<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="app">
<ul>
<li><a href="#/">Home</a></li>
<li><a href="#/about">About</a></li>
</ul>
<div id="view"></div>
</div>
<script>
let Home = {
template:'<h2>this is home</h2>'
}
let About = {
template: '<h2>this is about</h2>'
}
let Router = function (el) {
let view = document.getElementById(el);
let routes = [];
let load = function (route) {
route && (view.innerHTML = route.template)
}
let redirect = function () {
let url = window.location.hash.slice(1) || '/'
for(let route of routes){
url === route.url && load(route)
}
}
this.push = function (route) {
routes.push(route);
}
window.addEventListener('load',redirect,false)
window.addEventListener('hashchange',redirect,false)
}
let router = new Router('view');
router.push({
url:'/',
template:Home
});
router.push({
url:'/about',
template:About
});
</script>
</body>
</html>
vue-快速入门第6章02.html
原创虾米大王 ©著作权
文章标签 vue.js html 文章分类 JavaScript 前端开发
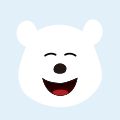
-
vue-快速入门第3章02.html
【代码】vue-快速入门第3章02.html。
vue.js html Vue -
vue-快速入门第2章02.html
【代码】vue-快速入门第2章02.html。
vue.js html Vue -
vue-快速入门第6章04.html
【代码】vue-快速入门第6章04.html。
vue.js html Vue -
vue-快速入门第6章01.html
【代码】vue-快速入门第6章01.html。
vue.js html Vue -
vue-快速入门第6章03.html
【代码】vue-快速入门第6章03.html。
vue.js html Vue -
vue-快速入门第6章05.html
【代码】vue-快速入门第6章05.html。
vue.js javascript 前端 Email html -
vue-案例教程第6章02.html
【代码】vue-案例教程第6章02.html。
vue.js html 原型链 -
vue-快速入门第2章08.html
【代码】vue-快速入门第2章08.html。
vue.js javascript 前端 html Vue -
vue-快速入门第2章09.html
【代码】vue-快速入门第2章09.html。
vue.js javascript 前端 html Vue -
vue-快速入门第2章07.html
【代码】vue-快速入门第2章07.html。
vue.js html Vue -
vue-快速入门第2章03.html
【代码】vue-快速入门第2章03.html。
vue.js html -
vue-快速入门第2章04.html
【代码】vue-快速入门第2章04.html。
html javascript 前端 运算符 函数对象 -
vue-快速入门第2章05.html
【代码】vue-快速入门第2章05.html。
vue.js html Vue -
vue-快速入门第3章01.html
【代码】vue-快速入门第3章01.html。
vue.js javascript 前端 html Vue -
vue-快速入门第2章06.html
【代码】vue-快速入门第2章06.html。
vue.js html Vue -
vue-快速入门第2章01.html
【代码】vue-快速入门第2章01.html。
vue.js html Math -
vue-案例教程第3章02.html
【代码】vue-案例教程第3章02.html。
javascript 前端 html -
理论推导logistic回归公式
先给出Logistic回归的sigmod函数数学表达式: &nbs
理论推导logistic回归公式 迭代 数据 权值