lk/kernel/thread.c
thread_resume:
/**
* @brief Make a suspended thread executable.
*
* This function is typically called to start a thread which has just been
* created with thread_create()
*
* @param t Thread to resume
*
* @return NO_ERROR on success, ERR_NOT_SUSPENDED if thread was not suspended.
*/
status_t thread_resume(thread_t *t)
{
DEBUG_ASSERT(t->magic == THREAD_MAGIC);
DEBUG_ASSERT(t->state != THREAD_DEATH);
bool resched = false;
bool ints_disabled = arch_ints_disabled();
THREAD_LOCK(state);
if (t->state == THREAD_SUSPENDED) {
t->state = THREAD_READY;
insert_in_run_queue_head(t);
if (!ints_disabled) /* HACK, don't resced into bootstrap thread before idle thread is set up */
resched = true;
}
mp_reschedule(MP_CPU_ALL_BUT_LOCAL, 0);
THREAD_UNLOCK(state);
if (resched)
thread_yield();
return NO_ERROR;
}
lk thread_resume
原创sunlei0625 ©著作权
©著作权归作者所有:来自51CTO博客作者sunlei0625的原创作品,请联系作者获取转载授权,否则将追究法律责任
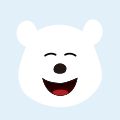
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java Thread Dump文件分析
Java Thread Dump文件分析
java 堆栈 Java