/*************************************************************************
> File Name: AtomicInteger.h
> Author: wangzhicheng
> Created Time: 2017-02-20
> statement: provide atomic integer and operation for multithread
************************************************************************/
#ifndef _ATOMIC_INTEGER_H_
#define _ATMOIC_INTEGER_H_
#include <stdio.h>
#include <stdint.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <pthread.h>
#include <iostream>
using namespace std;
/**
*@brief provide atomic integer and operation for multithread
*/
template<class T>
class AtomicInteger {
private:
volatile T value; // prevent g++ to optimize the value
public:
AtomicInteger():value(0) {
}
/*
*@brief get the original value
* */
T GetValue() {
// if value == 0 then value = 0 and return the original value
return __sync_val_compare_and_swap(&value, 0, 0);
}
/*
*@brief suffix ++ such as a++
* */
T operator ++ (int) {
return __sync_fetch_and_add(&value, 1);
}
/*
*@brief prefix ++ such as ++a
* */
T operator ++ () {
return __sync_add_and_fetch(&value, 1);
}
/*
*@brief 加某个数
* */
T operator + (int num) {
return __sync_add_and_fetch(&value, num);
}
/*
*@brief 减某个数
* */
T operator - (int num) {
return __sync_add_and_fetch(&value, -num);
}
/*
*@brief suffix -- such as a--
* */
T operator -- (int) {
return __sync_fetch_and_add(&value, -1);
}
/*
*@brief prefix -- such as --a
* */
T operator -- () {
return __sync_add_and_fetch(&value, -1);
}
/*
*@brief add operation
* */
T operator + (const T &other) {
return __sync_add_and_fetch(&value, other);
}
/*
*@brief = operation
* */
T operator = (const T &newValue) {
return __sync_lock_test_and_set(&value, newValue);
}
};//ns
typedef AtomicInteger<uint64_t>AtomicInt64;
typedef AtomicInteger<uint32_t>AtomicInt32;
#endif
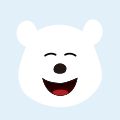
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
原子类 Atomic
原子类 atomic
原子类atomic 返回结果 默认值 原子类 -
原子操作-atomic
一、原子操作简介 所谓的原子操作,取的就是“原子是最小的、不可分割的最小个体”的意义,它表示在多个线程访问同
原子操作 多线程 #include 无锁队列