package com.safein.util;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import org.apache.log4j.Logger;
/**
* 公共配置文件读取操作类
* @author julong
* @date 2016年5月10日 上午9:35:40
*/
public class MessageUtil {
//日志
private static final Logger logger = Logger.getLogger(MessageUtil.class);
//当前对象
private static MessageUtil instance = null;
private Properties properties = null;
/**
* 配置文件
* @author julong
* @date 2016-7-25 上午11:46:27
*/
private static final String configFile = "message.properties";
/**
* 单例化对象
* @return
* @author julong
* @date 2016年5月11日 上午11:50:48
*/
public static synchronized MessageUtil getInstance() {
if (null == instance) {
instance = new MessageUtil();
}
return instance;
}
/**
* 私有化构造函数
* @author julong
* @date 2016年5月11日 上午11:50:26
*/
private MessageUtil() {
logger.debug("Init the config properties...");
properties = new Properties();
InputStream is = null;
try {
is = this.getClass().getClassLoader().getResourceAsStream(configFile);
properties.load(is);
} catch (IOException e) {
throw new RuntimeException("返回码配置文件读取失败");
} finally {
if (null != is) {
try {
is.close();
} catch (IOException e) {
throw new RuntimeException("关闭返回码配置文件读取流失败");
}
}
}
logger.debug("Finish read config properties.");
}
/**
* 获取当前是否存在键值对
* @param key
* @return
* @author julong
* @date 2016年5月10日 上午9:39:37
*/
public boolean containsKey(String key) {
return properties.containsKey(key);
}
/**
* 获取key对应的value
* @param retCode
* @return
* @author julong
* @date 2016年5月10日 上午9:40:11
*/
public String getProperty(String key) {
return properties.getProperty(key);
}
public static void main(String[] args) {
// TODO Auto-generated method stub
MessageUtil configProperties = new MessageUtil();
boolean result = configProperties.containsKey("000000");
System.out.println(result);
String value = configProperties.getProperty("000000");
System.out.println(value);
}
}
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import org.apache.log4j.Logger;
/**
* 公共配置文件读取操作类
* @author julong
* @date 2016年5月10日 上午9:35:40
*/
public class MessageUtil {
//日志
private static final Logger logger = Logger.getLogger(MessageUtil.class);
//当前对象
private static MessageUtil instance = null;
private Properties properties = null;
/**
* 配置文件
* @author julong
* @date 2016-7-25 上午11:46:27
*/
private static final String configFile = "message.properties";
/**
* 单例化对象
* @return
* @author julong
* @date 2016年5月11日 上午11:50:48
*/
public static synchronized MessageUtil getInstance() {
if (null == instance) {
instance = new MessageUtil();
}
return instance;
}
/**
* 私有化构造函数
* @author julong
* @date 2016年5月11日 上午11:50:26
*/
private MessageUtil() {
logger.debug("Init the config properties...");
properties = new Properties();
InputStream is = null;
try {
is = this.getClass().getClassLoader().getResourceAsStream(configFile);
properties.load(is);
} catch (IOException e) {
throw new RuntimeException("返回码配置文件读取失败");
} finally {
if (null != is) {
try {
is.close();
} catch (IOException e) {
throw new RuntimeException("关闭返回码配置文件读取流失败");
}
}
}
logger.debug("Finish read config properties.");
}
/**
* 获取当前是否存在键值对
* @param key
* @return
* @author julong
* @date 2016年5月10日 上午9:39:37
*/
public boolean containsKey(String key) {
return properties.containsKey(key);
}
/**
* 获取key对应的value
* @param retCode
* @return
* @author julong
* @date 2016年5月10日 上午9:40:11
*/
public String getProperty(String key) {
return properties.getProperty(key);
}
public static void main(String[] args) {
// TODO Auto-generated method stub
MessageUtil configProperties = new MessageUtil();
boolean result = configProperties.containsKey("000000");
System.out.println(result);
String value = configProperties.getProperty("000000");
System.out.println(value);
}
}
java读取配置文件工具类
原创
©著作权归作者所有:来自51CTO博客作者口袋里的小龙的原创作品,请联系作者获取转载授权,否则将追究法律责任
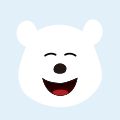
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
MySQL8.4.3-LTS版配置文件参数
MySQL8.4.3-my.cnf配置文件参数
mysql 默认值 ci -
springboot学习三:Spring Boot 配置文件语法、静态工具类读取配置文件、静态工具类读取配置文件
springboot学习三:Spring Boot 配置文件语法、静态工具类读取配置文件、profile多环境配置、devtools热部署
spring boot 学习 spring 配置文件 List -
Spring 读取配置 spring读取配置文件工具类
一、背景 我们都知道spring可以帮我们读取properties配置文件,我们只需要简单配置一行:<context:property-placeholder location="classpath:properties/*.properties" file-encoding="UTF-8"/>就能在java代码中使用@Value("${xxxx}")美滋滋的读取配置文件,但是如果有需
Spring 读取配置 propertiesutil PropertiesUtil java读取配置文件 java properties