1. #ifndef MOOON_SYS_SIMPLE_DB_H
2. #define MOOON_SYS_SIMPLE_DB_H
3. "sys/db_exception.h"
4. string>
5. vector>
6. SYS_NAMESPACE_BEGIN
7.
8. ::vectorstd::string> DBRow; // 用来存储一行所有字段的值
9. ::vectorDBRow> DBTable; // 用来存储所有行
10.
11. /**
12. * 访问DB的接口,是一个抽象接口,当前只支持MySQL
13. *
14. * 使用示例:
15. * db_connection = DBConnection::create_connection("mysql");
16. try
17. {
18. ;
19.
20. // 不指定DB名,以及不需要密码
21. ->set_host("127.0.0.1", 3600);
22. ->set_user("root", "");
23. ->set_charset("utf8");
24. ->enable_auto_reconnect();
25. ->set_timeout_seconds(600);
26.
27. ->open();
28. ->query(db_table, "select count(1) from test_table where id=%d", 2015);
29. }
30. (CDBException& db_error)
31. {
32. log("%s error: %s at %s:%d", db_connection.str().c_str(), db_error.what(), db_error.file(), db_error.lien());
33. }
34.
35. ::destroy_connection(db_connection);
36. */
37. class DBConnection
38. {
39. public:
40. /***
41. * 工厂方法 - 创建一个DB连接
42. * @db_type_name DB类型名,如:mysql、oracle、postgreSQL,不区别大小写
43. * 当前只支持MySQL和SQLite3,也就是参数值只能输入mysql(不区别大小写)
44. * @sql_max 支持的最大SQL语句长度,单位为字节数,不含结尾符
45. * 如果是支持的DB类型,则返回非NULL,否则返回NULL
46. */
47. * create_connection(const std::string& db_type_name, size_t sql_max);
48.
49. /***
50. * 销毁一个由create_connection()创建的DB连接
51. * @db_connection 需要销毁的DB连接
52. */
53. (DBConnection* db_connection);
54.
55. /***
56. * 判断是否为网络连接断开异常,
57. * 如使用过程中,与MySQL间的网络中断,或MySQL进程死掉等,这种情况下可以尝试重连接
58. */
59. (CDBException& db_error);
60.
61. public:
62. ~DBConnection() {}
63.
64. /***
65. * 设置需要连接的DB的IP和服务端口号
66. * 注意,只有在open()或reopen()之前调用才生效
67. */
68. (const std::string& db_ip, uint16_t db_port) = 0;
69.
70. /***
71. * 设置连接的数据库名
72. * 注意,只有在open()或reopen()之前调用才生效
73. */
74. (const std::string& db_name) = 0;
75.
76. /***
77. * 设置用来连接DB的用户名和密码
78. * 注意,只有在open()或reopen()之前调用才生效
79. */
80. (const std::string& db_user, const std::string& db_password) = 0;
81.
82. /***
83. * 设置访问DB的字符集
84. * 注意,只有在open()或reopen()之前调用才生效
85. */
86. (const std::string& charset) = 0;
87.
88. /***
89. * 设置为连接断开后自动重连接,如果不主动设置,默认不自动重连接
90. * 注意,只有在open()或reopen()之前调用才生效
91. */
92. () = 0;
93.
94. /***
95. * 设置用来连接的超时秒数,如果不主动设置,则使用默认的10秒
96. * 注意,只有在open()或reopen()之前调用才生效
97. */
98. (int timeout_seconds) = 0;
99.
100. /***
101. * 设置空值,字段在DB表中的值为NULL时,返回的内容
102. * 如果不主动设置,则默认空值时被设置为"$NULL$"。
103. */
104. (const std::string& null_value) = 0;
105.
106. /***
107. * 建立一个DB连接
108. * 出错抛出异常CDBException
109. */
110. () throw (CDBException) = 0;
111.
112. /**
113. * 关闭一个DB连接
114. * 使用open()建立的连接,在使用完后,要使用close()关闭它
115. */
116. () throw () = 0;
117.
118. /***
119. * 重新建立DB连接
120. * reopen()会先调用close()关闭连接,然后才重新建立连接,
121. * 因此调用reopen()之前,可不调用close(),当然即使调用了close()也不会有问题
122. */
123. () throw (CDBException) = 0;
124.
125. /***
126. * 数据库查询类操作,包括:select, show, describe, explain和check table等,
127. * 如果某字段在DB表中为NULL,则返回结果为"$NULL$",如果内容刚好为"$NULL$",则无法区分
128. * 出错抛出CDBException异常
129. */
130. (DBTable& db_table, const char* format, ...) throw (CDBException) __attribute__((format(printf, 3, 4))) = 0;
131.
132. /**
133. * 查询,期望只返回一行记录,
134. * 如果某字段在DB表中为NULL,则返回结果为空字符串,因此不能区分字段无值还是值为空字符串
135. * 如果查询失败,抛出CDBException异常,异常的错误码为-1,
136. * 如果查询实际返回超过一行记录,抛出CDBException异常,异常的错误码为DB_ERROR_TOO_MANY_ROWS
137. */
138. (DBRow& db_row, const char* format, ...) throw (CDBException) __attribute__((format(printf, 3, 4))) = 0;
139.
140. /**
141. * 查询,期望只返回单行单列,
142. * 如果某字段在DB表中为NULL,则返回结果为空字符串,因此不能区分字段无值还是值为空字符串
143. * 如果查询失败,抛出CDBException异常,异常的错误码为-1,
144. * 如果查询实际返回超过一行记录,抛出CDBException异常,异常的错误码为DB_ERROR_TOO_MANY_ROWS,
145. * 如果查询实际返回只有一行,但超过一列,则抛出CDBException异常,异常的错误码为DB_ERROR_TOO_MANY_COLS
146. */
147. ::string query(const char* format, ...) throw (CDBException) __attribute__((format(printf, 2, 3))) = 0;
148.
149. /***
150. * 数据库insert和update更新操作
151. * 成功返回受影响的记录个数,出错则抛出CDBException异常
152. */
153. int update(const char* format, ...) throw (CDBException) __attribute__((format(printf, 2, 3))) = 0;
154.
155. /**
156. * 取得可读的字符串信息
157. */
158. ::string str() throw () = 0;
159. };
160.
161. SYS_NAMESPACE_END
162. //
mysql8 innodb接口
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
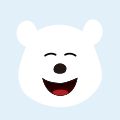
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
centos7安装mysql8
centos7安装mysql8全过程
mysql Server MySQL -
java 项目关闭 请求es 健康检查
随着 Serverless 的流行,将应用迁移到云上已经成了一种必然的趋势。我们今天来看一下如何将机器学习应用迁移到函数计算上。1. 本地开发首先我们看一下本地开发机器学习应用的步骤。我们大概可以将本地开发概括为三个步骤,分别是代码编写,安装依赖,运行调试。我们分别来看一下。1.1 代码编写假定我们的项目结构为:project root ├── index.py ├── model_data │
java 项目关闭 请求es 健康检查 python runtime serverless tensorflow