func main() {
cmd := exec.Command("ls", "-lah")
out, err := cmd.CombinedOutput()
if err != nil {
log.Fatalf("cmd.Run() failed with %s\n", err)
}
fmt.Printf("combined out:\n%s\n", string(out))
//比如我现在有个定时任务需要执行,封装一个方法自动执行
cmdStr := "cd /go/leyangjun/test/ && ./main crontab --run=single --job=myCrontabName"
runCmd := exec.Command("/bin/bash", "-c", cmdStr)
runCmdOut, err := runCmd.CombinedOutput()
if err != nil {
fmt.Println("命令执行失败")
}else{
fmt.Println("命令执行成功")
}
}
//检查命令是否存在
func checkExists() {
path, err := exec.LookPath("pwd")
if err != nil {
fmt.Printf("pwd不存在\n")
} else {
fmt.Printf("'pwd' executable is in '%s'\n", path)
}
}
//两个命令依次执行
func successivelyExists() {
c1 := exec.Command("ls")
c2 := exec.Command("wc", "-l")
c2.Stdin, _ = c1.StdoutPipe()
c2.Stdout = os.Stdout
_ = c2.Start()
_ = c1.Run()
_ = c2.Wait()
}
//按行读取输出的内容
func readByRowExists() {
cmd := exec.Command("ls", "-h")
stdout, _ := cmd.StdoutPipe()
cmd.Start()
reader := bufio.NewReader(stdout)
for {
line, err := reader.ReadString('\n')
line = strings.TrimSpace(line)
if err != nil || io.EOF == err {
break
}
log.Println(line)
}
cmd.Wait()
}
Go执行shell命令
原创LecKey ©著作权
文章标签 Go执行shell命令 shell命令 Go shell命令 GoLang执行shell命令 执行shell命令 文章分类 JavaScript 前端开发
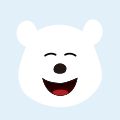
-
Shell概述、编写及执行脚本、Shell变量
shell基础
Shell bash vim -
IOS 执行shell命令 shell如何执行命令
1.shell的作用Shell的作用——命令解释器,“翻译官”介于系统内核与用户之间,负责解释命令行用户的登录shell登录后默认使用的shell程序,一般为/bin/bash不同shell的内部指令、运行环境等会有所区别2.编写shell脚本编写脚本代码使用vi文本编辑器每行一条Linux命令,按执行顺序依次编写赋予可执行权限:chmod +x test.sh使脚本具有可执行
IOS 执行shell命令 shell基础 shell脚本执行时间 shell脚本执行方法 shell作用 -
java 执行 shell命令 java执行shell命令失败
java 执行 shell命令 java执行shell命令失败
sql System 解决方案