#include <iostream>
#include <string>
#include <vector>
#include <cassert>
#include <typeinfo>
#include <algorithm>
#include <boost/any.hpp>
typedef boost::any cell_t;
typedef std::vector<cell_t> db_row_t;
db_row_t get_row(const char *) {
db_row_t row;
row.push_back(10);
row.push_back(10.1f);
row.push_back(std::string("hello world!"));
return row;
}
struct db_sum {
public:
explicit db_sum(double &sum) : sum_(sum) {
}
void operator () (const cell_t &value) {
const std::type_info &ti = value.type();
if (typeid(int) == ti) {
sum_ += boost::any_cast<int>(value);
}
else if (typeid(float) == ti) {
sum_ += boost::any_cast<float>(value);
}
}
private:
double &sum_;
};
int main() {
db_row_t row = get_row("");
double res = 0.0;
std::for_each(begin(row), end(row), db_sum(res));
std::cout << res << std::endl;
return 0;
}
函数对象好例子
原创
©著作权归作者所有:来自51CTO博客作者我是006的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:mysql脚本在执行过程中出现“ERROR 23 (HY000) at line 29963: Out of resources when opening file...”
下一篇:查看mysql某张表分区信息
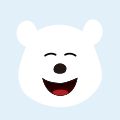
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
git博客好的例子
01: https://github.com/Gaohaoyang/gaohaoyang.github.io 02: https://gaohaoyang.github.io/2018/06/01/animation/ 03:
github 机器学习 嵌入式 .net javascript -
python 函数 例子
一些常用的内置函数经常会忘记,方便自己回溯用法下列内置函数基于Python3all()和any()参数为iterable,即元组或列表all()函数用于判断iterable中的所有元素是否都为TRUE,元素除了是 0、空、None、False 外都算 True。any()函数用于判断iterable中的所有元素是否都为False。 容易混淆,而且有坑,不清楚不建议使用 bool()元素为
python 函数 例子 function函数的用法 python eval函数用法 python中find函数的用法 python中input函数的用法 -
pem内容有换行符
1.pb9应用xp风格 将PB9升级到7119后,编译时有一个 New Visual Style Controls 选项,选中后,编译出来的文件在XP下就可以应用XP风格了 2.Yield()函数的作用 Yield()是一个不常用到的PowerBuilder函数。可是,在一个大的循环过程中,如果用户想在执行到一半时通过单击按钮或菜单来退出的话,
pem内容有换行符 powerbuilder function library string