import pymysql
class MysqlHelper:
def __init__(self, config):
self.host = config["host"]
self.port = config["port"]
self.user = config["user"]
self.password = config["password"]
self.db = config["db"]
self.charset = config["charset"]
self.con = None
self.cursor = None
def create_con(self):
"""
创建连接
"""
try:
self.con = pymysql.connect(host=self.host, port=self.port, user=self.user, password=self.password,
database=self.db, charset='utf8')
self.cursor = self.con.cursor()
return True
except Exception as e:
print(e)
return False
def close_con(self):
"""
关闭链接
"""
if self.cursor:
self.cursor.close()
if self.con:
self.con.close()
# sql执行
def execute_sql(self, sql):
"""
执行插入/更新/删除语句
"""
try:
self.create_con()
print(sql)
self.cursor.execute(sql)
self.con.commit()
except Exception as e:
print(e)
finally:
self.close_con()
def select(self, sql, *args):
"""
执行查询语句
"""
try:
self.create_con()
print(sql)
self.cursor.execute(sql, args)
res = self.cursor.fetchall()
return res
except Exception as e:
print(e)
return False
finally:
self.close_con()
if __name__ == '__main__':
config = {
"host": 'localhost',
"port": 3306,
"user": 'root',
"password": '123',
"db": 'test',
"charset": 'utf8'
}
db = MysqlHelper(config)
#添加
# db.execute_sql("insert into user (username, password) values ('username4','password4')")
#修改
db.execute_sql("update channellist set is_active=1 where id=2")
#查询
# res = db.select("SELECT * FROM channellist;")
# print(res)
python-mysql操作 封装工具类
转载
从小白到大神的蜕变~~
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
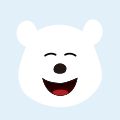
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Node Mysql操作封装
由于最近要写个签到系统,
node mysql 连接池 数据库配置 -
python:封装CRUD操作
# 封装数据库操作def SELECT(db, cursor, sql): try: # 执行SQL语句 db.ping(reconnect=True)
python list sql sql语句 回滚