4.3tensorflow2.0实战梯度下降求空间曲面的最低点
原创
©著作权归作者所有:来自51CTO博客作者小怪兽会微笑的原创作品,请联系作者获取转载授权,否则将追究法律责任
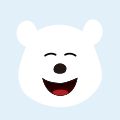
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
基于Python和TensorFlow实现BERT模型应用
在本文中,我们详细介绍了BERT模型的基本原理,并使用Python和TensorFlow实现了一个简单的BERT分类模型。
Python tensorflow 加载 Transformer Bert -
Tensorflow2(预课程)---1.4.1、自动计算梯度Tensorflow2(预课程) tensorflow 微信 人工智能 大数据