使用java语言实现移位密码加密过程
- 一、凯撒密码(移位密码)
- 二、运行软件
- 三、代码
- 1.加密
- 2.解密
- 3.运行
- 四、运行结果
- 1.加密结果
- 2.解密结果
一、凯撒密码(移位密码)
在密码学中,恺撒密码(英语:Caesar cipher),或称恺撒加密、恺撒变换、变换加密,是一种最简单且最广为人知的加密技术。它是一种替换加密的技术,明文中的所有字母都在字母表上向后(或向前)按照一个固定数目进行偏移后被替换成密文。例如,当偏移量是3的时候,所有的字母A将被替换成D,B变成E,以此类推。这个加密方法是以罗马共和时期恺撒的名字命名的,当年恺撒曾用此方法与其将军们进行联系。
二、运行软件
Eclipse是一个开放源代码的、基于 Java 的可扩展开发平台。就其本身而言,它只是一个框架和一组服务,用于通过插件组件构建开发环境。幸运的是,Eclipse附带了一个标准的插件集,包括 Java 开发工具(Java Development Tools,JDT)。
虽然大多数用户很乐于将Eclipse当作 Java IDE 来使用,但Eclipse的目标不仅限于此。Eclipse还包括插件开发环境(Plug-in Development Environment,PDE),这个组件主要针对希望扩展 Eclipse 的软件开发人员,因为它允许他们构建与 Eclipse 环境无缝集成的工具。由于 Eclipse 中的每样东西都是插件,对于给 Eclipse 提供插件,以及给用户提供一致和统一的集成开发环境而言,所有工具开发人员都具有同等的发挥场所。
这种平等和一致性并不仅限于 Java 开发工具。尽管 Eclipse 是使用 Java 语言开发的,但它的用途并不限于 Java 语言;例如,支持诸如 C/C++、COBOL 和 Eiffel 等编程语言的插件已经可用,或预计会推出。Eclipse 框架还可用来作为与软件开发无关的其他应用程序类型的基础,比如内容管理系统。
三、代码
1.加密
public class PutPasswordDemo {
void PutPassword(){
String input = null;
Scanner sc = new Scanner(System.in);
System.out.println("请输入字母:");
input = sc.next();
StringBuffer code = new StringBuffer();
Scanner sc1=new Scanner(System.in);
for(int i = 0;i < input.length();i++)
{
char x = input.charAt(i);
if(x >= 'a' && x <= 'w')
{
x = (char)(x+3);
code.append(x);
}
if(x >= 'x' && x <= 'z')
{
x=(char)(x-23);
code.append(x);
}
if(x >= 'A' && x <= 'W')
{
x = (char) (x+3);
code.append(x);
}
if(x >= 'X' && x <= 'Z')
{
x=(char)(x-23);
code.append(x);
}
}
System.out.println(code);
String str=""+code;
try {
BufferedOutputStream bos=new BufferedOutputStream(
new FileOutputStream("d:\\PutPassword.txt"));
byte[] data=str.getBytes();
bos.write(data);
bos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}}
2.解密
void GetPassword(){
String input = null;
Scanner sc = new Scanner(System.in);
System.out.println("请输入字母:");
input = sc.next();
StringBuffer code = new StringBuffer();
Scanner sc1=new Scanner(System.in);
System.out.println("解密:");
for(int i = 0;i < input.length();i++)
{
char x = input.charAt(i);
if(x >= 'a' && x <= 'c')
{
x=(char)(x+23);
code.append(x);
}
else if(x >= 'd' && x <= 'z')
{
x = (char)(x-3);
code.append(x);
}
else if(x >= 'A' && x <= 'C')
{
x=(char)(x+23);
code.append(x);
}
else if(x >= 'D' && x <= 'Z')
{
x = (char) (x-3);
code.append(x);
}
}
System.out.println(code);
String str=""+code;
try {
BufferedOutputStream bos=new BufferedOutputStream(
new FileOutputStream("d:\\GetPassword.txt"));
byte[] data=str.getBytes();
bos.write(data);
bos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}}
public static void ReadGetFile(){
try {
BufferedInputStream bis=new BufferedInputStream(
new FileInputStream("d:\\GetPassword.txt"));
byte[] data=new byte[1024];
int n=-1;
while((n=bis.read(data, 0, data.length))!=-1){
String str=new String(data,0,n,"utf-8");
System.out.println(str);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}}
public static void ReadPutFile(){
try {
BufferedInputStream bis=new BufferedInputStream(
new FileInputStream("d:\\PutPassword.txt"));
byte[] data=new byte[1024];
int n=-1;
while((n=bis.read(data, 0, data.length))!=-1){
String str=new String(data,0,n,"utf-8");
System.out.println(str);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
3.运行
package charpter;
import java.util.Scanner;
public class MoveDemo {
public static void main(String[] args) {
PutPasswordDemo pp=new PutPasswordDemo();
// String input = null;
// Scanner sc = new Scanner(System.in);
// System.out.println("请输入字母:");
// input = sc.next();
// StringBuffer code = new StringBuffer();
Scanner sc1=new Scanner(System.in);
System.out.println("加密请按1,解密请按2,读取上次加密文件请按3,读取上次解密文件请按4:");
int p;
p=sc1.nextInt();
if(p==1)
pp.PutPassword();
if(p==2)
pp.GetPassword();
if(p==3)
pp.ReadGetFile();
if(p==4)
pp.ReadPutFile();
}
}
四、运行结果
1.加密结果
以我姓名拼音majiarong为明文,偏移量为3时,获得密文pdmldurqj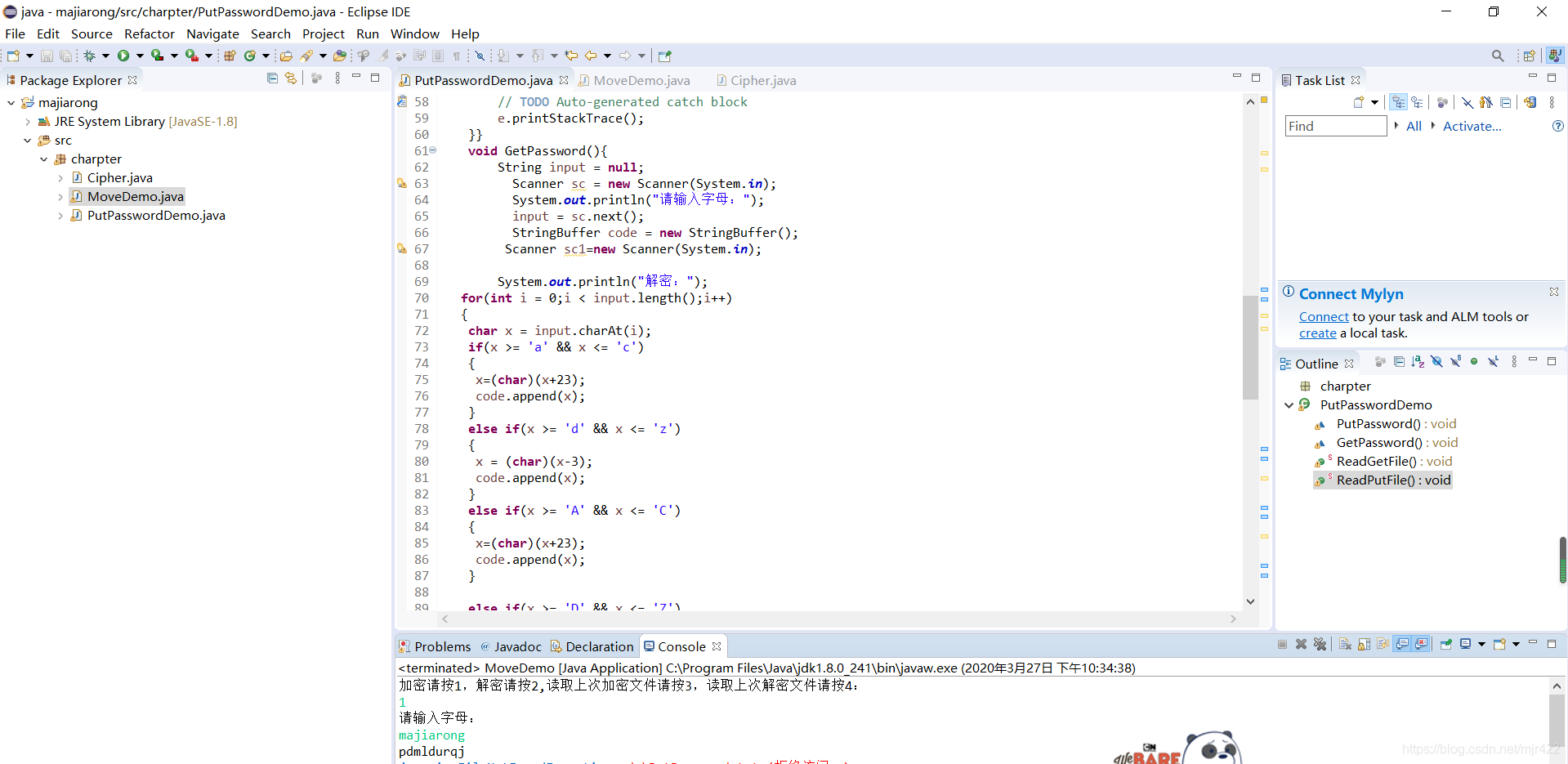
2.解密结果
以pdmldurqj为密文,偏移量为3时,获得明文majiarong我的姓名拼音