Scanner in = new Scanner(System.in);
while (in.hasNextLine()) {
String name1 = in.nextLine();
String name2 = in.nextLine();
int sum = calcSimilarity(name1, name2);
System.out.println(sum);
}
public static String getMaxLengthCommonString(String s1, String s2) {
if (s1 == null || s2 == null) {
return null;
}
a = s1.length();//s1长度做行
b = s2.length();//s2长度做列
if(a== 0 || b == 0) {
return "";
}
//设置匹配矩阵
boolean [][] array = new boolean[a][b];
for (int i = 0; i < a; i++) {
char c1 = s1.charAt(i);
for (int j = 0; j < b; j++) {
char c2 = s2.charAt(j);
if (c1 == c2) {
array[i][j] = true;
} else {
array[i][j] = false;
}
}
}
//求所有公因子字符串,保存信息为相对第二个字符串的起始位置和长度
List<ChildString> childStrings = new ArrayList<ChildString>();
for (int i = 0; i < a; i++) {
getMaxSort(i, 0, array, childStrings);
}
for (int i = 1; i < b; i++) {
getMaxSort(0, i, array, childStrings);
}
//排序
sort(childStrings);
if (childStrings.size() < 1) {
return "";
}
//返回最大公因子字符串
int max = childStrings.get(0).maxLength;
StringBuffer sb = new StringBuffer();
for (ChildString s: childStrings) {
if (max != s.maxLength) {
break;
}
sb.append(s2.substring(s.maxStart, s.maxStart + s.maxLength));
}
return sb.toString();
}
//排序,倒叙
private static void sort(List<ChildString> list) {
Collections.sort(list, new Comparator<ChildString>(){
public int compare(ChildString o1, ChildString o2) {
return o2.maxLength - o1.maxLength;
}
});
}
//求一条斜线上的公因子字符串
private static void getMaxSort(int i, int j, boolean[][] array, List<ChildString> sortBean) {
int length = 0;
int start = j;
for (; i < a && j < b; i++,j++) {
if (array[i][j]) {
length++;
} else {
sortBean.add(new ChildString(length, start));
length = 0;
start = j + 1;
}
if (i == a-1 || j == b-1) {
sortBean.add(new ChildString(length, start));
}
}
}
//公因子类
static class ChildString {
int maxLength;
int maxStart;
ChildString(int maxLength, int maxStart){
this.maxLength = maxLength;
this.maxStart = maxStart;
}
}
【Java】最长公共子串
原创
©著作权归作者所有:来自51CTO博客作者瑞新的博客的原创作品,请联系作者获取转载授权,否则将追究法律责任
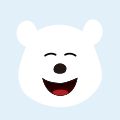
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java-长字符串加密
加密:为你的长字符串提供最高级别的保护!!!
加密算法 JAVA -
python解决最长公共子串和最长公共子序列
什么是最长公共子串??????最长公共子串就是指两个字符串中有部分子串相同且是最长的
python 列表 字符串 算法 List -
【动态规划】最长公共子序列与最长公共子串
3. 参考资料[1] cs2035, Longest Common Subsequence.[2] 一线码农, 经典算法题每日演练—
c语言 Common 经典算法 Dynamic -
【qduoj】最长公共子串
题干:描述 编写一个程序,求两个字符串的最长公共子串。输出两个字符串的长度,输出他们的最长公共子串及子串长度。如果有多个最长公共子串
#include 字符串 最长公共子串