#include <iostream> using namespace std; typedef struct Student{ char Num[12]; // 学号 char Name[10]; //姓名 int age; //年龄 float score; //成绩 Student *next; }Stu; void CreateList(Stu* &L,int n){ //(1)将随机输入的n个学生(信息),构成一个不带头结点的链表L int i; Stu *temp,*tail; tail=L; cout<<"Please input "<<n<<" students' information(Num,Name,age and score:\n"; for(i=1;i<=n;i++){ temp=new Stu; temp->next=NULL; cin>>temp->Num>>temp->Name>>temp->age>>temp->score; if(tail==NULL) L=temp; else tail->next=temp; tail=temp; } } int Length(Stu *L){ Stu* temp=L; int count=0; while(temp!=NULL){ count++; temp=temp->next; } return count; } void Display(Stu* L){ //(2)用递归方法遍历链表,即输出各结点的信息 if(L==NULL) return; else{ cout<<L->Num<<","<<L->Name<<","<<L->age<<","<<L->score<<endl; Display(L->next); } } float MaxScore(Stu* L){ //(2)计算链表中结点的最大成绩 Stu* temp=L->next; float maxscore=L->score; while(temp!=NULL){ if(temp->score>maxscore) maxscore=temp->score; temp=temp->next; } return maxscore; } float Average(Stu* L){//(2)计算链表中所有结点的平均成绩 Stu* temp=L; float sum=0;int count=0; while(temp!=NULL){ sum+=temp->score; count++; temp=temp->next; } return sum/count; } void main(void){ Stu* List=NULL; CreateList(List,3); cout<<endl; cout<<"the length of the list is "<<Length(List)<<endl; Display(List); cout<<endl; cout<<"the highest score is "<<MaxScore(List)<<endl; cout<<"the average score is "<<Average(List)<<endl; }
链表
转载
如何用C++实现链表?
博客签名:敬畏生命,珍惜时间,热爱生活。
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:gifcam
下一篇:构造函数、重载、析构函数
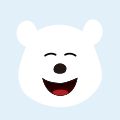
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】链式家族的成员——循环链表与静态链表
【数据结构】第二章——线性表(8)详细介绍了循环链表与静态链表的相关内容……
数据结构 C语言 循环链表 静态链表 -
双链表及其相关操作
讲解了链表的分类,常用链表(双链表)的增删查改,接口的优化。
链表 双向链表 双链表的增删查改 接口优化 链表的分类 -
链表-双向链表
双向链表实现
链表 删除元素 i++ -
排序链表【链表】
时间复杂度:空间复杂度:
链表 python 数据结构 空间复杂度 时间复杂度 -
链表-循环链表
链表-循环链表
链表 i++ 删除元素 -
链表初探-遍历链表
一起养成写作习惯!
前端 JavaScript 链表 数据结构 -
【链表】链表快速排序
链表快速排序
链表 数据结构 结点