package com.julongtech.test;
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class ZipTest {
public static void main(String[] args) {
// TODO Auto-generated method stub
ZipTest zipTest = new ZipTest();
try {
//http://apache.fayea.com/commons/fileupload/binaries/commons-fileupload-1.3.1-bin.zip
zipTest.downloadFile("http://apache.fayea.com/commons/fileupload/binaries/commons-fileupload-1.3.1-bin.zip","C:/commons-fileupload-1.3.1.zip");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/**
* 文件下载的方法
* @param urls
* @param fileName
* @author julong
* @date 2016年5月9日 下午10:30:46
*/
public void downloadFile(String urls, String fileName){
//定义文件流
FileOutputStream fileOutputStream = null;
//定义输出流
BufferedInputStream bufferedInputStream = null;
//定义网络连接
HttpURLConnection httpURLConnection = null;
URL url = null;
try {
byte[] buf = new byte[1024];
int size = 0;
//创建一个URL对象
url = new URL(urls);
//打开网络连接
httpURLConnection = (HttpURLConnection) url.openConnection();
//连接指定的资源
httpURLConnection.connect();
//获取网络输入流
bufferedInputStream = new BufferedInputStream(httpURLConnection.getInputStream());
//创建文件输出流
fileOutputStream = new FileOutputStream(fileName);
//读取文件流
while ((size = bufferedInputStream.read(buf)) != -1){
fileOutputStream.write(buf, 0, size);
}
fileOutputStream.close();
bufferedInputStream.close();
httpURLConnection.disconnect();
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
}finally{
try {
if(null !=fileOutputStream){
fileOutputStream.close();
}
if(null != bufferedInputStream){
bufferedInputStream.close();
}
if(null != httpURLConnection){
httpURLConnection.disconnect();
}
} catch (Exception e2) {
}
}
}
}
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class ZipTest {
public static void main(String[] args) {
// TODO Auto-generated method stub
ZipTest zipTest = new ZipTest();
try {
//http://apache.fayea.com/commons/fileupload/binaries/commons-fileupload-1.3.1-bin.zip
zipTest.downloadFile("http://apache.fayea.com/commons/fileupload/binaries/commons-fileupload-1.3.1-bin.zip","C:/commons-fileupload-1.3.1.zip");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/**
* 文件下载的方法
* @param urls
* @param fileName
* @author julong
* @date 2016年5月9日 下午10:30:46
*/
public void downloadFile(String urls, String fileName){
//定义文件流
FileOutputStream fileOutputStream = null;
//定义输出流
BufferedInputStream bufferedInputStream = null;
//定义网络连接
HttpURLConnection httpURLConnection = null;
URL url = null;
try {
byte[] buf = new byte[1024];
int size = 0;
//创建一个URL对象
url = new URL(urls);
//打开网络连接
httpURLConnection = (HttpURLConnection) url.openConnection();
//连接指定的资源
httpURLConnection.connect();
//获取网络输入流
bufferedInputStream = new BufferedInputStream(httpURLConnection.getInputStream());
//创建文件输出流
fileOutputStream = new FileOutputStream(fileName);
//读取文件流
while ((size = bufferedInputStream.read(buf)) != -1){
fileOutputStream.write(buf, 0, size);
}
fileOutputStream.close();
bufferedInputStream.close();
httpURLConnection.disconnect();
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
}finally{
try {
if(null !=fileOutputStream){
fileOutputStream.close();
}
if(null != bufferedInputStream){
bufferedInputStream.close();
}
if(null != httpURLConnection){
httpURLConnection.disconnect();
}
} catch (Exception e2) {
}
}
}
}
HTTP网络远程下载文件到本地
原创
©著作权归作者所有:来自51CTO博客作者口袋里的小龙的原创作品,请联系作者获取转载授权,否则将追究法律责任
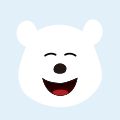
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
centos7用命令上传、下载文件
centos7用命令上传、下载文件
centos linux 上传下载 -
Python HTTP Basic 认证 + 下载文件到本地
简单代码示例im
浏览器 basic Python 下载 代码示例