#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <queue>
using namespace std;
//ac_automaton algorithm
const int MAXNODE = 550000;
const int SIGMA_SIZE = 26;
int fail[MAXNODE]; //失配数组
int last[MAXNODE]; //后缀链接
int ans;
//构造trie
int ch[MAXNODE][SIGMA_SIZE]; //将单词存储在ch中
int val[MAXNODE]; //每个单词出现的个数
int sz; //节点编号
void init()
{
ans = 0;
sz = 1;
memset(ch[0], 0, sizeof(ch[0]));
}
void insert(char *s)
{
int u = 0;
int len = strlen(s);
for(int i = 0; i < len; ++i)
{
int c = s[i] - 'a';
if(!ch[u][c])
{
memset(ch[sz], 0, sizeof(ch[sz]));
val[sz] = 0;
ch[u][c] = sz++;
}
u = ch[u][c];
}
val[u]++;
}
//计算last后缀链接
void getFail()
{
queue<int> q;
fail[0] = 0;
for(int c = 0; c < SIGMA_SIZE; c++)
{
int u = ch[0][c];
if(u)
{
fail[u] = 0; q.push(u); last[u] = 0;
}
}
while(!q.empty())
{
int r = q.front(); q.pop();
for(int c = 0;c < 26;c++)
{
int u = ch[r][c];
if(!u){ ch[r][c] = ch[fail[r]][c]; continue;}
q.push(u);
int v = fail[r];
while(v && !ch[v][c]) v = fail[v];
fail[u] = ch[v][c];
last[u] = val[fail[u]] ? fail[u] : last[fail[u]];
}
//cout << last[r] <<endl;
}
}
void solve(int j)
{
//cout << j <<endl;
if(!j) return;
if(val[j])
{
ans += val[j];
val[j] = 0;
}
solve(last[j]);
}
void find(char* T)
{
int len = strlen(T),j = 0;
getFail();
for(int i = 0; i < len; i++)
{
j = ch[j][T[i] - 'a'];
//cout << val[j] <<endl;
if(val[j]) solve(j);
else if(last[j]) solve(last[j]);
}
}
AC自动机模板(多模式匹配)
原创
©著作权归作者所有:来自51CTO博客作者qq5813099298a32的原创作品,请联系作者获取转载授权,否则将追究法律责任
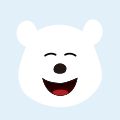
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C++ 模板匹配matchTemplate
C++ 模板匹配matchTemplate
opencv 模版匹配 -
【数据结构】详细介绍串的简单模式匹配——朴素模式匹配算法
【数据结构】第四章——串详细介绍串的朴素模式匹配算法……
数据结构 模式匹配 C语言 字符串匹配 -
java OpenCV多模板匹配 opencv的模板匹配
在这一篇文章中,我们将会了解数字图像处理中重要的组成部分之一的模板匹配。一:什么是模板匹配?在OpenCV教程中这样解释模板匹配:模板匹配是一项在一幅图像中寻找与另一幅模板图像最匹配(相似)部分的技术.这里说的模板是我们已知的小图像,模板匹配就是在一副大图像中搜寻目标。模板就是我们已知的在图中要找的目标,且该目标同模板有相同的尺寸、方向和图像,通过一定的算法可以在图中找到目标,确定其坐标位置。二:
java OpenCV多模板匹配 opencv 模板匹配 opencv 模板匹配带角度 opencv模板匹配 opencv模板匹配优化