// SequenceList.h: interface for the CSequenceList class. // ////////////////////////////////////////////////////////////////////// #if !defined(AFX_SEQUENCELIST_H__ECAED4FD_189E_4994_9843_BC7E9134CAF8__INCLUDED_) #define AFX_SEQUENCELIST_H__ECAED4FD_189E_4994_9843_BC7E9134CAF8__INCLUDED_ #if _MSC_VER > 1000 #pragma once #endif // _MSC_VER > 1000 #define MAXSIZE 100 #define LIST_INIT_SIZE 100 #define LIST_INCREMENT 100 typedef int DataType; typedef struct { DataType *data; int iLength; int iAllocatedSpace; }SqList; class CSequenceList { public: SqList sqList; CSequenceList(); virtual ~CSequenceList(); void operator = (const CSequenceList listSeq) { sqList.data = listSeq.sqList.data; sqList.iLength = listSeq.sqList.iLength; sqList.iAllocatedSpace = listSeq.sqList.iAllocatedSpace; } BOOL InitList(); BOOL Insert(int index, DataType elem); BOOL Delete(int index); DataType GetAt(int index); BOOL DestroyList(); BOOL IsEmpty(); int GetLength(); int Find(int from, DataType& elem); void Unique(); CSequenceList MergeList(CSequenceList& listA); void Reverse(); }; #endif // !defined(AFX_SEQUENCELIST_H__ECAED4FD_189E_4994_9843_BC7E9134CAF8__INCLUDED_) // SequenceList.cpp: implementation of the CSequenceList class. // ////////////////////////////////////////////////////////////////////// #include "stdafx.h" #include "DataStruct.h" #include "SequenceList.h" #ifdef _DEBUG #undef THIS_FILE static char THIS_FILE[]=__FILE__; #define new DEBUG_NEW #endif ////////////////////////////////////////////////////////////////////// // Construction/Destruction ////////////////////////////////////////////////////////////////////// CSequenceList::CSequenceList() { } CSequenceList::~CSequenceList() { } /************************************************************************ 函数名: InitList 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 初始化顺序表,为为顺序表动态分配空间 形参数: 返回值: 成功:true 失败: false ************************************************************************/ BOOL CSequenceList::InitList() { sqList.data = (DataType*)malloc(LIST_INIT_SIZE * sizeof(DataType)); //sqList.data = new DataType[LIST_INIT_SIZE]; if (sqList.data == NULL) { return false; } sqList.iLength = 0; sqList.iAllocatedSpace = LIST_INIT_SIZE; return true; } /************************************************************************ 函数名: Insert 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 往顺序表中index之前插入插入元素 形参数: index 从0开始的索引 elem 要插入的元素 返回值: 成功:true 失败: false ************************************************************************/ BOOL CSequenceList::Insert(int index, DataType elem) { int i; DataType *newBase; if (index < 0 || index > sqList.iLength) { return false; } if (sqList.iLength >= sqList.iAllocatedSpace) { newBase = (DataType*)realloc(sqList.data, (sqList.iAllocatedSpace + LIST_INCREMENT) * sizeof(DataType)); if (!newBase) { return false; } sqList.data = newBase; sqList.iAllocatedSpace += LIST_INCREMENT; } for(i = sqList.iLength; i > index; i--) { sqList.data[i] = sqList.data[i - 1]; } sqList.data[index] = elem; sqList.iLength++; return true; } /************************************************************************ 函数名: Delete 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 删除顺序表中指定位置的元素 形参数: index 从0开始的索引 返回值: 成功:true 失败: false ************************************************************************/ BOOL CSequenceList::Delete(int index) { int i; if (index < 0 || index > sqList.iLength - 1 || sqList.iLength == 0) { return false; } for(i = index; i < sqList.iLength - 1; i++) { sqList.data[i] = sqList.data[i + 1]; } sqList.data[sqList.iLength - 1] = '\0'; sqList.iLength--; return true; } /************************************************************************ 函数名: GetAt 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 返回顺序表中指定位置的元素 形参数: index 从0开始的索引 返回值: ************************************************************************/ DataType CSequenceList::GetAt(int index) { if (index < 0 || index > sqList.iLength - 1) { return false; } return sqList.data[index]; } /************************************************************************ 函数名: DestroyList 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 摧毁顺序表 形参数: 返回值: ************************************************************************/ BOOL CSequenceList::DestroyList() { if (sqList.data) { free(sqList.data); } sqList.iLength = 0; sqList.iAllocatedSpace = 0; return true; } /************************************************************************ 函数名: IsEmpty 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 判断顺序表是否为空 形参数: 返回值: ************************************************************************/ BOOL CSequenceList::IsEmpty() { if (sqList.iLength == 0) { return false; } return true; } /************************************************************************ 函数名: GetLength 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 返回顺序表的实际长度 形参数: 返回值: ************************************************************************/ int CSequenceList::GetLength() { return sqList.iLength; } /************************************************************************ 函数名: Find 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 在顺序表中从from开始查找元素elem,找到则返回从0开始的元素索引 形参数: from 开始查找位置 elem 要查找的元素 返回值: 没有找到则返回-1,找到则返回从0开始的元素索引 ************************************************************************/ int CSequenceList::Find(int from, DataType& elem) { int i; if (from < 0 || from > sqList.iLength - 1) { return -1; } for (i = from; i < sqList.iLength; i++) { if (sqList.data[i] == elem) { return i; } } return -1; } /************************************************************************ 函数名: Unique 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 将顺序表中的重复元素删除 形参数: 返回值: ************************************************************************/ void CSequenceList::Unique() { int i, index; for(i = 0; i < sqList.iLength - 1; i++) { index = i + 1; while ((index = Find(index, sqList.data[i])) >= 0) { Delete(index); } } } /************************************************************************ 函数名: MergeList 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 将两个有序表合并 形参数: 返回值: ************************************************************************/ CSequenceList CSequenceList::MergeList(CSequenceList& listA) { int i, j, k; CSequenceList listSeq; listSeq.InitList(); i = j = k = 0; while(i < sqList.iLength && j < listA.sqList.iLength) { if (sqList.data[i] < listA.sqList.data[j]) { listSeq.Insert(listSeq.sqList.iLength, sqList.data[i++]); } else { listSeq.Insert(listSeq.sqList.iLength, listA.sqList.data[j++]); } } while(i < sqList.iLength) { listSeq.Insert(listSeq.sqList.iLength, sqList.data[i++]); } while(j < listA.sqList.iLength) { listSeq.Insert(listSeq.sqList.iLength, listA.sqList.data[j++]); } return listSeq; } /************************************************************************ 函数名: Reverse 作 者: 谭友亮(Charles Tan) 日 期: 2013-4-12 作 用: 逆转顺序表 形参数: 返回值: ************************************************************************/ void CSequenceList::Reverse() { int i; DataType temp; for(i = 0; i < sqList.iLength / 2 + sqList.iLength % 2; i++) { temp = sqList.data[i]; sqList.data[i] = sqList.data[sqList.iLength - i - 1]; sqList.data[sqList.iLength - i - 1] = temp; } }
顺序表相关操作
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
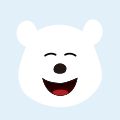
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
队列及其相关操作
队列的实现,以及简单的增删查改操作
数据 链表 队列 -
双链表及其相关操作
讲解了链表的分类,常用链表(双链表)的增删查改,接口的优化。
链表 双向链表 双链表的增删查改 接口优化 链表的分类 -
Oracle SQL执行计划操作(1)——表相关操作
本文详细说明了Oracle SQL语句执行计划中表相关操作,同时,结合实例对其进行了深入讲解。
plan SQL table operator 操作 -
python列表相关
LIST 列表 1. 定义:是一个有序且可变的容器,在里面可以存放多个不同类型的元素。
老男孩学习笔记 python for循环 整型 表数据