#include <stdio.h>
#define MAXVALUE 1000
#define MAXLEAF 30
#define MAXNODE 60
#define MAXBIT 10
typedef struct {
int bit[MAXBIT];
int start;
} HCodeType;
typedef struct {
char data;
int weight;
int parent;
int lchild;
int rchild;
} HNodeType;
//创建哈夫曼树
void HuffmanTree(HNodeType HuffNode[], int n);
//创建哈夫曼编码表
void HuffmanCode(HNodeType HuffNode[], HCodeType HuffCode[], int n);
//对给定字符串进行哈夫曼编码
void enCodeing(HNodeType HuffNode[], HCodeType HuffCode[], int n, char encode[], char str[]);
//对收到的编码进行解码
void deCodeing(HNodeType HuffNode[], int n, char encode[], char decode[]);
void HuffmanTree(HNodeType HuffNode[], int n) {
int i, j, m1, m2, x1, x2;
for (i = 0; i < n - 1; i++) {
m1 = m2 = MAXVALUE;
x1 = x2 = 0;
for (j = 0; j < n + i; j++) {
if (HuffNode[j].weight < m1 && HuffNode[j].parent == -1) {
m2 = m1;
x2 = x1;
m1 = HuffNode[j].weight;
x1 = j;
} else if (HuffNode[j].weight < m2 && HuffNode[j].parent == -1) {
m2 = HuffNode[j].weight;
x2 = j;
}
}
HuffNode[x1].parent = n + i;
HuffNode[x2].parent = n + i;
HuffNode[n + i].weight = HuffNode[x1].weight + HuffNode[x2].weight;
HuffNode[n + i].lchild = x1;
HuffNode[n + i].rchild = x2;
}
}
void HuffmanCode(HNodeType HuffNode[], HCodeType HuffCode[], int n) {
HCodeType cd;
int i, j, c, p;
for (i = 0; i < n; i++) {
cd.start = n - 1;
c = i;
p = HuffNode[i].parent;
while (p != -1) {
if (HuffNode[p].lchild == c)
cd.bit[cd.start] = 0;
else
cd.bit[cd.start] = 1;
cd.start--;
c = p;
p = HuffNode[p].parent;
}
for (j = cd.start + 1; j < n - 1; j++)
HuffCode[i].bit[j] = cd.bit[j];
HuffCode[i].start = cd.start;
}
}
void enCodeing(HNodeType HuffNode[], HCodeType HuffCode[], int n, char encode[], char str[]) {
int i, j, k;
i = 0;
while (str[i] != '\0') {
i++;
}
int len = i;
k = 0;
for (i = 0; i < len; i++) {
for (j = 0; j < n; j++) {
if (str[i] == HuffNode[j].data) {
for (int b = HuffCode[j].start + 1; b < n - 1; b++) {
encode[k++] = '0' + HuffCode[j].bit[b];
}
break;
}
}
}
encode[k] = '\0';
}
void deCodeing(HNodeType HuffNode[], int n, char encode[], char decode[]) {
int i, j, p;
p = 2 * n - 2;
i = 0;
while (encode[i] != '\0') {
i++;
}
int len = i;
for (i = 0; i < len; i++) {
if (encode[i] == '0') {
p = HuffNode[p].lchild;
} else if (encode[i] == '1') {
p = HuffNode[p].rchild;
}
if (HuffNode[p].lchild == -1 && HuffNode[p].rchild == -1) {
decode[i] = HuffNode[p].data;
p = 2 * n - 2;
}
}
decode[i] = '\0';
}
int main() {
HNodeType HuffNode[MAXNODE];
HCodeType HuffCode[MAXLEAF];
int n, i, m;
char encode[MAXVALUE] = {'\0'}, decode[MAXVALUE] = {'\0'}, str[MAXVALUE];
scanf("%d", &n);
getchar();
for (i = 0; i < 2 * n - 1; i++) {
HuffNode[i].data = '0';
HuffNode[i].weight = 0;
HuffNode[i].parent = -1;
HuffNode[i].lchild = -1;
HuffNode[i].rchild = -1;
}
for (i = 0; i < n; i++) {
scanf("%c,%d", &HuffNode[i].data, &HuffNode[i].weight);
getchar();
}
HuffmanTree(HuffNode, n);
HuffmanCode(HuffNode, HuffCode, n);
scanf("%d", &m);
getchar();
for (i = 0; i < m; i++) {
gets(str);
enCodeing(HuffNode, HuffCode, n, encode, str);
puts(encode);
}
scanf("%d", &m);
getchar();
for (i = 0; i < m; i++) {
gets(str);
deCodeing(HuffNode, n, str, decode);
puts(decode);
}
return 0;
}
哈希
原创
©著作权归作者所有:来自51CTO博客作者wx641ed5d0bdd07的原创作品,请联系作者获取转载授权,否则将追究法律责任
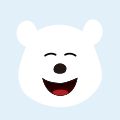
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【LeetCode】705. 设计哈希集合
【LeetCode】705. 设计哈希集合
leetcode 数据结构与算法 C++ -
【LeetCode】706. 设计哈希映射
【LeetCode】706. 设计哈希映射
leetcode 数据结构与算法 C++