# coding=utf-8
'''
Created on 2017年11月3日
@author: Administrator
'''
from PyQt4 import QtCore
from PyQt4 import QtGui
from PyQt4 import QtWebKit
from PyQt4 import QtNetwork
# 处理中文问题
import sys, json
# 通过os模块调用系统命令.os模块可以跨平台使用
import os
# 通过wmi可以访问、配置、管理和监视几乎所有的Windows资源
import wmi
# 获取系统的信息
import platform
# 获取cpu 内存 硬盘信息
import psutil
# 用来访问注册表
import winreg
# 检查网卡冗余
import socket
# windows日志
import mmap
import contextlib
# from Evtx.Evtx import FileHeader
# from Evtx.Views import evtx_file_xml_view
from xml.dom import minidom
# sqllist3数据库
import sqlite3
# 获取时间
import datetime
# 导出excel
import xlwt
# 导入多线程
import qthread
import threading
# 时间延迟
import time
# 查看是否安装了raid
import megacli
# 多余的服务
dontService = ['Alerter', 'Clipbook', 'Computer Browser', 'DHCP Client', 'Messenger', 'Remote Registry Service',
'Routing and Remote Access', 'Telnet', 'World Wide Web Publishing', 'Service', 'Print Spooler',
'Terminal Service', 'Task Scheduler']
# 杀毒软件
killVirusSoftware = ['QQPCRTP.exe', '360tray.exe']
killVirusSoftwareName = {'QQPCRTP.exe': '腾讯安全管家', '360tray.exe': '360杀毒'}
hashMapResult = {}
# 生成windows安全策略文件在C盘
def buildWindowsSecurityPolicy():
a = os.popen("secedit /export /cfg c:\gp.inf")
a.close()
# 获取windos策略文件,生成策略文件字典
def windowsSecurityPolicyToDict():
# 声明字典
hashmap = {"a": 1}
# 特殊情况
hashmap['ResetLockoutCount'] = 0
hashmap['LockoutDuration'] = 0
file = r"c:\gp.inf"
f = open(file, "r", encoding="UTF-16LE")
equ = "="
spl = " = "
while True:
data = f.readline()
if equ in data:
if spl in data:
strs = data.split(spl)
hashmap[strs[0]] = strs[1].strip().lstrip().rstrip(',')
else:
strs = data.split(equ)
hashmap[strs[0]] = strs[1].strip().lstrip().rstrip(',')
if not data:
break
f.close()
return hashmap
# 生成windows服务字典
def windowsServiceToDict():
# 默认的sqlserver
hashmap = {'SQL SERVER': 0}
noStatu = 0
for i in dontService:
hashmap[i] = noStatu
wmiobj = wmi.WMI()
services = wmiobj.Win32_Service()
for i in services:
hashmap[str(i.Caption)] = i.State
return hashmap
# 生成windows进程的字典
def windowsProcessToDict():
# 默认的sqlserver
hashmap = {'sqlservr.exe': 0}
result = os.popen('tasklist /fo csv')
res = result.read()
for line in res.splitlines():
process = line.split(",")
newProcess = process[0].replace(process[0][0], '')
hashmap[newProcess] = process[0]
return hashmap
# 生成端口的字典
def portToDict():
hashmap = {"135": 0, "139": 0, "445": 0}
result = os.popen('netstat -na')
res = result.read()
for line in res.splitlines():
if ("0.0.0.0:" in line):
lines = line.split("0.0.0.0:")
line0 = lines[1][0:5].strip()
hashmap[line0] = line0
return hashmap
# 生成公用的字典
def buildCommonMap():
# 系统类型,是windows还是linux
hashmap = {"systemType": platform.system()}
# 系统的默认ttl值
hashmap["Windows"] = 64
hashmap["Windows NT"] = 128
hashmap["Windows 2000"] = 128
hashmap["Windows XP"] = 128
hashmap["Windows 7"] = 64
hashmap["Windows 98"] = 32
hashmap["Linux"] = 64
return hashmap
# 判断ttl是否被修改过
def getIsDefaultTTL():
# return "true"
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE,
r"System\CurrentControlSet\Services\Tcpip\Parameters")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
if ('defaultttl' in k):
strs = str(k).split(",")
if (commonMap[commonMap["systemType"]] == strs[1]):
# 0允许远程桌面连接
return "true"
if ('DefaultTTL' in k):
strs = str(k).split(",")
if (commonMap[commonMap["systemType"]] == strs[1]):
# 0允许远程桌面连接
return "true"
except Exception:
return "false"
pass
winreg.CloseKey(key)
# 判断是否有杀毒软件
def getIsKillSoftware():
for software in killVirusSoftware:
len0 = len(windowsProcess)
windowsProcess[software] = software
if (len(windowsProcess) == len0):
return software
return "false"
# 获取cpu信息
def getCpuInfo():
cpu_count = psutil.cpu_count(logical=False) # 1代表单核CPU,2代表双核CPU
xc_count = psutil.cpu_count() # 线程数,如双核四线程
cpu_slv = round((psutil.cpu_percent(1)), 2) # cpu使用率
list = [cpu_count, xc_count, cpu_slv]
return list
# 获取内存信息
def getMemoryInfo():
memory = psutil.virtual_memory()
total_nc = round((float(memory.total) / 1024 / 1024 / 1024), 2) # 总内存
used_nc = round((float(memory.used) / 1024 / 1024 / 1024), 2) # 已用内存
free_nc = round((float(memory.free) / 1024 / 1024 / 1024), 2) # 空闲内存
syl_nc = round((float(memory.used) / float(memory.total) * 100), 2) # 内存使用率
ret_list = [total_nc, used_nc, free_nc, syl_nc]
return ret_list
# 获取硬盘信息
def getDiskInfo():
list = psutil.disk_partitions() # 磁盘列表
ilen = len(list) # 磁盘分区个数
i = 0
retlist2 = []
while i < ilen:
diskinfo = psutil.disk_usage(list[i].device)
total_disk = round((float(diskinfo.total) / 1024 / 1024 / 1024), 2) # 总大小
used_disk = round((float(diskinfo.used) / 1024 / 1024 / 1024), 2) # 已用大小
free_disk = round((float(diskinfo.free) / 1024 / 1024 / 1024), 2) # 剩余大小
syl_disk = diskinfo.percent
retlist1 = [i, list[i].device, total_disk, used_disk, free_disk, syl_disk] # 序号,磁盘名称,
retlist2.append(retlist1)
i = i + 1
return retlist2
# 判断网络是否连接
def getIsInternet():
result = os.popen('ping www.baidu.com')
res = result.read()
for line in res.splitlines():
if ("正在" in line):
return "true"
# 判断是否开启了桌面远程连接
def getIsDesktopConnection():
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\Control\Terminal Server")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
if ('fDenyTSConnections' in k):
strs = str(k).split(",")
if (' 0' == strs[1]):
# 0允许远程桌面连接
return 0
else:
# 1不允许远程桌面连接
return 1
except Exception:
pass
# print(Exception)
winreg.CloseKey(key)
# 判断禁止进入系统BOIS进行设置
def getIsBanBios():
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\USBSTOR")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
if ('Start' in k):
strs = str(k).split(",")
if (' 3' == strs[1]):
# 允许
return 3
else:
# 不允许
return 4
except Exception:
pass
# print(Exception)
winreg.CloseKey(key)
# 判断是否开启默认分区共享
def getIsSharedPartitions():
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\LanmanServer\Parameters")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
if ('AutoShareServer' in k):
strs = str(k).split(",")
if (' 0' == strs[1]):
# 已关闭分区默认共享
return 0
else:
# 开启分区默认共享
return 1
except Exception:
pass
# print(Exception)
winreg.CloseKey(key)
# 判断是否开启默认共享
def getIsShared():
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\LanmanServer\Parameters")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
if ('AutoShareWks' in k):
strs = str(k).split(",")
if (' 0' == strs[1]):
# 已关闭默认共享
return 0
else:
# 开启默认共享
return 1
except Exception:
pass
# print(Exception)
winreg.CloseKey(key)
# 判断是否是默认日志大小
def getIsDefalutLogSize():
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\eventlog\Security")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
if ('MaxSize' in k):
if (20971520 in k):
# 默认日志大小
return 0
else:
return 1
except Exception:
pass
# print(Exception)
winreg.CloseKey(key)
# 获取日志的地址
def getLogPaths():
list = []
# Security日志文件地址
key0 = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\eventlog\Security")
try:
i = 0
while True:
k0 = winreg.EnumValue(key0, i)
i += 1
# print(k)
if ('File' in k0 and 'DisplayNameFile' not in k0):
paths = k0[1]
list.append(paths)
except Exception:
pass
# print()
winreg.CloseKey(key0)
# Application日志文件地址
key1 = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\eventlog\Application")
try:
i = 0
while True:
k1 = winreg.EnumValue(key1, i)
i += 1
# print(k)
if ('File' in k1 and 'DisplayNameFile' not in k1):
paths = k1[1]
list.append(paths)
except Exception:
pass
# print()
winreg.CloseKey(key1)
# System日志文件地址
key2 = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\services\eventlog\System")
try:
i = 0
while True:
k2 = winreg.EnumValue(key2, i)
i += 1
# print(k)
if ('File' in k2 and 'DisplayNameFile' not in k2):
paths = k2[1]
list.append(paths)
except Exception:
pass
# print()
winreg.CloseKey(key2)
# 系统盘
systemDisk = os.getenv("SystemDrive")
listNew = []
for path in list:
path1 = path.replace('%SystemRoot%', systemDisk + "//Windows")
listNew.append(path1)
# print(path1)
return listNew
# \system32\winevt\Logs\Application.evtx
# 过滤掉不需要的事件,输出感兴趣的事件
def InterestEvent(xml, EventID):
xmldoc = minidom.parseString(xml)
root = xmldoc.documentElement
# print(root.childNodes)
# 获取EventID节点的事件ID
# booknode=root.getElementsByTagName('event')
# for booklist in booknode:
# bookdict={}
# bookdict['id']=booklist.getAttribute('id')
# bookdict['head']=booklist.getElementsByTagName('head')[0].childNodes[0].nodeValue.strip()
# bookdict['name']=booklist.getElementsByTagName('name')[0].childNodes[0].nodeValue.strip()
# bookdict['number']=booklist.getElementsByTagName('number')[0].childNodes[0].nodeValue.strip()
# bookdict['page']=booklist.getElementsByTagName('page')[0].childNodes[0].nodeValue.strip()
# if EventID == eventId:
# print xml
# 判断是否是打开防火墙
def getIsFirewall():
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE,
r"SYSTEM\CurrentControlSet\Services\SharedAccess\Parameters\FirewallPolicy\StandardProfile")
try:
i = 0
while True:
k = winreg.EnumValue(key, i)
i += 1
# print(k)
if ('EnableFirewall' in k):
if ('0' in k):
# 关闭防火墙
return 0
else:
# 打开防火墙
return 1
except Exception:
# print(Exception)
pass
winreg.CloseKey(key)
# 判断网卡冗余
def getIsNicRedundancy():
i = 0
for ip in socket.gethostbyname_ex(socket.gethostname())[2]:
localIP = ip
i = i + 1
if (i >= 2):
return 1
return 0
# 判断是否安装了raid
def getIsRaid():
try:
cli = megacli.MegaCLI()
cli.bbu()
# 安装了
return 1
except:
# 没有安装
return 0
# 判断用户是否需要密码
def getIsRequiredPassword():
result = os.popen('wmic useraccount list full')
res = result.read()
i = 0
count = 0
name0 = str(securityPolicy['NewAdministratorName'])
name = name0.replace(name0[0], '')
for line in res.splitlines():
if (name in line):
i = 1
if (i == 1):
count = count + 1
if (i == 1 and 'PasswordRequired=TRUE' in line and count <= 11):
return 1
if (count > 12):
return 0
# 获取管理员下面所有的用户
def buildUserList():
result = os.popen('Net Localgroup administrators')
res = result.read()
list = []
count = 0
for line in res.splitlines():
if ('成功完成' in line):
return list
if (count == 1):
list.append(line)
if ('---' in line):
count = 1
# 创建数据库和用户表
def buildDatabase():
conn = sqlite3.connect('baseline.db')
# print("Opened database successfully")
c = conn.cursor()
c.execute('''CREATE TABLE USER
(ID INT PRIMARY KEY NOT NULL,
NAME TEXT NOT NULL,
AGE INT NOT NULL,
LOGIN_TIME timestamp NOT NULL,
ADDRESS CHAR(50),
SALARY REAL);''')
c.execute("INSERT INTO USER (ID,NAME,AGE,LOGIN_TIME,ADDRESS,SALARY) \
VALUES (1, 'duke', 32,'2016-01-22 08:45:50', 'California', 20000.00 )");
# print("Table created successfully")
conn.commit()
conn.close()
# 显示结果1身份鉴别
def printResult1_2():
hashMap = {}
# print("任务2-->用户名称:" + securityPolicy['NewAdministratorName'])
task_2_0 = "不需要口令"
requiredPassword = getIsRequiredPassword()
if (requiredPassword == 1):
task_2_0 = "需要口令"
# print("任务2-->是否需要用户口令:" + task_2_0)
hashMap["NewAdministratorName"] = securityPolicy['NewAdministratorName'][1:][:-1]
hashMap["requiredPassword_dict"] = task_2_0
hashMap["requiredPassword"] = requiredPassword
return hashMap
# 假数据,争取做到100分
def oneHundred():
global hashMapResult
hashMapResult["PasswordComplexity"]='1'
hashMapResult["PasswordComplexity_dict"] = "已启用"
hashMapResult["Bois_dict"] = "禁用"
hashMapResult["Bois"] = 4
hashMapResult["NewAdministratorName16_dict"] = "没有"
hashMapResult["NewAdministratorName16"] = "test"
hashMapResult["software29_dict"] = "没有"
hashMapResult["software29"] = 1
hashMapResult["dontService_dict"] = "没有多余服务"
hashMapResult["dontService"] = "没有多余服务"
hashMapResult['PasswordComplexity'] = '1'
class DemoWin(QtWebKit.QWebView):
# signal一定要在init前,具体原因不清楚
start_to_think_signal = QtCore.pyqtSignal(int, str)
def __init__(self):
QtWebKit.QWebView.__init__(self)
# self.resize(920, 600)
self.setUrl(QtCore.QUrl('views/indexThread.html'))
self.setWindowTitle('基线检查工具')
self.setFixedWidth(920)
self.setFixedHeight(590)
self.show()
mainFrame = self.page().mainFrame()
winobj = WinObj(mainFrame)
mainFrame.javaScriptWindowObjectCleared.connect(
lambda: mainFrame.addToJavaScriptWindowObject('WinObj', winobj)) ##js调用python
def deal(self):
try:
# print("任务2-->是否需要用户口令:")
self.genare_thread = WmThread()
self.genare_thread.start()
except Exception as e:
print(e)
class WinObj(QtCore.QObject):
def __init__(self, mainFrame):
super(WinObj, self).__init__()
self.mainFrame = mainFrame
@QtCore.pyqtSlot(result="QString")
def getInfo(self):
dic_info = {1}
# 调用js函数,实现回调
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('onGetInfo', json.dumps(dic_info)))
return json.dumps(dic_info)
@QtCore.pyqtSlot(result="QString")
def printResult1_2_0(self):
# print(hashMapResult)
global hashMapResult
DemoWin.deal(self)
@QtCore.pyqtSlot(result="QString")
def printResult1_2(self):
# print(hashMapResult)
global hashMapResult
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_2', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_3', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_5', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_7', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_8', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_10', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_12', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_15', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_16', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_17', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_20', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_21', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_22', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_24', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult5_29', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult5_30', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult5_36', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult6_39', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult7_41', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult7_43', json.dumps(hashMapResult)))
self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult8_46', json.dumps(hashMapResult)))
# print(hashMapResult)
# 获取上次登录时间
@QtCore.pyqtSlot(result="QString")
def getCurrentUsers(self):
hashMap = {}
conn = sqlite3.connect('baseline.db')
# print("Opened database successfully")
c = conn.cursor()
cursor = c.execute("SELECT id, name,login_time, address, salary from USER")
for row in cursor:
hashMap["login_time"] = row[2]
hashMap["name"] = row[1]
# print("Table select successfully")
conn.commit()
conn.close()
return json.dumps(hashMap)
# 获取上次登录时间
@QtCore.pyqtSlot(result="QString")
def updateCurrentUsersTime(self):
hashMap = {}
id = 0
conn = sqlite3.connect('baseline.db')
# print("Opened database successfully")
c = conn.cursor()
cursor = c.execute("SELECT id, name,login_time, address, salary from USER")
for row in cursor:
hashMap["login_time"] = row[2]
hashMap["name"] = row[1]
id = row[0]
# print("Table select successfully")
now = datetime.datetime.now()
sql0 = "UPDATE USER set login_time = " + "'" + datetime.datetime.strftime(now,
'%Y-%m-%d %H:%M:%S') + "'" + " where id=" + str(
id)
c.execute(sql0)
conn.commit()
conn.close()
# 打印
@QtCore.pyqtSlot(str)
def printExcel(self, strVal):
# print(strVal)
hashMap = strVal.replace(" ", "").split(",")
# print(len(hashMap))
list1 = []
i_0 = 0
for map1 in hashMap:
list1.append(map1.split("<>"))
i_0 = i_0 + 1
f = xlwt.Workbook()
sheet1 = f.add_sheet('检查', cell_overwrite_ok=True)
row0 = ["检查项", "检查结果"]
# 写第一行
for i in range(0, len(row0)):
sheet1.write(0, i, row0[i], set_style('Times New Roman', 220, 0, True))
# 设置宽度
sheet1.col(0).width = 256 * 11 * 4
sheet1.col(1).width = 256 * 11 * 5
sheet1.col(2).width = 256 * 11 * 6
# 写第一行
for i in range(0, len(list1)):
sheet1.write(i + 1, 0, list1[i][0], set_style('Times New Roman', 220, 0, True))
if (list1[i][2] == "0"):
sheet1.write(i + 1, 1, list1[i][1], set_style('Times New Roman', 220, 2, True))
else:
sheet1.write(i + 1, 1, list1[i][1], set_style('Times New Roman', 220, 0, True))
sheet1.write(i + 1, 2, list1[i][3], set_style('Times New Roman', 220, 0, True))
# print(get_desktop())
f.save(get_desktop() + "\\" + "基线检查结果.xls")
hashMap = {}
hashMap["result"] = 1
return json.dumps(hashMap)
# 添加线程
class WmThread(QtCore.QThread):
finished_signal = QtCore.pyqtSignal(int) # 使用PySide2模块需要将pyqtSignal改成Signal
def __init__(self, parent=None):
super().__init__(parent)
def run(self):
try:
global hashMapResult
hashMapResult = {}
# task_2_0 = "不需要口令"
requiredPassword = getIsRequiredPassword()
if (requiredPassword == 1):
task_2_0 = "需要口令"
# print("任务2-->是否需要用户口令:" + task_2_0)
hashMapResult["NewAdministratorName"] = securityPolicy['NewAdministratorName'][1:][:-1]
hashMapResult["requiredPassword_dict"] = task_2_0
hashMapResult["requiredPassword"] = requiredPassword
hashMapResult["grade_result1_2"] = 5
# hashMap = {}
grade = 0
task3_0 = "没有启用"
task3_2 = "否"
if securityPolicy['PasswordComplexity'] == '1':
task3_0 = "已启用"
grade = grade + 1
# print("任务3-->是否启用本机组策略中“密码必须符合复杂性要求”策略:" + task3_0)
task3_1 = "小于8位"
if int(securityPolicy['MinimumPasswordLength']) > 8:
task3_1 = "大于8位"
grade = grade + 1
# print("任务3-->口令长度不得小于8位:" + task3_1)
# print("任务3-->是否为字母、数字或特殊字符的混合组合:" + task3_2)
task3_2 = "不是"
if int(securityPolicy['LockoutBadCount']) < 5:
task3_2 = "是"
grade = grade + 1
# print("任务3-->口令不重复的次数5次:" + task3_2)
task3_3 = "不是"
if int(securityPolicy['MaximumPasswordAge']) < 90:
task3_3 = "是"
grade = grade + 1
# print("任务3-->每三个月修改一次口令:" + task3_3)
task3_4 = "未禁用"
if securityPolicy['RequireLogonToChangePassword'] == '0':
task3_4 = "已禁用"
grade = grade + 1
# print("任务3-->禁用可还原密码:" + task3_4)
hashMapResult["PasswordComplexity_dict"] = task3_0
hashMapResult["PasswordComplexity"] = securityPolicy['PasswordComplexity']
hashMapResult["MinimumPasswordLength_dict"] = task3_1
hashMapResult["MinimumPasswordLength"] = securityPolicy['MinimumPasswordLength']
hashMapResult["MaximumPasswordAge_dict"] = task3_3
hashMapResult["MaximumPasswordAge"] = securityPolicy['MaximumPasswordAge']
hashMapResult["RequireLogonToChangePassword_dict"] = task3_4
hashMapResult["RequireLogonToChangePassword"] = securityPolicy['RequireLogonToChangePassword']
hashMapResult["grade_result1_3"] = grade
# hashMap = {}
grade = 0
task5_0 = "没有"
if int(securityPolicy['LockoutBadCount']) == 5:
task5_0 = "已"
grade = grade + 2
# print("任务5-->限制同一用户连续5次失败登录即锁定:" + task5_0)
task5_1 = "少于"
if int(securityPolicy['ResetLockoutCount']) >= 11:
task5_1 = "大于"
grade = grade + 2
# print("任务5-->锁定时间不少于10分钟:" + task5_1)
task5_2 = "没有"
if int(securityPolicy['LockoutDuration']) == 10:
task5_2 = "已"
grade = grade + 1
# print("任务5-->应开启屏幕保护中的密码保护功能并将时间设定为10分钟:" + task5_2)
hashMapResult["LockoutBadCount_dict"] = task5_0
hashMapResult["LockoutBadCount"] = securityPolicy['LockoutBadCount']
hashMapResult["ResetLockoutCount_dict"] = task5_1
hashMapResult["ResetLockoutCount"] = securityPolicy['ResetLockoutCount']
hashMapResult["LockoutDuration_dict"] = task5_2
hashMapResult["LockoutDuration"] = securityPolicy['LockoutDuration']
hashMapResult["grade_result1_5"] = grade
# hashMap = {}
grade = 5
desktopConnection = getIsDesktopConnection()
task7_0 = "不允许"
if (desktopConnection == 0):
task7_0 = "允许"
grade = 0
# print("任务7-->能探测出远程桌面是否关闭:" + task7_0)
hashMapResult["IsDesktopConnection_dict"] = task7_0
hashMapResult["IsDesktopConnection"] = desktopConnection
hashMapResult["grade_result1_7"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_7', json.dumps(hashMap)))
# hashMap = {}
task8_0 = "显示"
grade = 0
if \
securityPolicy[
'MACHINE\Software\Microsoft\Windows\CurrentVersion\Policies\System\DontDisplayLastUserName'].split(
",")[1] == '1':
task8_0 = "不显示"
grade = 5
# print("任务8-->每次登录均不显示上次登录帐户名:" + task8_0)
hashMapResult["DontDisplayLastUserName_dict"] = task8_0
hashMapResult["DontDisplayLastUserName"] = securityPolicy[
'MACHINE\Software\Microsoft\Windows\CurrentVersion\Policies\System\DontDisplayLastUserName'].split(
",")[1]
hashMapResult["grade_result1_8"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_8', json.dumps(hashMap)))
# hashMap = {}
# print()
banBios = getIsBanBios()
task10_0 = "禁止"
grade = 5
# 3代表可以进入 4代表不可进入
if (banBios == 3):
task10_0 = "允许"
grade = 0
# print("任务10-->是否禁止进入系统BOIS进行设置:" + task10_0)
hashMapResult["Bois_dict"] = task10_0
hashMapResult["Bois"] = banBios
hashMapResult["grade_result1_10"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult1_10', json.dumps(hashMap)))
hashMap = {}
grade = 5
# print()
sharedPartitions = getIsSharedPartitions()
task12_0 = "关闭"
# 0是关闭 1是开启
if (sharedPartitions == 1):
task12_0 = "开启"
grade = grade - 2
# print("任务12-->是否关闭自定义共享:" + task12_0)
shared = getIsShared()
task12_1 = "关闭"
# 0是关闭 1是开启
if (shared == 1):
task12_1 = "开启"
grade = grade - 3
# print("任务12-->是否关闭默认共享:" + task12_1)
hashMapResult["sharedPartitions_dict"] = task12_0
hashMapResult["sharedPartitions"] = sharedPartitions
hashMapResult["shared_dict"] = task12_1
hashMapResult["shared"] = shared
hashMapResult["grade_result2_12"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_12', json.dumps(hashMap)))
# hashMap = {}
# print()
grade = 5
userlist = len(buildUserList())
task_15_0 = "不"
if (userlist >= 2):
task_15_0 = "已"
grade = 0
# print("任务15-->应实现操作系统和数据库系统特权用户的权限分离:" + "未知")
# print("任务15-->是否存在多个管理员公用一个账号的情况:" + task_15_0)
sqlserver0 = windowsService['SQL SERVER']
sqlserver1 = windowsProcess['sqlservr.exe']
test0 = 0
task_15_1 = "安装"
if (sqlserver0 != 0 or sqlserver1 != 0):
test0 = test0 + 0
else:
task_15_1 = "没有安装"
# print("任务15-->是否安装sqlserver数据库:" + task_15_1)
hashMapResult["userlist_dict"] = task_15_0
hashMapResult["userlist"] = userlist
hashMapResult["sqlServer_dict"] = task_15_1
hashMapResult["grade_result2_15"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_15', json.dumps(hashMap)))
# hashMap = {}
# print()
task16_0 = "未禁用"
grade = 0;
# 0禁用
if securityPolicy['EnableGuestAccount'] == '0':
task16_0 = "禁用"
grade = grade + 2
# print("任务16-->限制Guest等默认账号的权限:" + task16_0)
task16_1 = "没有"
if securityPolicy['NewAdministratorName'][1:][:-1] != 'Administrator':
task16_1 = "是"
grade = grade + 2
# print("任务16-->重命名系统默认帐户:" + task16_1)
task16_2 = "没有"
if securityPolicy['PasswordComplexity'] == '1':
task16_2 = "已"
grade = grade + 1
# print("任务16-->帐户口令设置应满足口令设置要求:" + task16_2)
hashMapResult["EnableGuestAccount_dict"] = task16_0
hashMapResult["EnableGuestAccount"] = securityPolicy['EnableGuestAccount']
hashMapResult["NewAdministratorName16_dict"] = task16_1
hashMapResult["NewAdministratorName16"] = securityPolicy['NewAdministratorName'][1:][:-1]
hashMapResult["PasswordComplexity16_dict"] = task16_2
hashMapResult["PasswordComplexity16"] = securityPolicy['PasswordComplexity']
hashMapResult["grade_result2_16"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_16', json.dumps(hashMap)))
# hashMap = {}
grade = 0
# print()
task17_2 = "没有及时"
if int(securityPolicy['MaximumPasswordAge']) <= 93:
task17_2 = "及时"
grade = 5
# print("任务17-->应及时删除多余的、过期的帐户,避免共享帐户的存在:" + task17_2)
hashMapResult["MaximumPasswordAge_dict"] = task17_2
hashMapResult["MaximumPasswordAge"] = int(securityPolicy['MaximumPasswordAge'])
hashMapResult["grade_result2_17"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult2_17', json.dumps(hashMap)))
# hashMap = {}
# print()
grade = 0
task20_1 = "没有启用"
if str(windowsService['Windows Event Log']) == 'Running':
task20_1 = "启用"
grade = 5
# print("任务20-->是否启用操作系统日志功能,日志记录应该覆盖系统、应用程序、安全及每一个用户:" + task20_1)
hashMapResult["eventLog_dict"] = task20_1
hashMapResult["eventLog"] = str(windowsService['Windows Event Log'])
hashMapResult["grade_result3_20"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_20', json.dumps(hashMap)))
# hashMap = {}
grade = 0
# print()
task21_0 = "无审核"
if securityPolicy['AuditAccountManage'] != '0':
task21_0 = "审核"
grade = grade + 2
# print("任务21-->用户的添加和删除:" + task21_0)
hashMapResult["AuditAccountManage210_dict"] = task21_0
hashMapResult["AuditAccountManage210"] = securityPolicy['AuditAccountManage']
task21_1 = "没有开启"
if securityPolicy['AuditAccountManage'] != '0':
task21_1 = "开启"
grade = grade + 1
# print("任务21-->审计功能的启动和关闭:" + task21_1)
task21_2 = "没有调整"
if securityPolicy['AuditPolicyChange'] != '0':
task21_2 = "已调整"
grade = grade + 1
# print("任务21-->审计策略的调整:" + task21_2)
task21_3 = "没有"
if securityPolicy['AuditLogonEvents'] != '0':
task21_3 = "有"
grade = grade + 1
# print("任务21-->重要的系统操作(如用户登录、退出)等:" + task21_3)
hashMapResult["AuditAccountManage211_dict"] = task21_1
hashMapResult["AuditAccountManage211"] = securityPolicy['AuditAccountManage']
hashMapResult["AuditPolicyChange_dict"] = task21_2
hashMapResult["AuditPolicyChange"] = securityPolicy['AuditPolicyChange']
hashMapResult["AuditLogonEvents_dict"] = task21_3
hashMapResult["AuditLogonEvents"] = securityPolicy['AuditLogonEvents']
hashMapResult["grade_result3_21"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_21', json.dumps(hashMap)))
# hashMap = {}
# print()
# print("任务22-->日志记录应包括日期和时间、类型、主体标识、客体标识、事件的结果等:" + "未知")
hashMapResult["logListPath_dict"] = "未知"
hashMapResult["logListPath"] = "未知"
hashMapResult["grade_result3_22"] = 5
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_22', json.dumps(hashMap)))
# hashMap = {}
# print()
grade = 0
task24_3 = "默认日志容量"
logSize = getIsDefalutLogSize()
if logSize == 1:
task24_3 = "修改过日志容量"
grade = 5
# print("任务24-->应设置合理的日志文件容量,确保日志信息的完整性:" + task24_3)
hashMapResult["logSize_dict"] = task24_3
hashMapResult["logSize"] = logSize
hashMapResult["grade_result3_24"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult3_24', json.dumps(hashMap)))
# hashMap = {}
# print()
# print("任务29-->关闭Windows多余的组件,禁止安装其他与应用系统无关的应用程序:" + "未知")
hashMapResult["software29_dict"] = "未知"
hashMapResult["software29"] = "未知"
hashMapResult["grade_result5_29"] = 5
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult5_29', json.dumps(hashMap)))
# hashMap = {}
# print()
grade = 5
task_30_0 = "没有多余服务"
for service0 in dontService:
if (str(windowsService[service0]) == "Running"):
task_30_0 = service0
# service0 + str(windowsService[service0])
grade = grade - 3
break
# print("任务30-->关闭多余服务:" + task_30_0)
shared = getIsShared()
task12_1 = "关闭"
if (shared == 1):
task12_1 = "开启"
grade = grade - 2
# print("任务30-->删除系统默认共享:" + task12_1)
hashMapResult["dontService_dict"] = task_30_0
hashMapResult["dontService"] = task_30_0
hashMapResult["grade_result5_30"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult5_30', json.dumps(hashMap)))
# hashMap = {}
# print()
windowsVersion = platform.platform(True)
# print("任务36-->系统版本信息:" + windowsVersion)
task_36_0 = "不是默认值"
grade = 0
isDefaultTTL = getIsDefaultTTL()
if ("true" == isDefaultTTL):
task_36_0 = "是默认值"
grade = 5
# print("任务36-->更改默认TTL返回值:" + task_36_0)
hashMapResult["isDefaultTTL_dict"] = task_36_0
hashMapResult["isDefaultTTL"] = isDefaultTTL
hashMapResult["grade_result5_36"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult5_36', json.dumps(hashMap)))
# hashMap = {}
# print()
task_39_0 = "没有安装"
task_39_1 = "无"
if ("false" != getIsKillSoftware()):
task_39_0 = "安装了"
task_39_1 = killVirusSoftwareName[getIsKillSoftware()]
# print("任务39-->是否安装了杀毒软件:" + task_39_0)
# print("任务39-->杀毒软件名称:" + task_39_1)
hashMapResult["IsKillSoftware_dict"] = task_39_0
hashMapResult["IsKillSoftware"] = getIsKillSoftware()
hashMapResult["KillSoftware_dict"] = task_39_1
hashMapResult["KillSoftware"] = task_39_1
hashMapResult["grade_result6_39"] = 5
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult6_39', json.dumps(hashMap)))
# hashMap = {}
# print()
task_41_0 = "关闭状态"
grade = 0
isfirewall = getIsFirewall()
if (1 == isfirewall):
task_41_0 = "打开状态"
grade = 5
# print("任务41-->是否关闭防火墙:" + task_41_0)
# print("任务41-->限定服务器的地址访问:" + "未知")
hashMapResult["isfirewall_dict"] = task_41_0
hashMapResult["isfirewall"] = isfirewall
hashMapResult["grade_result7_41"] = grade
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult7_41', json.dumps(hashMap)))
# hashMap = {}
# print()
cpuInfo = getCpuInfo()
# print("任务43-->监视服务器的CPU、硬盘、内存、网络等资源的使用情况:")
# print("任务43-->cpu数量:" + str(cpuInfo[0]) + "核")
memoryInfo = getMemoryInfo()
# print("任务43-->内存大小:" + str(memoryInfo[0]) + "G")
diskInfo = getDiskInfo()
totalDisk = 0
for diskinfo in diskInfo:
totalDisk = totalDisk + diskinfo[2]
# print("任务43-->硬盘大小:" + str(totalDisk) + "G")
task_43_0 = "未连通"
isInternet = getIsInternet()
if (isInternet == "true"):
task_43_0 = "已连通"
# print("任务43-->网络是否连通:" + task_43_0)
hashMapResult["cpuInfo_dict"] = str(cpuInfo[0])
hashMapResult["memoryInfo_dict"] = str(memoryInfo[0])
hashMapResult["diskinfo_dict"] = str(totalDisk)
hashMapResult["isInternet_dict"] = task_43_0
hashMapResult["grade_result7_43"] = 5
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult7_43', json.dumps(hashMap)))
# hashMap = {}
# print()
raid = getIsRaid()
task_46_0 = "没有主机磁盘raid"
if (raid == 1):
task_46_0 = "有主机磁盘raid"
hashMapResult["raid_dict"] = task_46_0
hashMapResult["raid"] = raid
nicRedundancy = getIsNicRedundancy()
task_46_1 = "没有冗余"
if (nicRedundancy == 1):
task_46_1 = "网卡冗余"
# print("任务46-->能采集主机磁盘(RAID)、网卡等关键部件冗余、双机热备(cluster集群)等硬件内容:" + "未知")
# print("任务46-->网卡是否冗余:" + task_46_1)
hashMapResult["nicRedundancy_dict"] = task_46_1
hashMapResult["nicRedundancy"] = nicRedundancy
hashMapResult["grade_result8_46"] = 5
# print()
# self.mainFrame.evaluateJavaScript('%s(%s)' % ('showResult8_46', json.dumps(hashMap)))
# print(hashMapResult["NewAdministratorName"])
# print(hashMapResult)
#oneHundred()
except Exception as e:
# self.finished_signal.emit(0)
pass
# 设置表格样式
def set_style(name, weight, color, bold=False):
style = xlwt.XFStyle()
font = xlwt.Font()
# 设置字体类型
font.name = name
# 设置字体粗体
# font.bold = bold
# 设置字体颜色
font.colour_index = color
font._weight = weight
style.font = font
return style
def get_desktop():
key = winreg.OpenKey(winreg.HKEY_CURRENT_USER, \
r'Software\Microsoft\Windows\CurrentVersion\Explorer\Shell Folders', )
return winreg.QueryValueEx(key, "Desktop")[0]
class viewsThread(threading.Thread):
def __init__(self, threadID, name, counter):
threading.Thread.__init__(self)
self.threadID = threadID
self.name = name
self.counter = counter
def run(self): # 把要执行的代码写到run函数里面 线程在创建后会直接运行run函数
app = QtGui.QApplication(sys.argv)
demoWin = DemoWin()
sys.exit(app.exec_())
if __name__ == '__main__':
buildWindowsSecurityPolicy()
commonMap = buildCommonMap()
securityPolicy = windowsSecurityPolicyToDict()
windowsService = windowsServiceToDict()
windowsProcess = windowsProcessToDict()
# 创建新线程
app = QtGui.QApplication(sys.argv)
demoWin = DemoWin()
sys.exit(app.exec_())
# thread1 = DemoWinThread(1, "Thread-1", 1)
# thread1.start()
python Hough线检测 python基线检查脚本
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
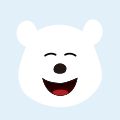
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Linux java 线程卡死
文章目录同步问题引出线程同步处理线程死锁 在多线程的处理之中,可以利用Runnable描述多个线程的操作资源,而Thread描述每一个线程对象,于是当多个线程访问统一资源的时候,如果处理不当就会产生数据的错误操作。 同步问题引出 下面编写一个简单的卖票程序,将创建若干个线程对象
Linux java 线程卡死 同步 死锁 synchronized System