#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* next;
}Node;
Node* createListHead();
Node* createListRear();
void printNodeList(Node* node);
//优化
Node* bestCreateList();
void bestInsertHead(Node* head, int data);
void bestInsertRear(Node* head, int data);
void destroyLinkList(Node* head);
int calcListLength(Node* head);
//find && del
Node* findList(Node* head, int key);//key是data(值)
void delListToNode(Node* head, Node* find);
//排序
void bubbleSort(Node* head);
void reverseList(Node* head);//反转
/*int main() {
//Node* node = createListHead();
//Node* node = createListRear();
//优化后
// 1.创建一个空链表
Node* head = bestCreateList();
// 2.往空链表中插入数据
bestInsertRear(head, 1);
bestInsertRear(head, 3);
bestInsertRear(head, 5);
printNodeList(head);
Node* node = findList(head, 3);
int countST = calcListLength(head);
printf("count=%d\n", countST);
delListToNode(head, node);
int countEND = calcListLength(head);
printf("count=%d\n", countEND);
printNodeList(head);
//printf("Node=%p,count=%d,next=%p\n", node,node->data,node->next);//Node=00B85A40,count=5,next=00000000
return 0;
}*/
int main() {
//优化后
// 1.创建一个空链表
Node* head = bestCreateList();
// 2.往空链表中插入数据
bestInsertRear(head, 2);
bestInsertRear(head, 10);
bestInsertRear(head, 6);
bestInsertRear(head, 88);
bestInsertRear(head, 23);
bestInsertRear(head, 93);
printNodeList(head);
printf("冒泡\n");
bubbleSort(head);
printNodeList(head);
printf("反转\n");
reverseList(head);
printNodeList(head);
return 0;
}
//尾插法
Node* createListRear() {
Node* head = (Node*)malloc(sizeof(Node));
if (head == NULL) {
return NULL;
}
head->next = NULL;
Node* r;
r = head;
int num = -1;
printf("请输入数据\n");
scanf_s("%i", &num);
while (num != -1) {
Node* p = (Node*)malloc(sizeof(Node));
p->data = num;
r->next = p;
p->next = NULL;
r = p;
scanf_s("%i", &num);
}
return head;
}
//头插法
Node* createListHead() {
//建立头结点
Node* head = (Node*)malloc(sizeof(Node));
if (head == NULL) {
return NULL;
}
head->next = NULL;
int num = -1;
printf("请输入数据\n");
scanf_s("%i", &num);
while (num != -1) {
Node* p = (Node*)malloc(sizeof(Node));
p->data = num;
p->next = head->next;
head->next = p;
scanf_s("%i", &num);
}
return head;
}
//遍历单向链表
void printNodeList(Node* node) {
Node* head = node->next;
while (head != NULL) {
int x = head->data;
printf("currentData = %i\n", x);
head = head->next;
}
}
//优化 单向链表的建立
Node* bestCreateList() {
Node* head = (Node*)malloc(sizeof(Node));
if (head == NULL) {
return NULL;
}
head->next = NULL;
return head;//返回创建好的节点
}
// 优化,单向链表 头插法
void bestInsertHead(Node* head,int data) {
// 1.创建一个新的节点
Node* cur = (Node*)malloc(sizeof(Node));
if (!cur) {
printf("xx");
return NULL;
}
else {
cur->data = data;
// 2.让新节点的下一个节点指向头节点的下一个节点
cur->next = head->next;
// 3.让头节点的下一个节点指向新节点
head->next = cur;
}
}
//优化 单向链表 尾插法
void bestInsertRear(Node* head, int data) {
// 记录到最后一个节点,尾插
Node* pre = head;
while (pre != NULL && pre->next != NULL) {
pre = pre -> next;
}
//建立新节点
Node* cur = (Node*)malloc(sizeof(Node));
//为什么要判断申请的指针是否为空?
//指针就是指向内存的某个地址的一个变量.
//空指针的地址是 0x00000 所以他不指向任何一个地方
//一般判断指针是否为空可以判断指针是否有效,以防止程序崩溃
//void *malloc(long NumBytes):该函数分配了NumBytes个字节,并返回了指向这块内存的指针。
//如果分配失败,则返回一个空指针(NULL)。关于分配失败的原因,应该有多种,比如说空间不足就是一种。
//printf("%p\n", cur);
if (cur == NULL || pre ==NULL) {
printf("null\n");
//exit;
}
else
{
cur->data = data;
pre->next = cur;
cur->next = NULL;
pre = cur;
}
}
// 销毁链表 释放内存
void destroyLinkList(Node* head) {
Node* cur = NULL;
while (head != NULL) {
cur = head->next;
free(head);
head = cur;
}
}
int calcListLength(Node* head)
{
int count = 0;
head = head->next;
while (head)
{
count++;
head = head->next;
}
return count;
}
//查找某个链表上某个节点
Node* findList(Node* head, int key)
{
head = head->next;
while (head) {
if (head->data == key) {
break;
}
else
{
head = head->next;
}
}
return head;
}
void delListToNode(Node* head, Node* find)
{
while (head->next != find) {
head = head->next;
}
head->next = find->next;
free(find);
}
//冒泡排序
void bubbleSort(Node* head)
{
int len = calcListLength(head);
printf("length:%d",len);
//定义前后结点
Node* p = NULL;
for (int i = 0; i < len - 1; i++) {
p = head->next;
for (int j = 0; j < len - 1 - i; j++) {
if (p == NULL) {
exit;
}else {
//printf("p1=%i, p2=%i\n", p->data, p->next->data);
if ((p->data) > (p->next->data)) {
int temp = p->data;
p->data = p->next->data;
p->next->data = temp;
}
p = p->next;
}
}
}
}
/**
* @brief reverseList 反转链表
* @param head 链表头指针 方法2:新建链表,头节点插入法
*/
void reverseList(Node* head) {
// 1.将链表一分为二
Node* pre, * cur;
pre = head->next;
head->next = NULL;
// 2.重新插入节点
while (pre) {
cur = pre->next;
pre->next = head->next;
head->next = pre;
pre = cur;
}
}
单向链表
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
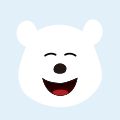
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Oracle11g之OGG单instance单向复制
ogg
oracle 初始化 ide -
【数据结构】链式家族的成员——循环链表与静态链表
【数据结构】第二章——线性表(8)详细介绍了循环链表与静态链表的相关内容……
数据结构 C语言 循环链表 静态链表