import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
/**
* 模拟字符流缓冲区 readline()
* 装饰模式
* @author WangShuang
*
*/
public class Demo {
public static void main(String[] args) {
MyBufferedReader my = null;
try {
FileReader fr = new FileReader("c:\\字符流缓冲区.txt");
my = new MyBufferedReader(fr);
String buff = null;
while((buff = my.myReadLine())!=null){
System.out.println(buff);
}
} catch (Exception e) {
throw new RuntimeException("读取错误");
}finally {
try {
if(my!=null)
my.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
class MyBufferedReader extends Reader {
Reader in = null;
public MyBufferedReader(Reader in) {
this.in = in;
}
//增强的方法
public String myReadLine() throws IOException{
StringBuffer sb = new StringBuffer();
int ch = 0;
while((ch = in.read())!=-1){
if(ch == '\r')
continue;
if(ch== '\n')
return sb.toString();
else
sb.append((char)ch);
}
if(sb.length()!=0){
return sb.toString();
}
return null;
}
@Override
public int read(char[] cbuf, int off, int len) throws IOException {
return in.read(cbuf, off, len);
}
@Override
public void close() throws IOException {
in.close();
}
}
字符流缓冲区原理之装饰模式
原创
©著作权归作者所有:来自51CTO博客作者奔跑吧爽爽的原创作品,请联系作者获取转载授权,否则将追究法律责任
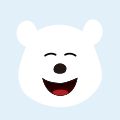
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章