导入的拓展包
matplotlib是Python中常用的画图工具, 在日常使用往往需要用到中文, 导入的同时也加入中文拓展包, 会方便很多.
import matplotlib.pyplot as plt
from pyplotz.pyplotz import PyplotZ
from pyplotz.pyplotz import plt
pltz = PyplotZ()
pltz.enable_chinese()
可以在导入程序包的同时, 设定好画图的分辨率以及画布的大小.
plt.rcParams['figure.dpi'] = 200
plt.rcParams['figure.figsize'] = [10,5]
简单折线图
给定一个pandas dataframe, 可以直接采用.plot()的方法画图 (无需import matplotlib).
import pandas as pd
index = [k for k in np.arange(0,1,0.001)]
c1 = pd.Series([k for k in index], index = index, name = 'y=x')
c2 = pd.Series([k*k for k in index], index = index, name = 'y=x^2')
df = pd.concat([c1,c2], axis = 1)
df.plot()
这样可以很方便地画出一个dataframe里面所有列的折线图, label就是列名. 而如果想要个性化列名的话, 可以选择一列一列地画. 对于pandas series来说, 当横坐标输入参数缺省时默认为series的index. 通过参数"color"和"label"可以来决定折线的颜色, 以及折线的标签.
plt.plot(df.iloc[:,0], color = 'black', label = 'y=x')
plt.plot(df.iloc[:,1], color = 'red', label = 'y=square(x)')
plt.legend()
而当需要标签为中文时, plt.legend()由于字体不包括中文, 会出问题. 而使用pltz.legend()就可以囊括中文.
plt.plot(df.iloc[:,0], color = 'black', label = '线性函数')
plt.plot(df.iloc[:,1], color = 'red', label = '抛物线')
pltz.legend()
还可以设定x坐标轴的标签, y坐标轴的标签, 以及整个图的标题.
plt.plot(df.iloc[:,0], color = 'black', label = '线性函数')
plt.plot(df.iloc[:,1], color = 'red', label = '抛物线')
pltz.legend()
pltz.xlabel('x轴')
pltz.ylabel('y轴')
pltz.title('图1: 线性函数与抛物线的函数图像')
当dataframe的列数过多时, 使用循环遍历画图即可. 全画完后再设定坐标标签, 标题, 图形标签等内容即可.
双y轴画图
有时画图的两个数据量级差距太大, 但又想画在同一张图上进行比较. 这个时候可以采用双y轴画图的方法. 注意对于坐标轴的处理要在每个axis的时候声明.
import numpy as np
import pandas as pd
index = [k for k in np.arange(0.1,1,0.001)]
c1 = pd.Series([1/k for k in index], index = index, name = 'y = 1/x')
c2 = pd.Series([np.log(k) for k in index], index = index, name = 'y = log(x)')
df = pd.concat([c1,c2], axis = 1)
fig = plt.figure()
ax1 = fig.add_subplot(111)
ax1.set_ylim([0,15])
ax1.plot(df.iloc[:,0], c = 'C0', label = 'y = 1/x')
ax1.legend()
ax2 = ax1.twinx()
ax2.set_ylim([-3,0])
ax2.plot(df.iloc[:,1], c = 'C1', label = 'y = log(x)')
ax2.legend()
当想给两个纵坐标都取标题的时候, 右边的坐标取标题需要旋转一下角度 (通过参数 rotation 实现), 比较美观. 同时还可以调整坐标轴的标签与坐标轴的距离(通过参数labelpad调整).
import numpy as np
import pandas as pd
index = [k for k in np.arange(0.1,1,0.001)]
c1 = pd.Series([1/k for k in index], index = index, name = 'y = 1/x')
c2 = pd.Series([np.log(k) for k in index], index = index, name = 'y = log(x)')
df = pd.concat([c1,c2], axis = 1)
fig = plt.figure()
ax1 = fig.add_subplot(111)
ax1.set_ylim([0,15])
ax1.plot(df.iloc[:,0], c = 'C0', label = 'y = 1/x')
ax1.legend()
plt.ylabel('y = 1/x')
ax2 = ax1.twinx()
ax2.set_ylim([-3,0])
ax2.plot(df.iloc[:,1], c = 'C1', label = 'y = log(x)')
ax2.legend()
plt.ylabel('y = log(x)', rotation = 270, labelpad = 15);
如果需要让两个图的label放在一起的话,则需要手动设置一下label.
柱状图
DataFrame可以直接绘制柱状图。
import pandas as pd
import numpy as np
index = ['Tom','Amy','Lily']
math = pd.Series([90,95,100], index = index, name = 'Math')
art = pd.Series([100,95,90], index = index, name = 'Art')
df = pd.concat([math,art], axis = 1)
df.plot.bar();
柱子的宽度可以通过传递参数 width 来设置,一个label对应的宽度就是 width,上例中因为一个label对应两根柱子,所以每根柱子的宽度就是 width/2,width 的默认参数一般为0.8.
如果有需要,还可以在柱状图上标注具体的数值
index = ['Tom','Amy','Lily']
math = pd.Series([90,95,100], index = index, name = 'Math')
art = pd.Series([100,95,90], index = index, name = 'Art')
df = pd.concat([math,art], axis = 1)
width = 0.5
df.plot.bar(width = width);
plt.xticks(range(0,len(df)), df.index, rotation = 0);
for a, b in zip(range(0,len(df)), df.Math):
plt.text(a-width/4, b, str(b), ha='center', va='bottom', fontsize=10)
for a, b in zip(range(0,len(df)), df.Art):
plt.text(a+width/4, b, str(b), ha='center', va='bottom', fontsize=10)
或者也可以绘制横着的柱状图
import pandas as pd
import numpy as np
index = ['Tom','Amy','Lily']
math = pd.Series([90,95,100], index = index, name = 'Math')
art = pd.Series([100,95,90], index = index, name = 'Art')
df = pd.concat([math,art], axis = 1)
df.plot.barh();
有需要的话也可以类似地在柱子上标注具体的数字
index = ['Tom','Amy','Lily']
math = pd.Series([90,95,100], index = index, name = 'Math')
art = pd.Series([100,95,90], index = index, name = 'Art')
df = pd.concat([math,art], axis = 1)
width = 0.5
df.plot.barh(width = width);
plt.yticks(range(0,len(df)), df.index, rotation = 0);
for a, b in zip(range(0,len(df)), df.Math):
plt.text(b,a-width/4, str(b), ha='left', va='center', fontsize=10)
for a, b in zip(range(0,len(df)), df.Art):
plt.text(b,a+width/4, str(b), ha='left', va='center', fontsize=10)
饼图
成分构成的饼图可以通过 pd.DataFrame.plot.pie() 直接绘制。
import pandas as pd
index = ['Tom','Amy','Lily']
component = pd.Series([0.15,0.25,0.3,0.1,0.2],
index = ['A','B','C','D','E'],
name = 'component')
component.plot.pie()
可以通过参数 autopct 来给饼图贴上标签。他的取值可以是一个函数,输入变量为 DataFrame 中的数值,输出变量通过函数来自定义。
component.plot.pie(autopct = lambda x: '%.2f'%x+'%')
python画图如何引入宋体库
转载文章标签 python画图如何引入宋体库 python中进行画图常采用的包是 Math 坐标轴 柱状图 文章分类 Python 后端开发
上一篇:任务计划程序启动redis服务
下一篇:java 线程占用内存这么显示
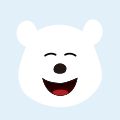
-
python怎么快捷引入报错的包
python报错及处理方法
Python 示例代码 自动安装