# Untitled - By: Administrator - 周二 九月 22 2020
import sensor, image, time , pyb
from pyb import UART
from pyb import Timer
from pyb import LED
import json
thresholds = [(53, 100, -101, 127, -59, 127), # 红色
#(21, 75, 3, -38, 34, 68), # 绿色
(52, 100, -101, 127, -59, 127),
(39, 100, 15, 127, -112, 127)] # generic_blue_thresholds
threshold_index = 2 # 0 for red, 1 for gre9en, 2 for blue
#####***********************************************************************
'''
通信定义的帧头,帧尾等,,,
'''
FunNum=100
TaskFlag=0
StartChar=0xab #帧头
EndChar=0xba #帧尾
EndCharRec=0x00
RecOK=0xd1 #成功的代码
flagFun1=0
flag1=0
nscan=1 #二维码扫描
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
sensor.set_auto_gain(False) # must be turned off for color tracking
sensor.set_auto_whitebal(False) # must be turned off for color tracking
uart=UART(1,9600) #PA9 txd PA10--rxd
uart.init(9600, bits=8, parity=None, stop=1) # init with given parameters timeout_char=1000
clock = time.clock()
outstr1='999+999' #123+321
#******************************************************************
def delayt(delayi):
while delayi>0:
delayi=delayi-1
##########################################################
def tick(timer): # we will receive the timer object when being called
global data
global FunNum
global TaskFlag
global nscan
if TaskFlag==1 : #如果是第一个功能,发送二维码数据
print('task01:',TaskFlag)
if TaskFlag==2 : #如果是第2个功能,发送颜色数据的位置
print('task02:',TaskFlag)
if TaskFlag==0:
print("taskflag0")
############################################################
#10HZ===0.1S=100MS
tim = Timer(4, freq=10) # create a timer object using timer 4 - trigger at 1Hz/
tim.callback(tick) # set the callback to our tick function
#**************************************************************
#******************************************************
'''
openmv 接收单片机发过来的功能码,选择执行的程序
如果功能码是0x11---- flagFun1=1 TaskFlag=1执行扫描二维码的程序
0x12---- flagFun1=2 TaskFlag=2执行扫描色块的程序
、、、、
'''
#*************************************************
def Uart_Receive(): #UART接收
global threshold_index
global TaskFlag
global EndChar
global EndCharRec
global StartChar
global flagFun1
global flag1
if uart.any():
temp_data0 = uart.readchar()
if temp_data0==0xab: #帧头相等0xab-171
FunNum = uart.readchar() #功能码0x11----17
EndCharRec=uart.readchar() #帧尾0xba----186
temp_data1=uart.readchar() #接收校验码
if EndCharRec==0xba: #帧尾相等
##计算校验码,看下和传来的是不是一样http://www.metools.info/code/c128.html
checksumtemp=(temp_data0+FunNum+EndCharRec)%256 #171+18+186=375-256=119---hex(77)
if checksumtemp==temp_data1: #如果校验码相等
time.sleep(1)
if FunNum==0x11 and flag1==0: #功能1:二维码扫描 第一扫描二维码,后面不带打开这个功能
#threshold_index=0
flagFun1=1
TaskFlag=1
flag1=1 #flag1=1,不再接受这个功能,让flag1=0条件不成立
elif FunNum==0x12:#功能2:颜色扫描
#threshold_index=1
TaskFlag=2
#***************************************************************
#88888888888888888888888888888888888888888888888888888888888888888888888888
while(True):
clock.tick()
img = sensor.snapshot()
Uart_Receive()
time.sleep(1000)
if flagFun1==1:#第一个功能:扫描二维码
#'''
#扫描50次,50次之后,有30次相同的结果,把那个结果当做有效结果,发送到51单片机中
#'''
##*************************************************************************
#连续发10秒,总有一次是成功的,如果单片机接收到0xab+0xdd+0xba+校验码,四个都相等,说明mv端接收到01号人物了。
#连续发10秒的数据,目的是担心单片机接收端出现问题,除非加上单片机的应答信号
for num in range(1,10):
print("Send OK code to 51")
uart.writechar(0xab) #//0xab
uart.writechar(0xd1)
uart.writechar(0xba)
#ab+dd+ba=578(shi)%256=66(shi)===0x42
uart.writechar((StartChar+RecOK+EndChar)%256)#
#time.sleep(1000)
flagFun1=0 #扫描二维码的任务结束,不在执行
#data0=bytearray([0xab,0x31,0xba,0x78])
#uart.write(data0)
time.sleep(1000)
#********************************************************************************
#发送成功的请求之后,对焦,找到二维码,直到找到二维码,没有找到二维码,
#主要是注意payload的类型。
#*******************************经过试验,基本经过一次,只要能检测,检测的结果基本都是对的
print("********Scan CODE*******")
while nscan<5:
delayt(100)
TaskFlag=2
clock.tick()
img = sensor.snapshot()
img.lens_corr(1.8) # strength of 1.8 is good for the 2.8mm lens.
for code in img.find_qrcodes():
img.draw_rectangle(code.rect(), color = (255, 0, 0))
#print(code)
time.sleep(1)
#print("OOOO*******OOOOOO*****OOOO")
outstr1=str(code.payload())
if outstr1!='999+999': #如果不等于初值,说明读取到了二维码,连续扫描5次,得到结果
nscan+=1
##得到二维码,拆接之后,一个个发送出去,变成字符串,发送出去
#time.sleep(1000)
##print(outstr1[3])
data01=bytearray([0xab,0x31,0x12,0xba])
#171+
#注意,ord,是将括号的单字符,变为对应的整数,chr将0-255变为字符
#可以用writechar(outstr1[0])
print("*********************")
print(ord(outstr1[0])) #51 3
print(ord(outstr1[1])) #49 1
print(ord(outstr1[2])) #50 2
print(ord(outstr1[3])) #43 +
print(chr(ord(outstr1[4]))) # 3
print(chr(ord(outstr1[5]))) # 2
print(chr(ord(outstr1[6]))) # 1
#171+49+51+49+50+43+49+50+51+186 %256=237,所以打印237
checksum02=(0xab+0x31+ord(outstr1[0])+ord(outstr1[1])+ord(outstr1[2])+ord(outstr1[3])+ord(outstr1[4])+ord(outstr1[5])+ord(outstr1[6])+0xba)%256
print(checksum02)
print("*********************")
#data01=bytearray([0xab,0x31,ord(outstr1[0]),ord(outstr1[1]),ord(outstr1[2]),ord(outstr1[3]),ord(outstr1[4]),ord(outstr1[5]),ord(outstr1[6]),0xba,checksum02])
# uart.write(data01)
uart.writechar(0xab)
uart.writechar(0x31)
uart.write(outstr1[0])
uart.write(outstr1[1])
uart.write(outstr1[2])
uart.write(outstr1[3])
uart.write(outstr1[4])
uart.write(outstr1[5])
uart.write(outstr1[6])
uart.writechar(0xba)
uart.writechar(checksum02)
time.sleep(1000)
TaskFlag=1
#print(clock.fps())
ope串st8,o端程序
原创
©著作权归作者所有:来自51CTO博客作者XGLIYOUQUAN的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:图像模块的学习
下一篇:yanzheng02
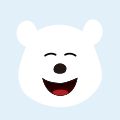
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
8种超简单的Golang生成随机字符串方式
go语言中,有没有什么最快最简单的方法,用来生成只包含英文字母的随机字符串。
go语言 Bytes Golang -
[USACO 2006 Ope B]Cows on a Leash
// Problem: [USACO 2006 Ope B]Cows on a Leash// Contest: NowCoder// URL: h
深度优先 贪心算法 #define git 区间覆盖 -
HDFS客户端程序开发-HDFS的I/O流操作
HDFS客户端程序开发-HDFS的I/O流操作上面我们学的API操作HDFS系统都是框架封装好的。如
hadoop 大数据 hdfs 输出流 -
st官方的ymodem程序
Symfony核心类Symfony的MVC方式使用了一些你以后会经常碰到的类l sfController是控制器,他解码请求并专递至动作(action)l sfRequest存储了所有的请求元素
st官方的ymodem程序 phpdoc 文档 数据库 sqlite