Android-数据存储(ShraedPreference)
原创
©著作权归作者所有:来自51CTO博客作者原小明呢的原创作品,请联系作者获取转载授权,否则将追究法律责任
1.回顾
上篇学习了 三种菜单的使用,OptionMenu , subMenu , ContextMenu 的 动态添加和 静态导入,其中ContextMenu使用的时候,记住 给需要的 View 注册 就行了;
前前篇学习了Notification的实现,实现很简单,但是用在实际项目中,还需要考虑,注意添加震动权限和闪光灯权限即可;
到此为止:
学习了 6个Adapter :SimapleAdapter ,BaseAdapter ,ArrayAdapter ,PagerAdapter ,FragmentAdapter 和FragmentStateAdapter的使用,总的来说 其实就两个 一个BaseAdapter 和 PagerAdapter ;
学习了2个Builder : AlertDialog.builder 和 Notification.builder 两个 builder ;
今天将学习了 一个 Editer !
2.重点
(1)Sharedference 的存储
(2)Sharedference 的读取
(3)做一个例子,将用户登录
3.Sharedference 存储数据
(1)得到Sharedference 对象
SharedPreferences spre = getSharedPreferences("login", 1);
(2)通过Editer 实现存储数据
最后一定要commit()
Editor editor= spre.edit();
editor.putString("name",editText1.getText().toString());
editor.commit();
4.Sharedference 读取数据
直接可以读取
//读取sharedPreferences
String name=spre.getString("name","");
if(name.equals("")||name!=null){
editText1.setText(name);
}
5.一个登陆的例子
5.1 效果图
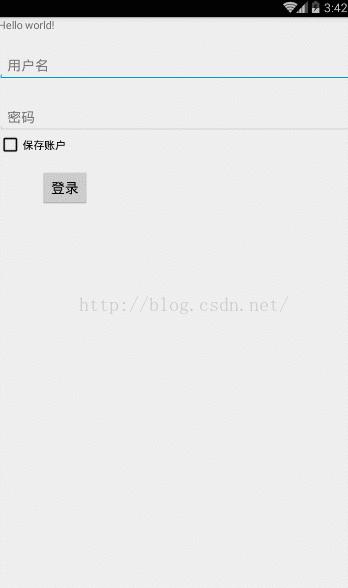
5.2 布局实现
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:cnotallow="${relativePackage}.${activityClass}" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true"
android:hint="用户名"
android:layout_below="@+id/textView1"
android:layout_marginTop="24dp"
android:ems="10" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/editText2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true"
android:layout_below="@+id/editText1"
android:layout_marginTop="28dp"
android:hint="密码"
android:ems="10" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/editText2"
android:layout_marginLeft="55dp"
android:layout_marginTop="48dp"
android:text="登录" />
<CheckBox
android:id="@+id/checkBox1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/editText2"
android:checked="false"
android:text="保存账户" />
</RelativeLayout>
5.3 业务实现
package com.example.studydemo9;
import android.app.Activity;
import android.content.SharedPreferences;
import android.content.SharedPreferences.Editor;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
private EditText editText1, editText2;
private Button btn_login;
private CheckBox checkBox1;
private SharedPreferences spre;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
spre = getSharedPreferences("login", 1);
checkBox1=(CheckBox) findViewById(R.id.checkBox1);
editText1 = (EditText) findViewById(R.id.editText1);
editText2 = (EditText) findViewById(R.id.editText2);
btn_login=(Button) findViewById(R.id.button1);
btn_login.setOnClickListener(new btn_loginListener());
//读取sharedPreferences
String name=spre.getString("name","");
if(name.equals("")||name!=null){
editText1.setText(name);
}
}
/**
* 登陆点击事件
*
* @author yuan
*
*/
class btn_loginListener implements OnClickListener {
@Override
public void onClick(View v) {
//点击事件
if(editText1.getText().toString().equals("yuan")&&editText2.getText().toString().equals("ming")){
//存账户
if(checkBox1.isChecked()){
Editor editor= spre.edit();
editor.putString("name",editText1.getText().toString());
editor.commit();
}
Toast.makeText(MainActivity.this,"登陆成功",Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(MainActivity.this,"登陆失败",Toast.LENGTH_SHORT).show();
}
}
}
}