1. <?php
2. /*** Mongodb类** examples:
3. * $mongo = new HMongodb("127.0.0.1:11223");
4. * $mongo->selectDb("test_db");
5. * 创建索引
6. * $mongo->ensureIndex("test_table", array("id"=>1), array('unique'=>true));
7. * 获取表的记录
8. * $mongo->count("test_table");
9. * 插入记录
10. * $mongo->insert("test_table", array("id"=>2, "title"=>"asdqw"));
11. * 更新记录
12. * $mongo->update("test_table", array("id"=>1),array("id"=>1,"title"=>"bbb"));
13. * 更新记录-存在时更新,不存在时添加-相当于set
14. * $mongo->update("test_table", array("id"=>1),array("id"=>1,"title"=>"bbb"),array("upsert"=>1));
15. * 查找记录
16. * $mongo->find("c", array("title"=>"asdqw"), array("start"=>2,"limit"=>2,"sort"=>array("id"=>1)))
17. * 查找一条记录
18. * $mongo->findOne("$mongo->findOne("ttt", array("id"=>1))", array("id"=>1));
19. * 删除记录
20. * $mongo->remove("ttt", array("title"=>"bbb"));
21. * 仅删除一条记录
22. * $mongo->remove("ttt", array("title"=>"bbb"), array("justOne"=>1));
23. * 获取Mongo操作的错误信息
24. * $mongo->getError();
25. */
26.
27. class
28.
29. //Mongodb连接
30. var $mongo;
31.
32. var $curr_db_name;
33. var $curr_table_name;
34. var $error;
35.
36. /**
37. * 构造函数
38. * 支持传入多个mongo_server(1.一个出问题时连接其它的server 2.自动将查询均匀分发到不同server)
39. *
40. * 参数:
41. * $mongo_server:数组或字符串-array("127.0.0.1:1111", "127.0.0.1:2222")-"127.0.0.1:1111"
42. * $connect:初始化mongo对象时是否连接,默认连接
43. * $auto_balance:是否自动做负载均衡,默认是
44. *
45. * 返回值:
46. * 成功:mongo object
47. * 失败:false
48. */
49. true, $auto_balance=true)
50. {
51. if
52. {
53. $mongo_server_num = count($mongo_server);
54. if ($mongo_server_num > 1
55. {
56. 1, $mongo_server_num);
57. $rand_keys = array_rand($mongo_server,$mongo_server_num);
58. 1];
59. foreach ($rand_keys as $key)
60. {
61. if ($key != $prior_server_num - 1)
62. {
63. ','
64. }
65. }
66. }
67. else
68. {
69. ',', $mongo_server);
70. } }
71. else
72. {
73. $mongo_server_str = $mongo_server;
74. }
75. try
76. this->mongo = new Mongo($mongo_server, array('connect'=>$connect));
77. }
78. catch
79. {
80. this->error = $e->getMessage();
81. return false;
82. }
83. }
84.
85. function getInstance($mongo_server, $flag=array())
86. {
87. static
88. if (emptyempty($flag['tag']))
89. {
90. 'tag'] = 'default'; }
91. if (isset($flag['force']) && $flag['force'] == true)
92. {
93. new
94. if (emptyempty($mongodb_arr[$flag['tag']]))
95. {
96. 'tag']] = $mongo;
97. }
98. return
99. }
100. else if (isset($mongodb_arr[$flag['tag']]) && is_resource($mongodb_arr[$flag['tag']]))
101. {
102. return $mongodb_arr[$flag['tag']];
103. }
104. else
105. {
106. new
107. 'tag']] = $mongo;
108. return
109.
110. /**
111. * 连接mongodb server
112. *
113. * 参数:无
114. *
115. * 返回值:
116. * 成功:true
117. * 失败:false
118. */
119. function connect()
120. {
121. try
122. this->mongo->connect();
123. return true;
124. }
125. catch
126. {
127. this->error = $e->getMessage();
128. return false;
129. }
130. }
131.
132. /**
133. * select db
134. *
135. * 参数:$dbname
136. *
137. * 返回值:无
138. */
139. function selectDb($dbname)
140. {
141. this->curr_db_name = $dbname;
142. }
143.
144. /**
145. * 创建索引:如索引已存在,则返回。
146. *
147. * 参数:
148. * $table_name:表名
149. * $index:索引-array("id"=>1)-在id字段建立升序索引
150. * $index_param:其它条件-是否唯一索引等
151. *
152. * 返回值:
153. * 成功:true
154. * 失败:false
155. */
156. function ensureIndex($table_name, $index, $index_param=array())
157. {
158. this->curr_db_name;
159. 'safe'] = 1;
160. try
161. this->mongo->$dbname->$table_name->ensureIndex($index, $index_param);
162. return true;
163. }
164. catch
165. {
166. this->error = $e->getMessage();
167. return false;
168. }
169. }
170.
171. /**
172. * 插入记录
173. *
174. * 参数:
175. * $table_name:表名
176. * $record:记录
177. *
178. * 返回值:
179. * 成功:true
180. * 失败:false
181. */
182. function insert($table_name, $record)
183. {
184. this->curr_db_name;
185. try
186. this->mongo->$dbname->$table_name->insert($record, array('safe'=>true));
187. return true;
188. }
189. catch
190. {
191. this->error = $e->getMessage();
192. return false;
193. }
194. }
195.
196. /**
197. * 查询表的记录数
198. *
199. * 参数:
200. * $table_name:表名
201. *
202. * 返回值:表的记录数
203. */
204. function count($table_name)
205. {
206. this->curr_db_name;
207. return $this->mongo->$dbname->$table_name->count();
208. }
209.
210. /**
211. * 更新记录
212. *
213. * 参数:
214. * $table_name:表名
215. * $condition:更新条件
216. * $newdata:新的数据记录
217. * $options:更新选择-upsert/multiple
218. *
219. * 返回值:
220. * 成功:true
221. * 失败:false
222. */
223. function update($table_name, $condition, $newdata, $options=array())
224. {
225. this->curr_db_name;
226. 'safe'] = 1;
227. if (!isset($options['multiple']))
228. {
229. 'multiple'] = 0; }
230. try
231. this->mongo->$dbname->$table_name->update($condition, $newdata, $options);
232. return true;
233. }
234. catch
235. {
236. this->error = $e->getMessage();
237. return false;
238. } }
239.
240. /**
241. * 删除记录
242. *
243. * 参数:
244. * $table_name:表名
245. * $condition:删除条件
246. * $options:删除选择-justOne
247. *
248. * 返回值:
249. * 成功:true
250. * 失败:false
251. */
252. function remove($table_name, $condition, $options=array())
253. {
254. this->curr_db_name;
255. 'safe'] = 1;
256. try
257. this->mongo->$dbname->$table_name->remove($condition, $options);
258. return true;
259. }
260. catch
261. {
262. this->error = $e->getMessage();
263. return false;
264. } }
265.
266. /**
267. * 查找记录
268. *
269. * 参数:
270. * $table_name:表名
271. * $query_condition:字段查找条件
272. * $result_condition:查询结果限制条件-limit/sort等
273. * $fields:获取字段
274. *
275. * 返回值:
276. * 成功:记录集
277. * 失败:false
278. */
279. function find($table_name, $query_condition, $result_condition=array(), $fields=array())
280. {
281. this->curr_db_name;
282. this->mongo->$dbname->$table_name->find($query_condition, $fields);
283. if (!emptyempty($result_condition['start']))
284. {
285. 'start']);
286. }
287. if (!emptyempty($result_condition['limit']))
288. {
289. 'limit']);
290. }
291. if (!emptyempty($result_condition['sort']))
292. {
293. 'sort']);
294. }
295. $result = array();
296. try
297. while
298. {
299. $result[] = $cursor->getNext();
300. }
301. }
302. catch
303. {
304. this->error = $e->getMessage();
305. return false;
306. }
307. catch
308. {
309. this->error = $e->getMessage();
310. return false;
311. }
312. return
313. }
314.
315. /**
316. * 查找一条记录
317. *
318. * 参数:
319. * $table_name:表名
320. * $condition:查找条件
321. * $fields:获取字段
322. *
323. * 返回值:
324. * 成功:一条记录
325. * 失败:false
326. */
327. function findOne($table_name, $condition, $fields=array())
328. {
329. this->curr_db_name;
330. return $this->mongo->$dbname->$table_name->findOne($condition, $fields);
331. }
332.
333. /**
334. * 获取当前错误信息
335. *
336. * 参数:无
337. *
338. * 返回值:当前错误信息
339. */
340. function getError()
341. {
342. return $this->error;
343. }
344. }
345.
346. ?>
数据库连接
1. //⑴默认格式
2. $m = new
3. //这里采用默认连接本机的27017端口,当然你也可以连接远程主机如192.168.0.4:27017,如果端口是27017,端口可以省略
4. //⑵标准连接
5. $m = new Mongo("mongodb://${username}:${password}@localhost");
选择想要的collection:
1. $mo = new
2. $coll = $mo->selectCollection('db', 'mycoll');//得到一个collection对象
插入数据(MongoCollection对象)
1. $coll = $mo->db->foo;
2. $a = array('a' => 'b');
3. $options = array('safe' => true);
4. $rs = $coll->insert($a, $options);
更新数据库中的记录(MongoCollection对象)
1. $coll = $mo->db->coll;
2. $c = array('a' => 1, 's' => array('$lt' => 100));
3. $newobj = array('e' => 'f', 'x' => 'y');
4. $options = array('safe' => true, 'multiple' => true);
5. $rs = $coll->update($c, $newobj, $options);
删除数据库中的记录(MongoCollection对象)
1. $coll = $mo->db->coll;
2. $c = array('a' => 1, 's' => array('$lt' => 100));
3. $options = array('safe' => true);
4. $rs = $coll->remove($c, $options);
查询collection获得多条记录(MongoCollection类)
1. $coll = $mo->db->coll;
2. $query = array('s' => array('$lt' => 100));
3. $fields = array('a' => true, 'b' => true);
4. $rs = $coll->find($query, $fields);
查询collection获得单条记录(MongoCollection类)
1. $coll = $mo->db->coll;
2. $query = array('s' => array('$lt' => 100));
3. $fields = array('a' => true, 'b' => true);
4. $rs = $coll->findOne($query, $fields);
针对游标对象MongoCursor的操作(MongoCursor类):
1. $cursor = $coll->find($query,$fields);
2. while($cursor->hasNext()){
3. $r = $cursor->getNext();
4. var_dump($r);
5. }
6. //或者
7. $cursor = $coll->find($query,$fields);
8. foreache($cursor as $k=>$v){
9. var_dump($v);
10. }
11. //或者
12. $cursor = $coll->find($query,$fields);
13. $array= iterator_to_array($cursor);
删除一个数据库
1. $m -> dropDB("comedy");
删除当前DB
1. $db = $mo->dbname;
2. $db->drop();
列出所有可用数据库
1. $m->listDBs(); //无返回值
获得当前数据库名
1. $db = $mo->dbname;
2. $db->_tostring();
mongoDB 查询 用group 语句 mongodb查询数据
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
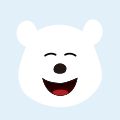
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
mongodb in查询性能 mongodb查询语句大全
MongoDB常用28条查询语句
mongodb in查询性能 mongodb 数据库 java 开发语言