()
{ y++; } x=2
public static void main(String args[ ]){ st.y=1
StaticTest st=new StaticTest(); st.y=1
System.out.println("x=" + x);
System.out.println("st.y=" + st.y);
st=new StaticTest();
System.out.println("st.y=" + st.y);
}
static { x++;}
}
9.写出以下程序的运行结果。 x=0
class StaticStuff x=5
{ x=2
static int x;
static { System.out.println("x=" + x); x+=5; }
public static void main(String args[ ]){
System.out.println("x=" + x);
}
static { System.out.println("x=" + x);x%=3; }
}
10.以下程序段的输出结果为 int , int 。
class Cruncher{
void crunch( int i ){
System.out.print(“int”); }
void crunch(String s){
System.out.print(“String”); }
public static void main(String args[ ]){
Cruncher crun=new Cruncher ( );
char ch=’h’;
int i=12;
crun.crunch(ch);
System.out.print(“,”);
crun.crunch(i); }
}
11.阅读以下程序,输出结果为 hellojava 。
import java.io.*;
public class TestString
{ public static void main(String args[ ])
{ StringC s = new StringC ("hello","java");
System.out.println(s); }
}
class StringC {
String s1;
String s2;
StringC( String str1 , String str2 )
{ s1 = str1; s2 = str2; }
public String toString( )
{ return s1+s2;}
}
12.阅读以下程序,写出输出结果。 in Second class
class First{ in Second class
public First(){
aMethod(); }
public void aMethod(){
System.out.println(“in First class”);}
}
public class Second extends First{
public Second(){
aMethod(); }
public void aMethod(){
System.out.println(“in Second class”);}
public static void main(String[ ] args){
new Second( ); }
}
13.写出以下程序的运行结果。 26
public class A
{
public static void main(String[ ] args)
{ System.out.println( test(15,26,4) ); }
static int test(int x, int y, int z)
{ return test( x, test(y,z) ); }
static int test(int x,int y)
{ if(x>y) return x;
else return y; }
}
14.写出以下程序的运行结果。 5.0
class MyException extends Exception{ Caught negative
public String toString( ){ return "negative"; }
}
public class ExceptionDemo{
public static void mySqrt(int a) throws MyException {
if( a<0 ) throw new MyException();
System.out.println(Math.sqrt(a));
}
public static void main( String args[] ){
try{ mySqrt(25 ); mySqrt(-5 ); }
catch( MyException e ){ System.out.println("Caught "+e); }
}
}
15.写出以下程序的运行结果。 s1==s2
class StringTest1
{
public static void main(String[] args)
{
String s1="hello";
String s2= "hello";
if(s1==s2){
System.out.println("s1==s2");
}else{
System.out.println("s1!=s2");}
}
}
16.写出以下程序的功能。从键盘输入一行字符,显示到屏幕上
import java.io.*;
public class ReadString{
public static void main(String[ ] args){
BufferedReader br=new BufferedReader (new InputStreamReader(System.in));
try{
System.out.println( br.readLine());
}catch(IOException e){}
}}
17.写出以下程序的运行结果。2 4 6 8
import java.io.*;
public class UseLabel
{ public static void main(String[] args)
{Loop:
for(int i=2; i<10; i++)
{ if( i%2!=0) continue Loop;
System.out.print(i+" "); }
}
}
18.写出以下程序的运行结果。 in First
class First { in Second
First() {
System.out.println ("in First"); }
}
public
class Second extends First {
Second() {
System.out.println("in Second"); }
public static void main(String[] args) {
Second mine= new Second(); }
}
19.写出以下程序的运行结果。 1
import java.io.*;
public class ATest{
public static void main(String args[]) {
Sub sb = new Sub( );
System.out.println(sb.method1( )); }
}
class Super{
int x=1 , y=2 ;
int method1(){ return x
}
class Sub extends Super{
int mothod1( ) { return ((x>y)?x:y); }
}
20.写出以下程序的功能。 将数组元素从大到小排序并显示到屏幕上
public class ABC
{
public static void main(String args[ ]){
int i , j ;
int a[ ] = { 9,7,5,1,3};
for ( i = 0 ; i < a.length-1; i ++ ) {
int k = i;
for ( j = i ; j < a.length ; j++ )
if ( a[j]>a[k] ) k = j;
int temp =a[i];
a[i] = a[k];
a[k] = temp; }
for ( i =0 ; i
System.out.print(a[i]+" ");
System.out.println( );
}
}
四.编程题
1.编写一个完整的Java Application 程序。包含接口ShapeArea,MyRectangle类,MyTriangle类及Test类,具体要求如下:
⑴接口ShapeArea:
double getArea():求一个形状的面积
double getPerimeter ():求一个形状的周长
⑵类 MyRectangle:
实现ShapeArea接口,并有以下属性和方法:
属性
width: double类型,表示矩形的长
height: double类型,表示矩形的高
方法
MyRectangle(double w, double h):构造函数
toString()方法 :输出矩形的描述信息,如“width=1.0,height=2.0, perimeter=6.0, area=2.0”
⑶类MyTriangle:
实现ShapeArea接口,并有以下属性和方法:
属性
x,y,z: double型,表示三角形的三条边
s: 周长的1/2(注:求三角形面积公式为,s=(x+y+z)/2 ,开方可用Math.sqrt(double)方法)
方法
MyTriangle(double x, double y, double z):构造函数,给三条边和s赋初值。
toString():输出矩形的描述信息,如“three sides:3.0,4.0,5.0,perimeter=12.0,area=6.0”
⑷Test类作为主类要完成测试功能
生成MyRectangle对象
② 调用对象的toString方法,输出对象的描述信息
2.编写一个完整的Java Application 程序。包含接口ShapeArea,类Circle、Rectangle、Test,具体要求如下:
⑴接口ShapeArea:
①接口方法
double getArea():求一个形状的面积
double getPerimeter ():求一个形状的周长
⑵类Circle:
实现ShapeArea接口,并有以下属性和方法:
属性
radius: double类型,表示圆的半径
方法
Circle(double r):构造函数
toString()方法 :输出圆的描述信息,如“radius=1.0, perimeter=6.28, area=3.14”
⑶Test类作为主类要完成测试功能
生成Circle对象
② 调用对象的toString方法,输出对象的描述信息
Java阅读程序题库 java程序阅读题及答案
转载文章标签 Java阅读程序题库 java语言程序设计期末考试试题及答案 System java Test 文章分类 Java 后端开发
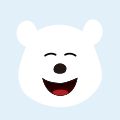
-
7.33阅读程序
函数
初始化 Max 局部变量 -
第九周练习 阅读程序
/* *Copyright(c) 2016,烟台大学计算机学院 *All rights reserved. *作 者:刘金石
C++ class 函数 析构函数 类的指针 -
第十六周 阅读程序
/* *Copyright(c) 2016,烟台大学计算机学院 *作 者:刘金石 *完成日期:2016年6月1
C++ ifstream ofstream ios 文本文件 -
python 程序练习批阅原理 python阅读程序题
一、选择题1.访问字符串中的部分字符的操作称为( A )。p89A.分片 B.合并 C.索引 D.赋值对分片的总结:大致就是用这样一个格式,
python 程序练习批阅原理 python 字符串 Python 正则表达式