using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace simulationQQ
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//public event copyToFatherTextBox copytoFather; //截屏完毕后交个父窗体处理截图
public bool begin = false; //是否开始截屏
public bool isDoubleClick = false;
public Point firstPoint = new Point(0, 0); //鼠标第一点
public Point secondPoint = new Point(0, 0); //鼠标第二点
public Image cachImage = null; //用来缓存截获的屏幕
public int halfWidth = 0;//保存屏幕一半的宽度
public int halfHeight = 0;//保存屏幕一般的高度
/*复制整个屏幕,并让窗体填充屏幕*/
public void copyScreen()
{
Rectangle r = Screen.PrimaryScreen.Bounds;
Image img = new Bitmap(r.Width, r.Height);
Graphics g = Graphics.FromImage(img);
g.CopyFromScreen(new Point(0, 0), new Point(0, 0), r.Size);
//窗体最大化,及相关处理
this.Width = r.Width;
this.Height = r.Height;
this.Left = 0;
this.Top = 0;
pictureBox1.Width = r.Width;
pictureBox1.Height = r.Height;
pictureBox1.BackgroundImage = img;
cachImage = img;
halfWidth = r.Width / 2;
halfHeight = r.Height / 2;
//this.Cursor = new Cursor(GetType(), "MyCursor.cur");
}
/*鼠标按下时开始截图*/
private void pictureBox1_MouseDown(object sender, MouseEventArgs e)
{
if(!isDoubleClick)
{
begin = true;
firstPoint = new Point(e.X, e.Y);
changePoint(e.X, e.Y);
msg.Visible = true;
}
}
/*鼠标移动时显示截取区域的边框*/
private void pictureBox1_MouseMove(object sender, MouseEventArgs e)
{
if (begin)
{
//获取新的右下角坐标
secondPoint = new Point(e.X, e.Y);
int minX = Math.Min(firstPoint.X, secondPoint.X);
int minY = Math.Min(firstPoint.Y, secondPoint.Y);
int maxX = Math.Max(firstPoint.X, secondPoint.X);
int maxY = Math.Max(firstPoint.Y, secondPoint.Y);
//重新画背景图
Image tempimage = new Bitmap(cachImage);
Graphics g = Graphics.FromImage(tempimage);
//画裁剪框
g.DrawRectangle(new Pen(Color.Red),minX,minY,maxX-minX,maxY-minY);
pictureBox1.Image = tempimage;
//计算坐标信息
msg.Text = "左上角坐标:(" + minX.ToString() + "," + minY.ToString() + ")/r/n";
msg.Text += "右下角坐标:(" + maxX.ToString() + "," + maxY.ToString() + ")/r/n";
msg.Text += "截图大小:" + (maxX - minX) + "×" + (maxY - minY) + "/r/n";
msg.Text += "双击任意地方结束截屏!";
changePoint((minX + maxX) / 2, (minY + maxY) / 2);
}
}
/*动态调整显示信息的位置,输入参数为当前截屏鼠标位置*/
public void changePoint(int x, int y)
{
if (x < halfWidth)
{
if (y < halfHeight)
{ msg.Top = halfHeight; msg.Left = halfWidth; }
else
{ msg.Top = 0; msg.Left = halfWidth; }
}
else
{
if (y < halfHeight)
{ msg.Top = halfHeight; msg.Left = 0; }
else
{ msg.Top = 0; msg.Left = 0; }
}
}
/*鼠标放开时截图操作完成*/
private void pictureBox1_MouseUp(object sender, MouseEventArgs e)
{
begin = false;
isDoubleClick = true; //之后再点击就是双击事件了
}
/*双击时截图时,通知父窗体完成截图操作,同时关闭本窗体*/
private void pictureBox1_DoubleClick(object sender, EventArgs e)
{
if (firstPoint != secondPoint)
{
int minX = Math.Min(firstPoint.X, secondPoint.X);
int minY = Math.Min(firstPoint.Y, secondPoint.Y);
int maxX = Math.Max(firstPoint.X, secondPoint.X);
int maxY = Math.Max(firstPoint.Y, secondPoint.Y);
Rectangle r = new Rectangle(minX, minY, maxX - minX, maxY - minY);
//copytoFather(r);
}
this.Close();
//msg.Text = r.ToString();
}
private void Form1_Load(object sender, EventArgs e)
{
copyScreen();
}
}
}
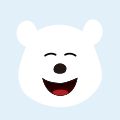
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C# 简单实现QQ截图功能
接上一篇写的截取电脑屏幕,我们在原来的基础上加一个选择区域的功能,实现自定义选择截图。个人比较懒,上一篇的代
sed desktop 缩放比例 -
C# 屏幕截图
C# 屏幕截图
C# 屏幕截图 System Windows Linq -
c# 图片截图
using System;using System.Collections.Generic;using System.ComponentModel;using System.Data;u
c# c# 图片截图 redis 缩放比例 ico -
C# 桌面截图工具 demo
自动截屏 并保存在 某个目录下。截图:工具下载地址:http://pan.baid
下载地址 .net 源代码下载