【精通Java】集合类体系之Collection
原创
©著作权归作者所有:来自51CTO博客作者江岸畔的小鱼的原创作品,请联系作者获取转载授权,否则将追究法律责任


个人名片:
🐼作者简介:一名大一在校生 🐻❄️个人主页:小新爱学习.
🕊️系列专栏:零基础学java ----- 重识c语言
🐓每日一句:“即使迄今为止所有的人生都大写着失败,但不妨碍我继续向前”
文章目录
- 1.1 集合知识回顾🦋
- 1.2 集合类体系结构🐣
- 1.3 Collection 集合的概述和使用🦀
- 1.4 Collection 集合常用方法🐬
- 1.5 Collection 集合的遍历🐷
- 1.6 集合的使用步骤🐯
集合🎉
1. Collection✨
1.1 集合知识回顾🦋
集合类的特点:提供一种储存空间可变的储存模型,存储的数据结构随地发生改变
1.2 集合类体系结构🐣

1.3 Collection 集合的概述和使用🦀

Collection 层次结构 中的根接口。Collection 表示一组对象,这些对象也称为 collection 的元素。一些 collection 允许有重复的元素,而另一些则不允许。一些 collection 是有序的,而另一些则是无序的。JDK 不提供此接口的任何直接 实现:它提供更具体的子接口(如 Set 和 List)实现。此接口通常用来传递 collection,并在需要最大普遍性的地方操作这些 collection。
Collection 集合的概述
- Collection 表示一组对象,这些对象也称为 collection 的元素。
- JDK 不提供此接口的任何直接 实现:它提供更具体的子接口(如 Set 和 List)实现。
创建Collection 集合对象
import java.util.ArrayList;
import java.util.Collection;
/*
创建Collection 集合对象
- 多态的形式
- 具体的实现类ArrayList
*/
public class CollectionDemo01 {
public static void main(String[] args) {
//创建collection对象、
Collection<String> c = new ArrayList<String>();
//添加元素: boolean add(E e)
c.add("hmm");
c.add("20");
c.add("大一");
//输出集合对象
System.out.println(c);//重写了to String方法
}
}

1.4 Collection 集合常用方法🐬
方法名
| 说明
|
boolean add(E e)
| 确保此 collection 包含指定的元素(可选操作)。
|
boolean remove(Object o)
| 从此 collection 中移除指定元素的单个实例,如果存在的话(可选操作)。
|
void clear()
| 移除此 collection 中的所有元素(可选操作)。
|
boolean contains(Object o)
| 如果此 collection 包含指定的元素,则返回 true。
|
boolean isEmpty()
| 如果此 collection 不包含元素,则返回 true。
|
int size()
| 返回此 collection 中的元素数。 集合长度
|
import java.util.ArrayList;
import java.util.Collection;
//boolean add(E e) |确保此 collection 包含指定的元素(可选操作)。
// boolean remove(Object o) |从此 collection 中移除指定元素的单个实例,如果存在的话(可选操作)。
// void clear() |移除此 collection 中的所有元素(可选操作)。
// boolean contains(Object o) |如果此 collection 包含指定的元素,则返回 true。
// boolean isEmpty() |如果此 collection 不包含元素,则返回 true。
// int size() |返回此 collection 中的元素数。 集合长度
public class CollectionDemo02 {
public static void main(String[] args) {
//创建集合对象
Collection<String> c = new ArrayList<String>();
//boolean add(E e) |确保此 collection 包含指定的元素(可选操作)。
// System.out.println(c.add("hmm"));
// System.out.println(c.add("20"));
// System.out.println(c.add("大一"));
//调用c.add 方法返回都是true
c.add("hello");
c.add("world");
c.add("java");
// boolean remove(Object o) |从此 collection 中移除指定元素的单个实例,如果存在的话(可选操作)。
//System.out.println(c.remove("world"));
// void clear() |移除此 collection 中的所有元素(可选操作)。
// c.clear();
// boolean contains(Object o) |如果此 collection 包含指定的元素,则返回 true。
//System.out.println(c.contains("world"));
//System.out.println(c.contains("hmm"));
// boolean isEmpty() |如果此 collection 不包含元素,则返回 true。
System.out.println(c.isEmpty());
int size() |返回此 collection 中的元素数。 集合长度
System.out.println(c.size());
//输出集合对象
System.out.println(c);
}
}
1.5 Collection 集合的遍历🐷
Iterator:迭代器,集合的专用遍历方法
public interface Iterator
对 collection 进行迭代的迭代器。迭代器取代了 Java Collections Framework 中的 Enumeration。迭代器与枚举有两点不同:
迭代器允许调用者利用定义良好的语义在迭代期间从迭代器所指向的
- collection 移除元素。
- 方法名称得到了改进。
此接口是 Java Collections Framework 的成员。
Inerator中的常用方法
- boolean hasNext() 如果仍有元素可以迭代,则返回 true。
- E next() 返回迭代的下一个元素。
- void remove() 从迭代器指向的 collection 中移除迭代器返回的最后一个元素(可选操作)。
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
// - boolean hasNext() 如果仍有元素可以迭代,则返回 true。
// - E next() 返回迭代的下一个元素。
//- void remove() 从迭代器指向的 collection 中移除迭代器返回的最后一个元素(可选操作)。
public class CollectionDemo03 {
public static void main(String[] args) {
//创建集合对象
Collection<String> c = new ArrayList<String>();
//添加集合元素
c.add("hello");
c.add("world");
c.add("java");
//public interface Iterator<E>对 collection进行迭代的迭代器。
Iterator<String> it = c.iterator();
/*
public Iterator<E> iterator() {
return new ArrayList.Itr();
private class Itr implements Iterator<E> {
...
}
*/
// - E next() 返回迭代的下一个元素。
// System.out.println(it.next());
// System.out.println(it.next());
// System.out.println(it.next());
// System.out.println(it.next());//.NoSuchElementException由 Enumeration 的 nextElement 方法抛出,表明枚举中没有更多的元素。
// - boolean hasNext() 如果仍有元素可以迭代,则返回 true。
// if(it.hasNext()){
// System.out.println(it.next());
// }
//用while循环改进
while(it.hasNext()){
System.out.println(it.next());
String s = it.next();
System.out.println(s);
}
//输出集合
//System.out.println(c);
}
}
1.6 集合的使用步骤🐯
1. 步骤1: 创建集合对象2. 步骤2:添加元素
步骤2.1:创建元素
步骤2.2:添加元素到集合 3. 步骤3:遍历集合
3.1:通过集合对象获取迭代器对象
3.2:通过迭代器对象的hasNext()方法判断是否还有元素
3.3:通过迭代器对象的next()方法获取下一个元素
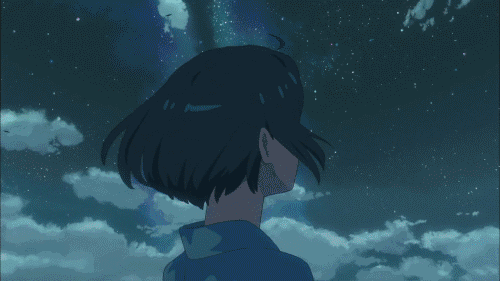