declare --声明
char(15); --定义变量
number(7,2); --数字类型
boolean:=true; --boolean类型
begin --开始
:='HelloWorld'; --赋值
(msg); --输出
end; --结束
--声明的类型为 与emp.sal类型一致的变量
declare
%type; ---和emp表中的 sal类型一样
begin
--sal:=100.99;
select sal into sal from emp where empno=7499;
(sal);
end;
--记录一行
declare ---声明
%rowtype; --emp_rec 变量 类型 emp%rowtype
begin
select * into emp_rec from emp where empno=7499; ---给emp_rec赋值的时候 必须给它一条记录 into emp_rec 赋值的意思
(emp_rec.deptno); ---输出 emp_rec.sal
end; --结束
select * from emp;
--If语句 形式1
declare
number(5,2);
begin
:=80;
if grade>70 then
('成绩优秀');
end if;
end;
--if语句 形式2
declare
number(5,2);
begin
:=60;
if grade>70 then
('成绩优秀');
else
('需要努力');
end if;
end;
--if 语句 形式3
declare
number(5,2);
begin
:=60;
if grade>70 then
('成绩优秀');
elsif grade>50 then ---一定要注意 这里是elsif
('需要努力');
else
('努力');
end if;
end;
accept num prompt('请输入一个数字');--接受键盘输入的值 这里会自动保存到num 中 下面就可以通过plsql语句操作了
declare --声明
number := # --注意 &num &取值的意思 &num把它赋值给 pnum 注意 声明变量 变量 变量类型[:=赋予的值]
begin
(pnum);
if pnum>10 then
('输入的数字大于10');
end if;
end;
---循环语句
declare --声明
number := 1;--注意 声明变量 变量 变量类型[:=赋予的值]
begin
while pnum<10 --while语句
loop --循环的开始
(pnum);
:=pnum+1; --循环改变的条件
end loop; --循环的结束
end;
---循环语句
declare --声明
number := 1;--注意 声明变量 变量 变量类型[:=赋予的值]
begin
loop --循环的开始
exit when pnum>10; --循环退出的条件
(pnum);
:=pnum+1; --循环改变的条件
end loop; --循环的结束
end;
---循环语句
declare --声明
number := 1;--注意 声明变量 变量 变量类型[:=赋予的值]
begin
for i in 1..10
loop --循环的开始
(i);
end loop; --循环的结束
end;
---循环语句
declare --声明
begin
for i in 1..10 --in 变量 变量必须是游标
loop --循环的开始
(i);
end loop; --循环的结束
end;
select * from emp;
--给manager 涨 800 salesaman 400 其它涨 200
declare
%type;
begin
select job into job_rec from emp; --存在问题1 : 返回的job岗位为多个 而 job_rec只能接受一个值
if job_rec = 'MANAGER' then
update emp set sal=sal+800; --存在问题2 : update 语句有where条件
elsif job_rec='SALESMAN' then
update emp set sal=sal+400;
else
update emp set sal=sal+200;
end if;
end;
-- 游标的主要属性如下:
-- %found 布尔属性 如果sql语句至少影响一行 则为true 否则为false
-- %notfound 布尔属性 与%found相反。
-- %isopen 布尔属性 游标是否打开 打开为true 否则为false
-- %rowcount 数字属性 返回受sql语句影响的行数
--查询sql语句
declare
%rowtype;--记录
begin
select * into emp_rec from emp where empno=7499;
('结果'||sql%rowcount);
end;
--更新sql语句
declare
begin
update emp set sal=3000 where empno=7499;
if sql%found then
('更新的记录数'||sql%rowcount);
else
('没有更新记录');
end if;
end;
declare
begin
update emp set sal=3000 where empno=7499;
if sql%notfound then --更新 false
('没有更新记录');
else
('更新的记录数'||sql%rowcount);
end if;
end;
---隐式游标
declare --声明
%rowtype;--记录
begin
for emp_rec in (select * from emp) --in 变量 变量必须是游标
loop --循环的开始
('员工名称:'||emp_rec.ename);
end loop; --循环的结束
end;
--声明游标,打开游标,检索数据,关闭游标。
--声明游标
declare
cursor cl is select * from emp; --查询出所有的emp记录 存储到 cl游标中
%rowtype;--记录
begin
open cl; --打开游标
loop
exit when cl%notfound; --循环退出的条件 cl%notfound 当没有记录的时候 返回true
fetch cl into emp_rec;--检索数据
(emp_rec.empno);
end loop;
close cl;--关闭游标
end;
declare
cursor cl is select * from emp; --查询出所有的emp记录 存储到 cl游标中
begin
for emp_row in cl --打开游标 (自动) 检索数据(自动) 关闭游标(自动) emp_row 变量也不需要定义
loop
(emp_row.ename);
end loop;
end;
--带参数的游标
declare
cursor cl(pno number) is select * from emp where empno=pno; --查询出所有的emp记录 存储到 cl游标中
begin
for emp_row in cl(7499) --打开游标 (自动) 检索数据(自动) 关闭游标(自动) emp_row 变量也不需要定义
loop
(emp_row.ename);
end loop;
end;
--涨工资的案例
--写一段PL/SQL程序,显示部门号为30的员工信息
--例外
--返回多行例外的处理
declare
%rowtype;
begin
select * into emp_row from emp; --隐式游标
exception ---exception 例外的声明
when too_many_rows then --when 例外的名称(系统的例外 也可以是自定例外的名称) then 发生例外执行的操作
('实际返回了多行数据');
end;
--自定义的例外
declare
exception; --声明自定义例外
%rowtype;
cursor cl(pno number) is select *from emp where empno=pno;
begin
open cl(0000);
fetch cl into emp_row;
if cl%notfound then raise no_data; --在这里 抛出例外
end if;
close cl;
exception ---捕获例外
when no_data then
('没有找到数据的错误');
end;
postgresql 打印输出变量
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:实习企业客户服务部门组织架构说明
下一篇:android 3D点云可视化
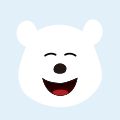
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
日志打印
日志打印
日志打印 Spring日志 日志框架选择与转换 -
Go 环境变量日志打印输出
Go 环境变量日志打印输出
golang 开发语言 后端 字段 互斥锁 -
打印输出图形
打印输出 * 1 3 6 10 15 * 2 5 9 14 * 4 8 13 * 7 12 * 11
java 算法 数据结构 休闲 图形输出 -
javascript 打印输出插件 javascript 打印输出语句
本章技术分享为js的输入和输出语句
js html 方向键 输入输出