#include <stdio.h>
#include <stdlib.h>
#define true 1
#define false 0
#define bool char
typedef char ElemType;
typedef struct node1
{
ElemType data;
struct node1 *next;
}LinkStackNode1,*LinkStack1;
//typedef LinkStackNode1 *LinkStack1;
typedef struct node2
{
int data;
struct node2 *next;
}LinkStackNode2,*LinkStack2;
//typedef LinkStackNode2 *LinkStack2;
void InitStack1(LinkStack1 *S)
{
*S=(LinkStackNode1 *)malloc(sizeof(LinkStackNode1));
(*S)->next=NULL;
}
void InitStack2(LinkStack2 *S)
{
(*S) = (LinkStackNode2 *)malloc(sizeof(LinkStackNode2));
(*S)->next=NULL;
}
bool Push1(LinkStack1 top,char e)
{
LinkStackNode1 *tmp;
tmp=(LinkStackNode1 *)malloc(sizeof(LinkStackNode1));
if(tmp==NULL)
{
printf("申请空间失败");
return false;
}
tmp->data=e;
tmp->next=top->next;
return true;
}
bool Push2(LinkStack2 top,int e)
{
LinkStackNode2 *tmp;
tmp=(LinkStackNode2 *)malloc(sizeof(LinkStackNode2));
if(tmp==NULL)
{
printf("申请空间失败");
return false;
}
tmp->data=e;
tmp->next=top->next;
return true;
}
bool Pop1(LinkStack1 top,char *e)
{
LinkStackNode1 *tmp;
tmp=top->next;
if(tmp==NULL)
{
printf("栈空error");
return false;
}
*e=tmp->data;
free(tmp);
return true;
}
bool Pop2(LinkStack2 top,int *e)
{
LinkStackNode2 *tmp;
tmp=top->next;
if(tmp==NULL)
{
printf("栈空error");
return false;
}
*e=tmp->data;
free(tmp);
return true;
}
bool In1(char ch,LinkStack1 OPSet)
{
LinkStackNode1 *tmp;
tmp=OPSet->next;
while(tmp!=NULL)
{
if(tmp->data==ch)
{
return true;
}
tmp=tmp->next;
}
return true;
}
char GetTop1(LinkStack1 S)
{
LinkStackNode1 *tmp;
tmp=S->next;
return tmp->data;
}
char Compare(char a,char b)
{
if(b=='*' || b=='/')
{
if(a=='*' || a=='/')
{
return '=';
}
else if(a=='-' || a=='+')
{
return '<';
}
}
else if(b=='+' || b=='-')
{
if(a=='+' || b=='-')
{
return '=';
}
else if(a=='*' || a=='/')
{
return '>';
}
}
}
int Execute(int a,char op,int b)
{
printf("未完成");
if(op=='+')
{
return a+b;
}
else if(op=='-')
{
return a-b;
}
else if(op=='*')
{
return a*b;
}
else if(op=='/')
{
return a/b;
}
else
{
printf("??");
}
}
int ExpEvaluation()
{
LinkStack1 OPTR,OPSet;
LinkStack2 OVS;
int n,v,a,b;
char ch,op;
//
InitStack1(&OPSet);
Push1(OPSet,'+');
Push1(OPSet,'-');
Push1(OPSet,'*');
Push1(OPSet,'/');
Push1(OPSet,'^');
Push1(OPSet,'%');
ch=GetTop1(OPSet);
printf("haha");
putchar(ch);
InitStack1(&OPTR);
InitStack2(&OVS);
Push1(OPTR,'#');
printf("Input(end with #): ");
while(true)
{
scanf("%d",&n);
Push2(OVS,n);
ch=getchar();
switch(Compare(ch,GetTop1(OPTR)))
{
case '>':
Push1(OPTR,ch);
break;
case '=':
case '<':
Pop1(OPTR,&op);
Pop2(OVS,&b);
Pop2(OVS,&a);
v=Execute(a,op,b);
Push2(OVS,v);
}
if(ch=='#')
{
break;
}
}
/*
while(ch!='#' || GetTop(OPTR)!='#')
{
if(!In(ch,OPSet))
{
n=GetNumber(ch);
push(&OVS,n);
ch=getchar();
}
else
{
switch(Compare(ch,GetTop(OPTR)))
{
case '>':
Push(&OPTR,ch);
ch=getchar();
break;
case '=':
case '<':
Pop(&OPTR,&op);
Pop(&OVS,&b);
Pop(&OVS,&a);
v=Execute(a,op,b);
Push(&OVS,v);
break;
}
}
}
*/
return true;
}
int main()
{
if(ExpEvaluation())
{
printf("Yes\n");
}
return 0;
}
链式栈 C语言练习
原创
©著作权归作者所有:来自51CTO博客作者饮闲的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:定长顺序栈 C语言
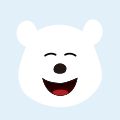
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
1.链式存储的线性表——C语言实现
本文是作者学习数据结构过程中在单链表基本运算代码实现时遇到问题并解决问题后的结果
单链表 基本运算 存储结构 代码实现 -
【数据结构】C语言实现顺序栈
【数据结构】第三章——栈、队列和数组详细介绍通过C语言实现顺序栈
数据结构 C语言 顺序栈 -
【数据结构】C语言实现共享栈
【数据结构】第三章——栈、队列与数组详细介绍通过C语言实现共享栈
数据结构 C语言 栈 共享栈 -
(C语言)栈的链式实现(数据结构九)
1.数据类型定义在代码中为了清楚的表示一些错误和函数运行状态,我们预先定义一些变量来表示这些状态。在head.h头文件
c 数据结构 栈 单链表 #define -
链式栈模板
//stack.h#include#include#define TRUE 1#define FALSE 0#define OK 1#define ERROR 0#define OVERFLOW -1#define UNDERFLOW ...
#define #include 数据 前端 数据 数据库 编程语言 -
谈谈你对JavaScript语言的看法
JavaScript语言的前身叫作Livescript。自从Sun公司推出著名的Java语言之后,Netscape公司引进了Sun公司有关Java的程序概念,将自己原有的Livescript 重新进行设计,并改名为JavaScript。 JavaScript是一种基于对象和事件驱动并具有安全性能的
谈谈你对JavaScript语言的看法 java javascript 浏览器 语言