1.引入element-ui upload组件
<el-upload class="avatar-uploader" :headers="myHeaders" :action="上传图片的地址" :show-file-list="false" :on-success="handleAvatarSuccess" :before-upload="beforeAvatarUpload">
<img v-if="imageUrl" :src="imageUrl" class="avatar" />
<i v-else class="el-icon-plus avatar-uploader-icon"></i>
</el-upload>
2.在style中 定义el-upload的样式
<style>
.avatar-uploader .el-upload {
border: 1px dashed #d9d9d9;
border-radius: 6px;
cursor: pointer;
position: relative;
overflow: hidden;
}
.avatar-uploader .el-upload:hover {
border-color: #409EFF;
}
.avatar-uploader-icon {
font-size: 28px;
color: #8c939d;
width: 178px;
height: 178px;
line-height: 178px;
text-align: center;
}
.avatar {
width: 178px;
height: 178px;
display: block;
}
</style>
3.在methods里 定义限制图片的方法
methods:{
//图片上传成功的回调函数
handleAvatarSuccess(res, file) {
if (file.raw.isFlag && res.code == 0) {
this.imageUrl = URL.createObjectURL(file.raw);
this.newBanner_Form.imgUrl = res.data.url;
}
},
//图片上传前的回调函数
beforeAvatarUpload(file) {
const isJPG = file.type === "image/jpeg" || file.type === "image/png";
if (!isJPG) {
this.$message.error("上传头像图片只能是 JPG和PNG 格式!");
}
//调用[限制图片尺寸]函数
this.limitFileWH(702, 285, file).then((res) => {
file.isFlag = res
})
return isJPG && file.isFlag;
},
//限制图片尺寸
limitFileWH(E_width, E_height, file) {
let _this = this;
let imgWidth = "";
let imgHight = "";
const isSize = new Promise(function(resolve, reject) {
let width = E_width;
let height = E_height;
let _URL = window.URL || window.webkitURL;
let img = new Image();
img.onload = function() {
imgWidth = img.width;
imgHight = img.height;
let valid = img.width == width && img.height == height;
valid ? resolve() : reject();
}
img.src = _URL.createObjectURL(file);
}).then(() => {
return true;
}, () => {
_this.$message.warning({
message: '上传文件的图片大小不合符标准,宽需要为' + E_width + 'px,高需要为' + E_height + 'px。当前上传图片的宽高分别为:' + imgWidth + 'px和' +
imgHight + 'px',
btn: false
})
return false;
});
return isSize
},
}
4.解决before-upload钩子返回false时,文件仍然上传成功的问题
如果我们在before-upload中直接返回true或者是false,那么它其实也是会上传文件的,因为它也会触发on-change函数。
我这里是采用在对应的函数中返回一个promise来解决的,就像下面这样:
beforeAvatarUpload(file) {
return new Promise((resolve,reject) => {
const isJPG = file.type === 'image/jpeg' || file.type === 'image/png';
if (!isJPG) {
this.$message.error('上传头像图片只能是 JPG和PNG 格式!');
}
//调用[限制图片尺寸]函数
this.limitFileWH(702, 285, file).then((res) => {
file.isFlag = res;
});
if (file.isFlag) {
return resolve(true);
} else {
return reject(false);
}
})
},
Vue+Element ui上传图片限制图片尺寸
转载上一篇:uni-app的一些方法
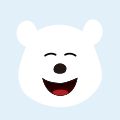
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
图片尺寸判断等
我们到底能走多远系列(21)扯淡:又是一年过年,给各位拜个很早很早的年,实在点的祝福:祝各位明年工资全部翻一番啦
json javascript ViewUI 上传 ajax -
MarkDown 简书上传图片尺寸设置
刚在简书上写了一篇文章,发现与和GitHub的格式有点不一样,特在此总结出来,方便其他和我当初一样的吃瓜群众了解真相。
markdown 简书 3c github