import java.util.ArrayList;
import java.util.List;
// https://www.nowcoder.com/questionTerminal/a9fec6c46a684ad5a3abd4e365a9d362
public class Solution {
private static int[] toArray(List<TreeNode> nodes) {
if (nodes == null || nodes.size() == 0) {
return new int[0];
}
int[] result = new int[nodes.size()];
for (int i = 0; i < result.length; ++i) {
result[i] = nodes.get(i).val;
}
return result;
}
/**
* @param root TreeNode类 the root of binary tree
* @return int整型二维数组
*/
public static int[][] threeOrders(TreeNode root) {
// write code here
int[][] result = new int[3][];
List<TreeNode> nodes = PreOrder.solve(root);
result[0] = toArray(nodes);
nodes = MidOrder.solve(root);
result[1] = toArray(nodes);
nodes = PostOrder.solve(root);
result[2] = toArray(nodes);
return result;
}
}
class TreeNode {
int val = 0;
TreeNode left = null;
TreeNode right = null;
}
class PreOrder {
/**
* 两种情况
* 1. 没有左子树
* 2. 第一次来到这个节点的时候(mostRight指向null)
*
* @param root
* @return
*/
public static List<TreeNode> solve(TreeNode root) {
if (root == null) {
return new ArrayList<>(0);
}
List<TreeNode> result = new ArrayList<>();
TreeNode cur = root, mostRight;
while (cur != null) {
mostRight = cur.left;
if (mostRight != null) {
while (mostRight.right != null && mostRight.right != cur) {
mostRight = mostRight.right;
}
if (mostRight.right == null) {
result.add(cur);
mostRight.right = cur;
cur = cur.left;
continue;
} else {
mostRight.right = null;
}
} else {
result.add(cur);
}
cur = cur.right;
}
return result;
}
}
class MidOrder {
/**
* 两种情况
* 1. 没有左子树
* 2. 第二次来到这个节点的时候(mostRight指向cur)
*
* @param root
* @return
*/
public static List<TreeNode> solve(TreeNode root) {
if (root == null) {
return new ArrayList<>(0);
}
List<TreeNode> result = new ArrayList<>();
TreeNode cur = root, mostRight;
while (cur != null) {
mostRight = cur.left;
if (mostRight != null) {
while (mostRight.right != null && mostRight.right != cur) {
mostRight = mostRight.right;
}
if (mostRight.right == null) {
mostRight.right = cur;
cur = cur.left;
continue;
} else {
mostRight.right = null;
}
}
result.add(cur);
cur = cur.right;
}
return result;
}
}
class PostOrder {
private static TreeNode reverse(TreeNode head) {
if (head == null) {
return head;
}
TreeNode pre = null, cur = head, next;
while (cur != null) {
next = cur.right;
cur.right = pre;
pre = cur;
cur = next;
}
return pre;
}
/**
* 能回到两次,且第二次回到的时候,取左树右边界
*
* @param root
* @return
*/
public static List<TreeNode> solve(TreeNode root) {
if (root == null) {
return new ArrayList<>(0);
}
List<TreeNode> result = new ArrayList<>();
TreeNode cur = root, mostRight;
while (cur != null) {
mostRight = cur.left;
if (mostRight != null) {
while (mostRight.right != null && mostRight.right != cur) {
mostRight = mostRight.right;
}
if (mostRight.right == null) {
mostRight.right = cur;
cur = cur.left;
continue;
} else {
mostRight.right = null;
result.addAll(getRightEdge(cur.left));
}
}
cur = cur.right;
}
result.addAll(getRightEdge(root));
return result;
}
private static List<TreeNode> getRightEdge(TreeNode head) {
if (head == null) {
return new ArrayList<>(0);
}
List<TreeNode> result = new ArrayList<>();
TreeNode tail = reverse(head);
head = tail;
while (head != null) {
result.add(head);
head = head.right;
}
reverse(tail);
return result;
}
}
Morris遍历
转载
心之所向,素履以往 生如逆旅,一苇以航
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
下一篇:奇数下标是奇数,偶数下标是偶数
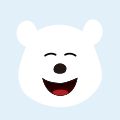
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
python优雅遍历字典删除元素
Python基础知识
删除元素 Python 简洁性 -
数据结构与算法-morris遍历
morris遍历的优点在于节省空间,因为不需要递归。
数据结构 System 逆序 先序遍历 -
Morris
Morris
Morris -
常考数据结构与算法-morris遍历
下图右边就是左边的树的morris序
子树 逆序 后序遍历 后序 中序遍历 -
488,二叉树的Morris中序和前序遍历
二叉树的Morris遍历方式
二叉树 编程开发 -
python中done的含义
1、内置函数 lambda用法一:类函数格式如下,[]表示可选 [生成器 =] lambda 参数x : 函数体 [实参] f = (lambda x: x=i) print(f(1)) # 输出1,这时候类似函数调用用法二:迭代print map(lambda x: x.startswith('s'), cities['City name']) # 实参部分是一个列表 [False,
python中done的含义 Lambda TensorFlow panda dot和matmul等乘法